// ADD YOUR INSTANCE VARIABLES HERE public DailySalesTally() { // FILL IN /** * Adds a purchase to the tally * eparam p the purchase public void addPurchase(Purchase p) { // FILL IN /** * Calculates the total for the day for the client with the given name @param clientName @return name of the client for whom to calculate the total total for the given client public double get TotalForclient(String clientName) { return -1.0; // FIX ME /** culates the grand tota. the day for purchases * @return the grand total for the day for all purchases public double getGrandTotal() { return -1.0; // FIX ME Represents a purchase made in Robert's doggy spa/boutique public class Purchase { // ADD YOUR INSTANCE VARIABLES HERE Constructs a new Purchase @param description @param clientName * eparam price * eparam boutiquePurchase whether or not this was a boutique purchase description of the purchase name of client who made the purchase price of the purchase public Purchase(String description, String clientName, double price, boolean boutiquePurchase) { // FILL IN public String getDescription() { return ""; // FIX ME public String getclientName() ( return ""; // FIX ME public double getPrice() { return -1.0; // FIX ME public boolean isBoutiquePurchase() { return false; // FIX ME
// ADD YOUR INSTANCE VARIABLES HERE public DailySalesTally() { // FILL IN /** * Adds a purchase to the tally * eparam p the purchase public void addPurchase(Purchase p) { // FILL IN /** * Calculates the total for the day for the client with the given name @param clientName @return name of the client for whom to calculate the total total for the given client public double get TotalForclient(String clientName) { return -1.0; // FIX ME /** culates the grand tota. the day for purchases * @return the grand total for the day for all purchases public double getGrandTotal() { return -1.0; // FIX ME Represents a purchase made in Robert's doggy spa/boutique public class Purchase { // ADD YOUR INSTANCE VARIABLES HERE Constructs a new Purchase @param description @param clientName * eparam price * eparam boutiquePurchase whether or not this was a boutique purchase description of the purchase name of client who made the purchase price of the purchase public Purchase(String description, String clientName, double price, boolean boutiquePurchase) { // FILL IN public String getDescription() { return ""; // FIX ME public String getclientName() ( return ""; // FIX ME public double getPrice() { return -1.0; // FIX ME public boolean isBoutiquePurchase() { return false; // FIX ME
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Java. Please use the template, thank you!

Transcribed Image Text:2
3 public class DailySalesTally {
4
// ADD YOUR INSTANCE VARIABLES HERE
public DailySalesTally() {
// FILL IN
70
8.
9.
}
10
11
120
/**
13
* Adds a purchase to the tally
14
@param p the purchase
*/
public void addPurchase(Purchase p) {
// FILL IN
}
*
15
16
170
18
19
20
21
220
/**
23
* Calculates the total for the day for the client with the given name
*
24
@param clientName
@return
*/
25
name of the client for whom to calculate the total
26
total for the given client
27
public double getTotalForClient(String clientName) {
return -1.0; // FIX ME
}
280
29
30
31
32
330
/**
34
* Calculates the grand total for the day for all purchases
35
@return the grand total for the day for all purchases
* /
public double getGrandTotal() {
*
36
37
38e
39
return -1.0; // FIX ME
}
41 }
40
30 /**|
Represents a purchase made in Robert's doggy spa/boutique
*/
6 public class Purchase {
4
7
8.
// ADD YOUR INSTANCE VARIABLES HERE
9.
10
110
/**
12
* Constructs a new Purchase
13
*
@param description
@param clientName
@param price
@param boutiquePurchase whether or not this was a boutique purchase
*/
description of the purchase
name of client who made the purchase
price of the purchase
14
15
16
17
18
public Purchase(String description, String clientName, double price, boolean boutiquePurchase) {
// FILL IN
}
190
20
21
22
23
240
25
26
27
public String getDescription() {
return ""; // FIX ME
28
290
30
public String getClientName() {
return ""; // FIX ME
31
}
32
33
340
35
public double getPrice() {
return -1.0; // FIX ME
36
}
37
38
390
public boolean isBoutiquePurchase() {
return false; // FIX ME
}
40
41
42 }

Transcribed Image Text:Problem 8: Purchase and DailySalesTally.
Robert the dog has decided to open a doggy spa and playground where all his doggy friends can come to play, get
groomed, and even shop for specialty doggy boutique items! To encourage customers to spend more money in his
business, Robert wants to implement a customer loyalty program in which, whenever a client makes a purchase, they
get a 10% discount on any other purchases they make on that same day.
In addition, Robert really wants to grow his boutique business, so for a limited time, he is giving a 5% discount on all
boutique purchases.
Implement a class Purchase to describe a service or item purchased in Robert's business. Use this template:
Purchase.java I
A daily sales tally holds a collection of Purchase objects (hint: use an array list). Implement a class DailySalesTally
to represent this, using this template: DailySalesTally.java
Example:
= new DailySalesTally ();
tally.addPurchase (new Purchase ("Day care", "Fluffy", 25.0, false));
DailysalesTally tally
tally.addPurchase (new Purchase ("Bow tie collar", "Carl", 15.75, true));
tally.getTotalForClient ("Fluffy"); // returns 25.0
tally.getTotalForClient ("Carl"); // returns 14.96
tally.getTotalForClient ("carl"); // returns 0
tally.getTotalForClient ("Abby"); // returns 0
tally.getGrandTotal (); // returns 39.96
tally.addPurchase (new Purchase ("Bath and blow dry", "Fluffy", 39.95, false));
tally.addPurchase (new Purchase ("Dog biscuits", "Carl", 6.25, true));
tally.getTotalForClient ("Fluffy"); // returns 60.96
tally.getTotalForClient ("Carl"); // returns 20.30
tally.getGrandTotal (); // returns 81.26
tally.addPurchase (new Purchase ("Nail trim", "Abby", 12.25, false));
tally.addPurchase (new Purchase ("Argyle sweater", "Roger", 99.99, true));
tally.getTotalForClient ("Fluffy"); // returns 60.96
tally.getTotalForClient ("Carl"); // returns 20.30
tally.getTotalForClient ("Abby"); // returns 12.25
tally.getTotalForClient ("Roger"); || returns 94.99
tally.getGrandTotal (); // returns 188.50
tally.addPurchase (new Purchase ("Bath and blow dry", "Carl", 39.95, false));
tally.addPurchase (new Purchase ("Dog booties", "Fluffy", 27.13, true));
tally.getTotalForClient ("Fluffy"); // returns 84.15
tally.getTotalForClient ("Carl"); // returns 56.26
tally.getTotalForClient ("Abby"); // returns 12.25
tally.getTotalForClient ("Roger"); // returns 94.99
tally.getGrandTota1(); // returns 247.65
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 4 images

Recommended textbooks for you
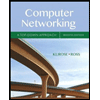
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
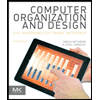
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
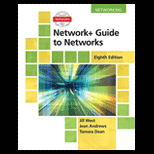
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
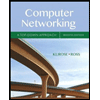
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
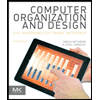
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
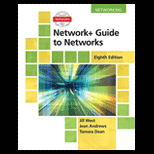
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
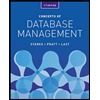
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
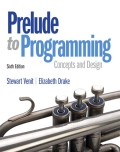
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
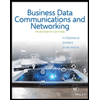
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY