hw5
.pdf
keyboard_arrow_up
School
University Of Arizona *
*We aren’t endorsed by this school
Course
252
Subject
Computer Science
Date
Apr 3, 2024
Type
Pages
17
Uploaded by MagistrateQuetzal3896 on coursehero.com
CSc 252: Computer Organization
HW 5
due at 5pm, two days
before
the test
Turn in through GradeScope
Policy Reminders
You must turn in a single
PDF
to GradeScope. Other file formats will not be accepted. (Sorry, this is
necessary for the sanity of the TAs!)
You are allowed to work with other students on this homework, as we will not be grading it for
correctness. However,
each student must turn in their own copy of the homework.
Show your work for all problems.
While we won’t be grading for correctness, you will not receive
full credit unless you show your work.
After all, showing your work is required on the test - and homeworks are intended to help you practice
for the test!
Required Problems:
5.1(e-f), 5.2(d), 5.3(d), 5.4(f)
Allowable Instructions
When writing MIPS assembly, the only instructions that you are allowed to use (so far) are:
add, addi, sub, addu, addiu, subu
beq, bne, j, jal, jr
slt, slti
and, andi, or, ori, nor, nori, xor, xori
sll, srl, sra
lw, lh, lb, sw, sh, sb
la
syscall
mult, div, mfhi, mflo
While MIPS has many other useful instructions (and the assembler recognizes many pseudo-instructions),
do not use them! We want you to learn the
fundamentals
of how assembly language works - you can use
fancy tricks after this class is over.
Problem 5.1 - Dependencies
In each part below, list all of the depdencies between instructions.
I have numbered the instructions for
convenience.
5.1(a)
add
$s2, $s5, $s4
# instruction 1
add
$s4, $s2, $s5
# instruction 2
sw
$s5, 100($s2)
# instruction 3
add
$s3, $s4, $s2
# instruction 4
5.1(b)
add
$s2, $s5, $s4
# instruction 1
add
$s5, $s2, $s5
# instruction 2
sw
$s5, 100($s2)
# instruction 3
lw
$s5, 104($s2)
# instruction 4
NOTE:
Think carefully about what is read, and what is written, in lw/sw. Where are the dependencies?
5.1(c)
lw
$t0, 0($s0)
# instruction 1
sw
$t0, 0($s1)
# instruction 2
lw
$t1, 0($t0)
# instruction 3
beq
$t0, $t1, LABEL
# instruction 4
5.1(d)
add
$s2, $s0, $s1
# instruction 1
add
$s3, $s1, $s2
# instruction 2
add
$s4, $s2, $s3
# instruction 3
add
$s5, $s3, $s4
# instruction 4
5.1(e) - Turn in this one
la
$t0, foo
# instruction 1
lw
$t1, 0($t0)
# instruction 2
add
$t2, $s0, $s1
# instruction 3
sub
$t3, $t1, $t2
# instruction 4
5.1(f) - Turn in this one
add
$s2, $s0, $s1
# instruction 1
add
$s5, $s3, $s4
# instruction 2
sub
$s6, $s2, $s5
# instruction 3
addi $s7, $s6, 1
# instruction 4
Page 2
Problem 5.2 - Converting MIPS to C
In the following problems, I will give you a snippet of MIPS assembly, which you will convert to C. Assume
that all the MIPS code will use
tX
registers for temporary values (that is, values which are not given names
in C), and that any
sX
registers represent variables which have names in C.
If the code includes any
.data
section, then include exactly equivalent C declarations in your code. Also,
if the code uses any
sX
registers, give C declarations for matching variables. The names don’t matter, but
giving the proper types is important.
The possible types are:
int
- MIPS words. Use this when you don’t know anything else.
int*
- Pointers to MIPS words or arrays of words.
char*
- Pointers to bytes or strings.
To figure out types, you will have to use any number of clues - such as which variables are used in
la, lw,
sw
instructions, how arrays are indexed, or what syscalls are used.
Use comments to clearly show what
register is associated with each variable name.
Likewise, if the assembly calls a function, give a declaration (not a definition!) for the function, including
what you can figure out about the parameters and return type (if any).
Read the examples closely to see what I’m looking for.
