Add the following methos to the class Person(code bellow) in Python: a) Add a method isDescendant(personID) to Person which takes a person identifier string as an argument and returns True if the identified person is an descendant of self or False if this is not the case. The answer should be True when the identified person is self. b) Add a method printAncestors() to Person that does what its name suggests. You aren't likely to manage to make this look as good as the output of printDescendants, but each line should include some indication how many generations up the tree the ancestor is. Just starting the line with a number and appropriate indentation is sufficient. from collections import namedtuple class Person(): # Stores info about a single person # Created when an Individual (INDI) GEDCOM record is processed. #------------------------------------------------------------------- def __init__(self,ref): # Initializes a new Person object, storing the string (ref) by # which it can be referenced. self._id = ref self._asSpouse = [] # use a list to handle multiple families self._asChild = None def addName(self, names): # Extracts name parts from a list of names and stores them self._given = names[0] self._surname = names[1] self._suffix = names[2] def addIsSpouse(self, famRef): # Adds the string (famRef) indicating family in which this person # is a spouse, to list of any other such families self._asSpouse.append(famRef) def addIsChild(self, famRef): # Stores the string (famRef) indicating family in which this person # is a child self._asChild = famRef def printDescendants(self, prefix=''): # print info for this person and then call method in Family print(prefix + self.name()) # recursion stops when self is not a spouse for fam in self._asSpouse: families[fam].printFamily(self._id,prefix) def name (self): # returns a simple name string return self._given + ' ' + self._surname.upper()\ + ' ' + self._suffix def treeInfo (self): # returns a string representing the structure references included in self if self._asChild: # make sure value is not None childString = ' | asChild: ' + self._asChild else: childString = '' if self._asSpouse != []: # make sure _asSpouse list is not empty # Use join() to put commas between identifiers for multiple families # No comma appears if there is only one family on the list spouseString = ' | asSpouse: ' + ','.join(self._asSpouse) else: spouseString = '' return childString + spouseString def eventInfo(self): ## add code here to show information from events once they are recognized return '' def __str__(self): # Returns a string representing all info in a Person instance # When treeInfo is no longer needed for debugging it can return self.name() \ + self.eventInfo() \ + self.treeInfo() ## Comment out when not needed for debugging # end of class Person #----------------------------------------------------------------------- # Declare a named tuple type used by Family to create a list of spouses Spouse = namedtuple('Spouse',['personRef','tag']) class Family(): # Stores info about a family # An instance is created when an Family (FAM) GEDCOM record is processed. #------------------------------------------------------------------- def __init__(self, ref): # Initializes a new Family object, storing the string (ref) by # which it can be referenced. self._id = ref self._spouse1 = None self._spouse2 = None self._children = [] def addSpouse(self, personRef, tag): # Stores the string (personRef) indicating a spouse in this family newSpouse = Spouse(personRef, tag) if self._spouse1 == None: self._spouse1 = newSpouse else: self._spouse2 = newSpouse def addChild(self, personRef): # Adds the string (personRef) indicating a new child to the list self._children.append(personRef)
Add the following methos to the class Person(code bellow) in Python:
a) Add a method isDescendant(personID) to Person which takes a person identifier string as an argument and returns True if the identified person is an descendant of self or False if this is not the case. The answer should be True when the identified person is self.
b) Add a method printAncestors() to Person that does what its name suggests. You aren't likely to manage to make this look as good as the output of printDescendants, but each line should include some indication how many generations up the tree the ancestor is. Just starting the line with a number and appropriate indentation is sufficient.
from collections import namedtuple
class Person():
# Stores info about a single person
# Created when an Individual (INDI) GEDCOM record is processed.
#-------------------------------------------------------------------
def __init__(self,ref):
# Initializes a new Person object, storing the string (ref) by
# which it can be referenced.
self._id = ref
self._asSpouse = [] # use a list to handle multiple families
self._asChild = None
def addName(self, names):
# Extracts name parts from a list of names and stores them
self._given = names[0]
self._surname = names[1]
self._suffix = names[2]
def addIsSpouse(self, famRef):
# Adds the string (famRef) indicating family in which this person
# is a spouse, to list of any other such families
self._asSpouse.append(famRef)
def addIsChild(self, famRef):
# Stores the string (famRef) indicating family in which this person
# is a child
self._asChild = famRef
def printDescendants(self, prefix=''):
# print info for this person and then call method in Family
print(prefix + self.name())
# recursion stops when self is not a spouse
for fam in self._asSpouse:
families[fam].printFamily(self._id,prefix)
def name (self):
# returns a simple name string
return self._given + ' ' + self._surname.upper()\
+ ' ' + self._suffix
def treeInfo (self):
# returns a string representing the structure references included in self
if self._asChild: # make sure value is not None
childString = ' | asChild: ' + self._asChild
else: childString = ''
if self._asSpouse != []: # make sure _asSpouse list is not empty
# Use join() to put commas between identifiers for multiple families
# No comma appears if there is only one family on the list
spouseString = ' | asSpouse: ' + ','.join(self._asSpouse)
else: spouseString = ''
return childString + spouseString
def eventInfo(self):
## add code here to show information from events once they are recognized
return ''
def __str__(self):
# Returns a string representing all info in a Person instance
# When treeInfo is no longer needed for debugging it can
return self.name() \
+ self.eventInfo() \
+ self.treeInfo() ## Comment out when not needed for debugging
# end of class Person
#-----------------------------------------------------------------------
# Declare a named tuple type used by Family to create a list of spouses
Spouse = namedtuple('Spouse',['personRef','tag'])
class Family():
# Stores info about a family
# An instance is created when an Family (FAM) GEDCOM record is processed.
#-------------------------------------------------------------------
def __init__(self, ref):
# Initializes a new Family object, storing the string (ref) by
# which it can be referenced.
self._id = ref
self._spouse1 = None
self._spouse2 = None
self._children = []
def addSpouse(self, personRef, tag):
# Stores the string (personRef) indicating a spouse in this family
newSpouse = Spouse(personRef, tag)
if self._spouse1 == None:
self._spouse1 = newSpouse
else: self._spouse2 = newSpouse
def addChild(self, personRef):
# Adds the string (personRef) indicating a new child to the list
self._children.append(personRef)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

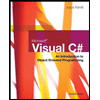
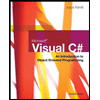