A Python dictionary that returns a list of values for each key. The dictionary will contain information about my favorite books, including the title, author, and year of publication. The key will be the title of the book, and the values will be a list containing the author and the year of publication. favorite_books = { "The Great Gatsby": ["F. Scott Fitzgerald", 1925], "To Kill a Mockingbird": ["Harper Lee", 1960], "1984": ["George Orwell", 1949] } NOTE: You can replace the book details as your need in above code. Next, modify the invert_dict function from Section 11.5 of "Think Python" to invert my dictionary. The modified function will need to turn each of the list items into separate keys in the inverted dictionary. def invert_dict(d): inverse = dict() for key in d: vals = d[key] for val in vals: if val not in inverse: inverse[val] = [key] else: inverse[val].append(key) return inverse Here, the function takes a dictionary as an argument and returns an inverted dictionary where the keys are the values of the original dictionary, and the values are a list of keys from the original dictionary that had the corresponding value. Finally, I will run the invert_dict function on my favorite_books dictionary and print the original and inverted dictionaries. print("Original dictionary:") print(favorite_books) inverted_dict = invert_dict(favorite_books) print("Inverted dictionary:") print(inverted_dict) Python Code and Explanation: favorite_books = { "The Great Gatsby": ["F. Scott Fitzgerald", 1925], "To Kill a Mockingbird": ["Harper Lee", 1960], "1984": ["George Orwell", 1949] } def invert_dict(d): inverse = dict() for key in d: vals = d[key] for val in vals: if val not in inverse: inverse[val] = [key] else: inverse[val].append(key) return inverse print("Original dictionary:") print(favorite_books) inverted_dict = invert_dict(favorite_books) print("Inverted dictionary:") print(inverted_dict) This program defines a dictionary named favorite_books that stores information about three books: "The Great Gatsby", "To Kill a Mockingbird", and "1984". Each book is associated with a list of two items: the author's name and the year of publication. The program then defines a function invert_dict(d) that takes a dictionary d as input and returns a new dictionary where the keys and values of the input dictionary are inverted. Specifically, each value in the input dictionary becomes a key in the output dictionary, and the corresponding keys in the input dictionary become the values in the output dictionary. If multiple keys in the input dictionary have the same value, the corresponding keys are stored in a list as the value in the output dictionary. The program then applies the invert_dict() function to the favorite_books dictionary and assigns the result to a new variable named inverted_dict. Finally, the program prints the original and inverted dictionaries. The output of the program shows the original and inverted dictionaries. The original dictionary lists information about three books. The inverted dictionary lists the authors and publication years as keys, with the corresponding book titles as the values. Note that since the publication years of "The Great Gatsby" and "1984" are the same, their corresponding book titles are stored as a list under the key "1949". Modify your above program to read dictionary items from a file and write the inverted dictionary to a file. Include the following in your Learning Journal submission: The input file for your original dictionary (with at least six items). The Python program to read from a file, invert the dictionary, and write to a different file. The output file for your inverted dictionary. The code and its output must be explained technically. The explanation can be provided before or after the code, or in the form of comments within the code. For code modification type of questions, always mention or clearly highlight the part which is modified, along with the reason stated, as a code comment. The descriptive part of your response must be at least 200 words.
A Python dictionary that returns a list of values for each key. The dictionary will contain information about my favorite books, including the title, author, and year of publication. The key will be the title of the book, and the values will be a list containing the author and the year of publication.
favorite_books = {
"The Great Gatsby": ["F. Scott Fitzgerald", 1925],
"To Kill a Mockingbird": ["Harper Lee", 1960],
"1984": ["George Orwell", 1949]
}
NOTE: You can replace the book details as your need in above code.
Next, modify the invert_dict function from Section 11.5 of "Think Python" to invert my dictionary. The modified function will need to turn each of the list items into separate keys in the inverted dictionary.
def invert_dict(d):
inverse = dict()
for key in d:
vals = d[key]
for val in vals:
if val not in inverse:
inverse[val] = [key]
else:
inverse[val].append(key)
return inverse
Here, the function takes a dictionary as an argument and returns an inverted dictionary where the keys are the values of the original dictionary, and the values are a list of keys from the original dictionary that had the corresponding value.