5.2(a)
.global myFunc
myFunc:
addiu
$sp, $sp, -24
sw
$fp, 0($sp)
sw
$ra, 4($sp)
addiu
$fp, $sp, 20
add
$t0, $a0, $a1
add
$t1, $a2, $a3
sub
$v0, $t0, $t1
lw
$ra, 4($sp)
lw
$fp, 0($sp)
addiu
$sp, $sp, 24
jr
$ra
Page 3
5.2(b)
.data
str:
.asciiz
...something...
foo:
.word
0
.text
addi
$s0, $zero, 0
la
$s1, str
LOOP:
lb
$t0, 0($s1)
beq
$t0, $zero, LOOP_END
addi
$t1, $zero, 0x78
# 0x78 = ’x’
bne
$t0, $t1, FALSE
addi
$s0, $s0, 1
FALSE:
addi
$s1, $s1, 1
j
LOOP
LOOP_END:
la
$t0, foo
sw
$s0, 0($t0)
Page 4
5.2(c)
addi
$a0, $zero, 1
jal
doThatThing
addi
$t0, $v0, $zero
addiu
$sp, $sp, -4
sw
$t0, 0($sp)
addi
$a0, $zero, 2
jal
doThatThing
addi
$t1, $v0, $zero
addiu
$sp, $sp, -4
sw
$t1, 0($sp)
addi
$a0, $zero, 3
jal
doThatThing
addi
$t2, $v0, $zero
lw
$t1, 0($sp)
lw
$t0, 4($sp)
addiu
$sp, $sp, 8
addi
$v0, $zero, 1
add
$a0, $t0, $t1
add
$a0, $a0, $t2
syscall
addi
$v0, $zero, 11
addi
$a0, $zero, 0xa
syscall
Page 5
5.2(d) - Turn in this one
.global problemPartD
problemPartD:
addiu
$sp, $sp, -24
sw
$fp, 0($sp)
sw
$ra, 4($sp)
addiu
$fp, $sp, 20
addiu
$sp, $sp, -4
sw
$s0, 0($sp)
addi
$s0, $zero, 0
LOOP:
jal
getAValue
beq
$v0, $zero, LOOP_END
add
$s0, $s0, $v0
j
LOOP
LOOP_END:
add
$v0, $s0, $zero
lw
$s0, 0($sp)
addiu
$sp, $sp, 4
lw
$ra, 4($sp)
lw
$fp, 0($sp)
addiu
$sp, $sp, 24
jr
$ra
Page 6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
You should offer a way to enhance the file's organisation.
arrow_forward
Question No:
2012123nt505
2This is a subjective question, hence you have to write your answer in the Text-Field given below.
76610
A team of engineers is designing a bridge to span the Podunk River. As part of the design process,
the local flooding data must be analyzed. The following information on each storm that has been
recorded in the last 40 years is stored in a file: the location of the source of the data, the amount of
rainfall (in inches), and the duration of the storm (in hours), in that order. For example, the file
might look like this:
321 2.4 1.5
111 33 12.1
etc.
a. Create a data file.
b. Write the first part of the program: design a data structure to store the storm data from the file,
and also the intensity of each storm. The intensity is the rainfall amount divided by the duration.
c. Write a function to read the data from the file (use load), copy from the matrix into a vector of
structs, and then calculate the intensities.
(2+3+3)
arrow_forward
Apart from immutability, what other extended file attributes are there?
arrow_forward
Apart from being immutable, what other extended file attributes are
available to choose from?
arrow_forward
Assignment 2 Directions
Please choose Option 1 or Option 2. You do not need to do both.
Option 1:
Please write a short paper based on Module 2 Hardware Components. Anything in the module can be a topic.
Write a summary paper as follows:
• Prepare a Word document with a minimum of 3 pages (not including title and references pages) and a minimum of 3
solid references.
Be sure to use APA Guidelines for references.
• Make sure to save the file in the following format: FirstName_LastName_Paper.doc(x)
• Upload the completed file as an attachment to this assignment.
.
Option 2:
1. Create an algorithm to add two integer numbers.
2. Implement the program in a high level language like C or Java. It does not need to run for me, but the code should
be included in a text document called FirstnameLastnameHLA2.txt in your assignment submission.
3. Implement the program in MIPSzy Assembly language. Use the high level code as comments to the right of the
Assembly code as the textbook does.
