A fairly common algorithmic task is to process some data set in reverse order. Typically you put some data in temporary storage, then take it out, always in a Last-In, First-Out (LIFO) order. A stack is the data structure that was invented to help manage this process. A real-world example of a stack is the dispenser of the trays in the lunch room. You always take the top tray from the top, never from the middle or the bottom. Similarly, the lunch workers always put trays on the top, never at the middle or bottom. For this problem, you are going to determine whether the grouping symbols--parentheses, brackets, curly braces, etc.--in an arithmetic expression, such as [(5+7)*3], match each other. Here are all matching group symbols: (), 0, 0, and <>. For this problem, you can ignore all digits and operands. Study the following cases to examine how matching symbols are evaluated. Parentheses Match 5+7 true (5+7) true )5+7( false ((5+7)*3) true <{5+7}*3> true [(5+7)*]3 true (5+7)*3 true 5+(7*3) true ((5+7)*3 false [(5+7]*3) false [(5+7)*3]) false ([(5+7)*3] false <50 +{ 37 - {3*2}} +[19+ {8*9}]> true Please implement the following method: public static boolean checkParen (String s) { //implement this method to return true if grouping symbols match each other, otherwise return false. }
A fairly common algorithmic task is to process some data set in reverse order. Typically you put some data in temporary storage, then take it out, always in a Last-In, First-Out (LIFO) order. A stack is the data structure that was invented to help manage this process. A real-world example of a stack is the dispenser of the trays in the lunch room. You always take the top tray from the top, never from the middle or the bottom. Similarly, the lunch workers always put trays on the top, never at the middle or bottom. For this problem, you are going to determine whether the grouping symbols--parentheses, brackets, curly braces, etc.--in an arithmetic expression, such as [(5+7)*3], match each other. Here are all matching group symbols: (), 0, 0, and <>. For this problem, you can ignore all digits and operands. Study the following cases to examine how matching symbols are evaluated. Parentheses Match 5+7 true (5+7) true )5+7( false ((5+7)*3) true <{5+7}*3> true [(5+7)*]3 true (5+7)*3 true 5+(7*3) true ((5+7)*3 false [(5+7]*3) false [(5+7)*3]) false ([(5+7)*3] false <50 +{ 37 - {3*2}} +[19+ {8*9}]> true Please implement the following method: public static boolean checkParen (String s) { //implement this method to return true if grouping symbols match each other, otherwise return false. }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help with this Java problem as it's explained in the image below:
import java.util.*;
public class Main {
public static boolean checkParen(String s) {
//implement this method to return true if grouping symbols match
//each other, otherwise return false.
/* Type your code here. */
}
}
![A fairly common algorithmic task is to process some data set in reverse order. Typically you put some data in temporary storage, then take
it out, always in a Last-In, First-Out (LIFO) order. A stack is the data structure that was invented to help manage this process. A real-world
example of a stack is the dispenser of the trays in the lunch room. You always take the top tray from the top, never from the middle or the
bottom. Similarly, the lunch workers always put trays on the top, never at the middle or bottom. For this problem, you are going to determine
whether the grouping symbols--parentheses, brackets, curly braces, etc.--in an arithmetic expression, such as [(5+7)*3], match each other.
Here are all matching group symbols: (), 0, 0, and <>. For this problem, you can ignore all digits and operands. Study the following cases to
examine how matching symbols are evaluated.
Parentheses
Match
5+7
true
(5+7)
true
)5+7(
false
((5+7)*3)
true
<{5+7}*3>
true
[(5+7)*]3
true
(5+7)*3
true
5+(7*3)
true
((5+7)*3
false
[(5+7]*3)
false
[(5+7)*3])
false
([(5+7)*3]
false
<50 +{ 37 - {3*2}} +[19+ {8*9}]> true
Please implement the following method:
public static boolean checkParen (String s) {
//implement this method to return true if grouping symbols match each other, otherwise
return false.
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e135d5c-f867-4afd-9f0d-b505f4f19664%2Fba82e751-78f3-4d0e-bd25-ea0fcd7e8e37%2F4s5dtg_processed.png&w=3840&q=75)
Transcribed Image Text:A fairly common algorithmic task is to process some data set in reverse order. Typically you put some data in temporary storage, then take
it out, always in a Last-In, First-Out (LIFO) order. A stack is the data structure that was invented to help manage this process. A real-world
example of a stack is the dispenser of the trays in the lunch room. You always take the top tray from the top, never from the middle or the
bottom. Similarly, the lunch workers always put trays on the top, never at the middle or bottom. For this problem, you are going to determine
whether the grouping symbols--parentheses, brackets, curly braces, etc.--in an arithmetic expression, such as [(5+7)*3], match each other.
Here are all matching group symbols: (), 0, 0, and <>. For this problem, you can ignore all digits and operands. Study the following cases to
examine how matching symbols are evaluated.
Parentheses
Match
5+7
true
(5+7)
true
)5+7(
false
((5+7)*3)
true
<{5+7}*3>
true
[(5+7)*]3
true
(5+7)*3
true
5+(7*3)
true
((5+7)*3
false
[(5+7]*3)
false
[(5+7)*3])
false
([(5+7)*3]
false
<50 +{ 37 - {3*2}} +[19+ {8*9}]> true
Please implement the following method:
public static boolean checkParen (String s) {
//implement this method to return true if grouping symbols match each other, otherwise
return false.
}
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
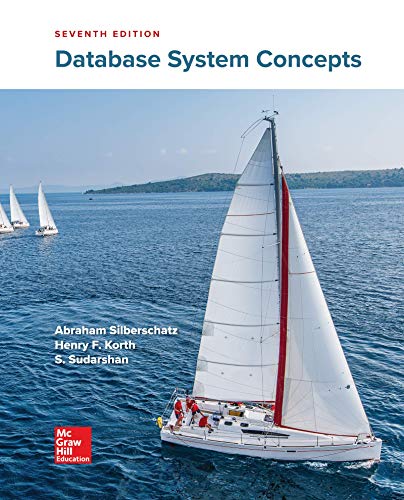
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
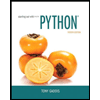
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
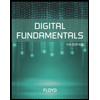
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
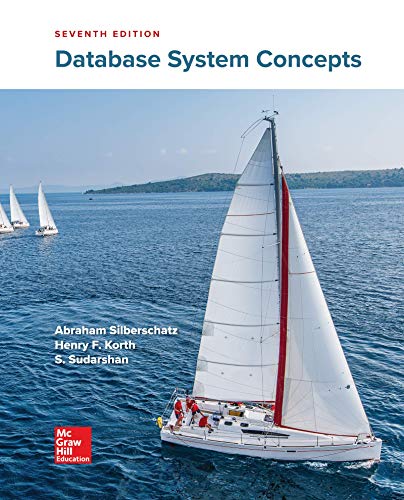
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
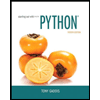
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
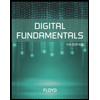
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
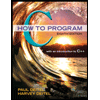
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
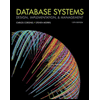
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
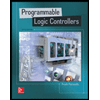
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education