1 – Assignment Following on the success of homework 1, your mother has asked you to augment your tag sale price recording program to save all of the data to files. She noticed that if you close the program around lunchtime, all of the sales data is lost. She wants it to be permanent. Change your program from homework 1 to do two new things: • Upon opening the program, load data from a file (name the file whatever you want) into the array in which you store prices. Update the statistics in the GUI using the loaded data. • Continue to run the program normally while collecting data… • Before closing the program, write all of the collected data to the file. If you already loaded all data from the file into the array when you started the program, then all of that data and the new data collected should be in the array. Overwrite the existing file with the new data File format – The simplest method to store data in the file would be entering a newline after each price, so that your file looks like this: 3.50 0.50 2.00 25.00 Notes: • Make sure to open a file in read mode when reading… • Make sure to open a file in write mode when writing. If overwriting an existing file, make sure you set the correct flags, otherwise you will be appending to the file • Make sure you close the files after you are done reading or writing to them. When a file is opened, the file is locked from other programs using it at all, until you close it… This is true even for your own program, you can’t open the same file again without closing it first. Visual basic/visual studio Current code Imports System.IO Public Class FrmMain Dim prices(0) As Double ' Array to store prices of items sold Dim totalSales As Double ' Total amount of money collected Dim totalItems As Integer ' Total number of items sold Dim averageSalePrice As Double ' Average sale price Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click Me.Close() End Sub Private Sub btnSubmitPrice_Click(sender As Object, e As EventArgs) Handles btnSubmitPrice.Click Dim price As Double = InputBox("Enter Price: ", "Enter a Number") ' Add the price to the array and update the statistics ReDim Preserve prices(prices.Length + 1) prices(prices.Length - 1) = price totalSales += price totalItems += 1 averageSalePrice = totalSales / totalItems ' Display the statistics lblTotalSales.Text = "Total Sales: " + totalSales.ToString() lblTotalItems.Text = "Total Items: " + totalItems.ToString() lblAverageSalePrice.Text = "Average Sale Price: " + averageSalePrice.ToString() End Sub End Class
1 – Assignment
Following on the success of homework 1, your mother has asked you to augment your tag sale price
recording
lunchtime, all of the sales data is lost. She wants it to be permanent.
Change your program from homework 1 to do two new things:
• Upon opening the program, load data from a file (name the file whatever you want) into the
array in which you store prices. Update the statistics in the GUI using the loaded data.
• Continue to run the program normally while collecting data…
• Before closing the program, write all of the collected data to the file. If you already loaded all
data from the file into the array when you started the program, then all of that data and the
new data collected should be in the array. Overwrite the existing file with the new data
File format – The simplest method to store data in the file would be entering a newline after each price,
so that your file looks like this:
3.50
0.50
2.00
25.00
Notes:
• Make sure to open a file in read mode when reading…
• Make sure to open a file in write mode when writing. If overwriting an existing file, make sure
you set the correct flags, otherwise you will be appending to the file
• Make sure you close the files after you are done reading or writing to them. When a file is
opened, the file is locked from other programs using it at all, until you close it… This is true even
for your own program, you can’t open the same file again without closing it first.
Visual basic/visual studio
Current code
Imports System.IO
Public Class FrmMain
Dim prices(0) As Double ' Array to store prices of items sold
Dim totalSales As Double ' Total amount of money collected
Dim totalItems As Integer ' Total number of items sold
Dim averageSalePrice As Double ' Average sale price
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
Me.Close()
End Sub
Private Sub btnSubmitPrice_Click(sender As Object, e As EventArgs) Handles btnSubmitPrice.Click
Dim price As Double = InputBox("Enter Price: ", "Enter a Number")
' Add the price to the array and update the statistics
ReDim Preserve prices(prices.Length + 1)
prices(prices.Length - 1) = price
totalSales += price
totalItems += 1
averageSalePrice = totalSales / totalItems
' Display the statistics
lblTotalSales.Text = "Total Sales: " + totalSales.ToString()
lblTotalItems.Text = "Total Items: " + totalItems.ToString()
lblAverageSalePrice.Text = "Average Sale Price: " + averageSalePrice.ToString()
End Sub
End Class


Step by step
Solved in 2 steps with 3 images

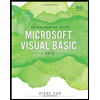
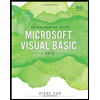
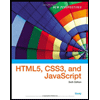