4. If you…
arrow_forward
Other from being immutable, what other extended file attributes are available to choose from?
arrow_forward
It would be helpful if you could provide a suggestion to enhance the way a file is organized.
arrow_forward
Computer Science
The size of a file as reported by the ls -l listing is 10323433 bytes. However, the size of the same file when reported by the du command is 1000 blocks where a block is 512 bytes. Explain the discrepancy between these two sizes.
arrow_forward
What were the intentions behind the creation of the Bevco.xls file?
arrow_forward
You need to provide a means through which the file's
organization might be improved.
arrow_forward
CPE162_exer3_6 [Read-Only] [Compatibility Mode] - Word
Ace Jan C. Lape
File
Home
Insert
Design
Layout
References
Mailings
Review
View
Help
O Tell me what you want to do
& Share
X Cut
P Find -
Arial
- 11
A A Aa -
AaBbCc AAB6CCL AaBbC AaBbCc[ AaBbCcl AaBbCcD
akc Replace
A Select -
EE Copy
BI U
Paste
- abe x, x A- aly , A
1 Caption
Emphasis Heading 1 1 Normal
Strong
Subtitle
-
Format Painter
Clipboard
Font
Paragraph
Styles
Editing
Computer Engineering Department
Laboratory Activity Form
Course Number
ES 085
Course Title
Computer Programming 2
Topics Covered:
Array
Implement a program using the single-subscripted array and its
operations with emphasis on the creation of programmer-defined data
types and the use of separate files for the definition - .h, implementation -
.c and client code - .c.
Objectives:
Description
Airline Reservation System
A small airline has just purchased a computer for its new automated reservations system. The
president has asked you to program the new system. You…
arrow_forward
Which specific purpose does the Bevco.xls file serve?
arrow_forward
What use does the Bevco.xls file serve?
arrow_forward
What degree of dissimilarity exists between a file's logical and physical descriptions?
arrow_forward
CCS122_exer_pointer [Read-Only] [Compatibility Mode] - Word
Ace Jan C. Lape
File
Home
Insert
Design
Layout
References
Mailings
Review
View
Help
O Tell me what you want to do
& Share
X Cut
P Find -
Arial
- 11
- A A Aa -
AABBCCL AABBC AaBbCc[ AaBbCcl AaBbCcD AaBbC
akc Replace
A Select -
EE Copy
Paste
BI U - abe x, x A - ay - A
Emphasis
Heading 1
I Normal
Strong
Subtitle
Title
Format Painter
Clipboard
Font
Paragraph
Styles
Editing
1. Create a project named "batch_10_01" inside "batch_10_01" folder.
2. Create the header file "pointer h" and define the methods of the following
functions using pointers as function parameters:
void areaCircle(int *radius, int *acirc)
{
/*compute the area of a circle and store the result to
the variable pointed by *acirc */
}
void circumCircle(int *radius.int *circumCircle)
{
*compute the circumference of a circle andd store the result
to the variable pointed by *circumCircle */
}
void diaCirc(int *radius, int diamCirc)
{/*compute the diameter of a circle andd…
arrow_forward
You should suggest a solution to improve the file's organisation.
arrow_forward
Write about CSV data storage format with an example.
Download a .CSV file from the internet and answer the below questions:
What is the file name?
Describe the downloaded CSV file.
What are the columns inside the file?
What are the data types of the field?
Discuss different forms of Data pre-processing. Give an example of
each.
arrow_forward
Structural in-dependence exists when it is
possible to make changes in the file structure
without affecting the application program's
ability to access the data.
Select one:
True
False
arrow_forward
Besides immutable, what are some of the other extended file attributes?
arrow_forward
Why do you feel file extensions are important? Please provide a
comprehensive justification for your position. You have the
option of naming three different file extensions in a folder that
is chosen at random on your computer. You are welcome to
additionally mention the names of any software programmes
that were used to build them, if you so choose. It should be
possible to open the majority of file extensions by right-clicking
on a file and selecting properties from the resulting pop-up
menu (Microsoft Windows environment).
arrow_forward
True or False: Logical extractions are bit-by-bit copiesof the file system, including deleted data.
arrow_forward
Purpose: Create and use a Database
Data Files:Use BS4, Regular Expressions or Pandas to read in the two data files for this assignment:
Co2.html:
200242002.292...
200252002.375...
200262002.458...
...
SeaLevel.csv
2002.3797,3.43000,1.23000,,
2002.4069,1.13000,0.33000,,
2002.4340,-5.67000,-2.17000,,
arrow_forward
This article will offer an explanation of file extensions and the apps
that generate them. It is critical to have examples.
arrow_forward
What is the use of the .cbak file and how often should it be saved?
arrow_forward
Other than immutable, what other extended file properties are there?
arrow_forward
What is the use of the Bevco.xls file?
arrow_forward
Python
Find the P10 value of a dataset from a .csv file using your own code and without the use of the percentile function in Numpy or Pandas.
arrow_forward
What file extensions do razor views have?
arrow_forward
What is the significance of the Bevco.xls file?
arrow_forward
Learn about file extensions and how they affect your current content. It's logical.
arrow_forward
Python Programming - Write a CSV-to-JSON translator that expects the path to a CSV file as argument, and for each line in the file, prints out a JSON object encapsulating that record.
arrow_forward
Guidelines
The input and the output of the program will be from & to files.