Finally, I will run the invert_dict function on my favorite_books dictionary and print the original and inverted dictionaries.
print("Original dictionary:")
print(favorite_books)
inverted_dict = invert_dict(favorite_books)
print("Inverted dictionary:")
print(inverted_dict)
Python Code and Explanation:
favorite_books = {
"The Great Gatsby": ["F. Scott Fitzgerald", 1925],
"To Kill a Mockingbird": ["Harper Lee", 1960],
"1984": ["George Orwell", 1949]
}
def invert_dict(d):
inverse = dict()
for key in d:
vals = d[key]
for val in vals:
if val not in inverse:
inverse[val] = [key]
else:
inverse[val].append(key)
return inverse
print("Original dictionary:")
print(favorite_books)
inverted_dict = invert_dict(favorite_books)
print("Inverted dictionary:")
print(inverted_dict)
This
The program then defines a function invert_dict(d) that takes a dictionary d as input and returns a new dictionary where the keys and values of the input dictionary are inverted. Specifically, each value in the input dictionary becomes a key in the output dictionary, and the corresponding keys in the input dictionary become the values in the output dictionary. If multiple keys in the input dictionary have the same value, the corresponding keys are stored in a list as the value in the output dictionary.
The program then applies the invert_dict() function to the favorite_books dictionary and assigns the result to a new variable named inverted_dict. Finally, the program prints the original and inverted dictionaries.
The output of the program shows the original and inverted dictionaries. The original dictionary lists information about three books. The inverted dictionary lists the authors and publication years as keys, with the corresponding book titles as the values. Note that since the publication years of "The Great Gatsby" and "1984" are the same, their corresponding book titles are stored as a list under the key "1949".
Modify your above program to read dictionary items from a file and write the inverted dictionary to a file.
Include the following in your Learning Journal submission:
- The input file for your original dictionary (with at least six items).
- The Python program to read from a file, invert the dictionary, and write to a different file.
- The output file for your inverted dictionary.

Step by step
Solved in 3 steps

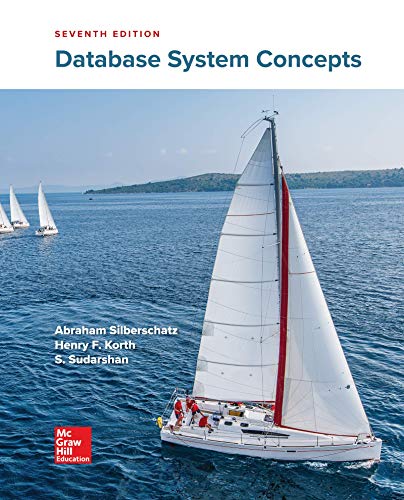
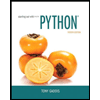
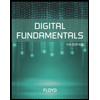
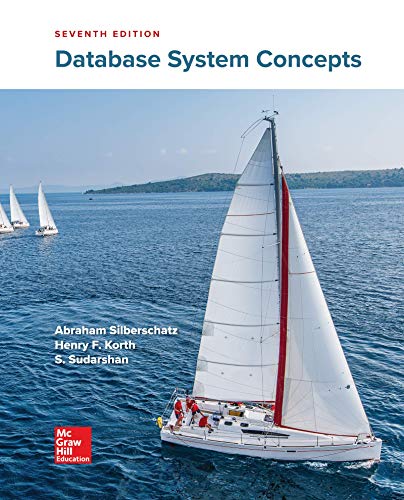
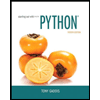
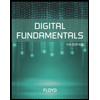
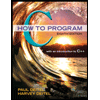
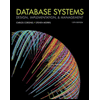
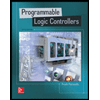