For the input file: o The program will take the file name as an argument (args [0]).
For the output file: o The program will take the output file name as an argument (args [1]).
You are free to explore the input and output methods as you develop the solution to the assignment; but before the submission, you have to prepare all the programs to take the input from a file in (args [0]) and print the output to the file in (args [1]).
Attached to this assignment are sample input and output files for each question. Those files have the same format as the files that your program will be tested through. So, please adhere to the format in those files, and make sure that your code can read and write the data given in that format.
arrow_forward
A master file containing nearly indestructible data is completely useless.
arrow_forward
ASSEMBLY
In order to link different .asm files together, you need to include them into the main .asm file that contains your main procedure.
True
False
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
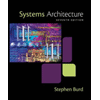
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Related Questions
- You should offer a way to enhance the file's organisation.arrow_forwardQuestion No: 2012123nt505 2This is a subjective question, hence you have to write your answer in the Text-Field given below. 76610 A team of engineers is designing a bridge to span the Podunk River. As part of the design process, the local flooding data must be analyzed. The following information on each storm that has been recorded in the last 40 years is stored in a file: the location of the source of the data, the amount of rainfall (in inches), and the duration of the storm (in hours), in that order. For example, the file might look like this: 321 2.4 1.5 111 33 12.1 etc. a. Create a data file. b. Write the first part of the program: design a data structure to store the storm data from the file, and also the intensity of each storm. The intensity is the rainfall amount divided by the duration. c. Write a function to read the data from the file (use load), copy from the matrix into a vector of structs, and then calculate the intensities. (2+3+3)arrow_forwardApart from immutability, what other extended file attributes are there?arrow_forward
- Apart from being immutable, what other extended file attributes are available to choose from?arrow_forwardAssignment 2 Directions Please choose Option 1 or Option 2. You do not need to do both. Option 1: Please write a short paper based on Module 2 Hardware Components. Anything in the module can be a topic. Write a summary paper as follows: • Prepare a Word document with a minimum of 3 pages (not including title and references pages) and a minimum of 3 solid references. Be sure to use APA Guidelines for references. • Make sure to save the file in the following format: FirstName_LastName_Paper.doc(x) • Upload the completed file as an attachment to this assignment. . Option 2: 1. Create an algorithm to add two integer numbers. 2. Implement the program in a high level language like C or Java. It does not need to run for me, but the code should be included in a text document called FirstnameLastnameHLA2.txt in your assignment submission. 3. Implement the program in MIPSzy Assembly language. Use the high level code as comments to the right of the Assembly code as the textbook does. 4. If you…arrow_forwardOther from being immutable, what other extended file attributes are available to choose from?arrow_forward
- It would be helpful if you could provide a suggestion to enhance the way a file is organized.arrow_forwardComputer Science The size of a file as reported by the ls -l listing is 10323433 bytes. However, the size of the same file when reported by the du command is 1000 blocks where a block is 512 bytes. Explain the discrepancy between these two sizes.arrow_forwardWhat were the intentions behind the creation of the Bevco.xls file?arrow_forward
- You need to provide a means through which the file's organization might be improved.arrow_forwardCPE162_exer3_6 [Read-Only] [Compatibility Mode] - Word Ace Jan C. Lape File Home Insert Design Layout References Mailings Review View Help O Tell me what you want to do & Share X Cut P Find - Arial - 11 A A Aa - AaBbCc AAB6CCL AaBbC AaBbCc[ AaBbCcl AaBbCcD akc Replace A Select - EE Copy BI U Paste - abe x, x A- aly , A 1 Caption Emphasis Heading 1 1 Normal Strong Subtitle - Format Painter Clipboard Font Paragraph Styles Editing Computer Engineering Department Laboratory Activity Form Course Number ES 085 Course Title Computer Programming 2 Topics Covered: Array Implement a program using the single-subscripted array and its operations with emphasis on the creation of programmer-defined data types and the use of separate files for the definition - .h, implementation - .c and client code - .c. Objectives: Description Airline Reservation System A small airline has just purchased a computer for its new automated reservations system. The president has asked you to program the new system. You…arrow_forwardWhich specific purpose does the Bevco.xls file serve?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
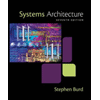
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning