All I need is the main.cpp file Programming Specifications: Task: Two files have been provided (wordcounter.h and wordcount.cpp). You must open a text file, read in each word until end of file, add each word to the WordCount library and print the report. Your program must request a filename of a text file that contains words to count. You must check to see if it is a valid file and issue an error message if not and request a new file name until a valid one is entered. You must have at least one function that either passes in a parameter and at least one function that returns a value. You can have a single routine that does both. You must declare the class object properly, use AddWord() to add the word to the catalog and use PrintSummary() to produce the output. Design Considerations: The file will contain only words (no punctuation or special characters) less than or equal to 13 letters. There will be no limit to the number of words. You must open the file and read each individual word using standard input (cin) until the end-of-file is detected calling AddWord() class member function to add the word to the class library. The file must be closed properly. =================================================================== wordcounter.cpp #include #include #include #include "wordcounter.h" using namespace std; // Basic Double Link List. a Head and tail and set them to // point to each other. Set Wordobject to low in Head and High value // in the tail so all other words are inserted in between. WordCounter::WordCounter() { Head = new struct WordStruct; Tail = new struct WordStruct; Head->Wordobject = " "; // 15 spaces, low value Head->Counter=1; Tail->Wordobject = "~~~~~~~~~~~~~~~"; // 15 ~, very high value Tail->Counter=1; Head->Prev = NULL; Head->Next = Tail; Tail->Prev = Head; Tail->Next = NULL; }; // Member Function: WordCounter // Description: Accept a word string, check the list to see if // exists, increment the counter if it does, make it uppercase // and add it to the list of words in alphabetical order. // Parameter List: const string word: Word to be inserted. void WordCounter::Addword (const string word) { string tempword = Uppercase(word); static struct WordStruct * NewWord; if (Findword(word)) { Curr->Counter++; return; } Curr = Head; while(tempword > Curr->Wordobject) Curr=Curr->Next; NewWord = new WordStruct; NewWord->Wordobject = tempword; NewWord->Counter = 1; NewWord->Next = Curr; NewWord->Prev = Curr->Prev; Curr->Prev = NewWord; NewWord->Prev->Next=NewWord; } // Member Function: Printsummary // Description: Print all of the words, count, and summary // information. // Parameter List: None void WordCounter::Printsummary() { int uniquewords=0; int totalwords=0; int remainder=0; int wordwidth=0; cout << "Word Counter Summary" << endl; cout << endl; cout << " Word Count" << endl; cout << " _______________ _____" << endl; cout << endl; Curr = Head->Next; while(Curr != Tail) { wordwidth = (15-Curr->Wordobject.length())/2; remainder = Curr->Wordobject.length()%2; for(int index=0; indexWordobject; cout <Counter<Counter; Curr = Curr->Next; } cout << endl; cout <<"Total Number of Words: " << totalwords << endl; cout <<"Total Number of Unique Words: " << uniquewords << endl; } // Member Function: FindWord // Description: Scans the list and checks to see if a word exists. // Parameter Description: const string word: string to be searched // for. bool WordCounter::Findword(const string word) { Curr = Head->Next; string tempstr = Uppercase(word); while(Curr != Tail) { if(tempstr == Curr->Wordobject) return true; Curr=Curr->Next; } return false; } // Member Function: Uppercase // Description: Makes a string upper case letters. // Parameter Description: const string word: Word to be changed to // uppercase. string WordCounter::Uppercase(string word) { int wlength = word.length(); for (int index=0; index < wlength; index++) word[index] = toupper(word[index]); return word; } =================================================================== wordcounter.h #ifndef WORDCOUNTER_H #define WORDCOUNTER_H #include #include using namespace std; // Class WordCounter: Allows the user to a WordCounter * // that catalogs the words and prints out summary of total * // words and total unique words in alphabetical order. * class WordCounter { public: WordCounter(); // Constructor void Addword(const string word); // Adds a word to list void Printsummary(); // Prints the report struct WordStruct { int Counter; string Wordobject; struct WordStruct * Prev; struct WordStruct * Next; }; private: struct WordStruct * Curr; // Points to the current record struct WordStruct * Head; // The Head of the list struct WordStruct * Tail; // The Tail of the list bool Findword(const string word); // Finds if a word exists in the list string Uppercase(string word); // Converts the word to upper case }; #endif
All I need is the main.cpp file
Programming Specifications:
Task: Two files have been provided (wordcounter.h and wordcount.cpp). You must open a text file, read in each word until end of file, add each word to the WordCount library and print the report.
- Your program must request a filename of a text file that contains words to count. You must check to see if it is a valid file and issue an error message if not and request a new file name until a valid one is entered.
- You must have at least one function that either passes in a parameter and at least one function that returns a value. You can have a single routine that does both.
- You must declare the class object properly, use AddWord() to add the word to the catalog and use PrintSummary() to produce the output.
Design Considerations:
- The file will contain only words (no punctuation or special characters) less than or equal to 13 letters. There will be no limit to the number of words.
- You must open the file and read each individual word using standard input (cin) until the end-of-file is detected calling AddWord() class member function to add the word to the class library.
- The file must be closed properly.
===================================================================
wordcounter.cpp
#include <iostream>
#include <string>
#include <iomanip>
#include "wordcounter.h"
using namespace std;
// Basic Double Link List. a Head and tail and set them to
// point to each other. Set Wordobject to low in Head and High value
// in the tail so all other words are inserted in between.
WordCounter::WordCounter()
{
Head = new struct WordStruct;
Tail = new struct WordStruct;
Head->Wordobject = " "; // 15 spaces, low value
Head->Counter=1;
Tail->Wordobject = "~~~~~~~~~~~~~~~"; // 15 ~, very high value
Tail->Counter=1;
Head->Prev = NULL;
Head->Next = Tail;
Tail->Prev = Head;
Tail->Next = NULL;
};
// Member Function: WordCounter
// Description: Accept a word string, check the list to see if
// exists, increment the counter if it does, make it uppercase
// and add it to the list of words in alphabetical order.
// Parameter List: const string word: Word to be inserted.
void WordCounter::Addword (const string word)
{
string tempword = Uppercase(word);
static struct WordStruct * NewWord;
if (Findword(word))
{ Curr->Counter++;
return;
}
Curr = Head;
while(tempword > Curr->Wordobject)
Curr=Curr->Next;
NewWord = new WordStruct;
NewWord->Wordobject = tempword;
NewWord->Counter = 1;
NewWord->Next = Curr;
NewWord->Prev = Curr->Prev;
Curr->Prev = NewWord;
NewWord->Prev->Next=NewWord;
}
// Member Function: Printsummary
// Description: Print all of the words, count, and summary
// information.
// Parameter List: None
void WordCounter::Printsummary()
{ int uniquewords=0;
int totalwords=0;
int remainder=0;
int wordwidth=0;
cout << "Word Counter Summary" << endl;
cout << endl;
cout << " Word Count" << endl;
cout << " _______________ _____" << endl;
cout << endl;
Curr = Head->Next;
while(Curr != Tail)
{ wordwidth = (15-Curr->Wordobject.length())/2;
remainder = Curr->Wordobject.length()%2;
for(int index=0; index<wordwidth; index++)
cout<< ' ' ;
cout <<Curr->Wordobject;
cout <<setw(10+wordwidth-remainder)<<right<<Curr->Counter<<endl;
uniquewords++;
totalwords = totalwords+ Curr->Counter;
Curr = Curr->Next;
}
cout << endl;
cout <<"Total Number of Words: " << totalwords << endl;
cout <<"Total Number of Unique Words: " << uniquewords << endl;
}
// Member Function: FindWord
// Description: Scans the list and checks to see if a word exists.
// Parameter Description: const string word: string to be searched
// for.
bool WordCounter::Findword(const string word)
{ Curr = Head->Next;
string tempstr = Uppercase(word);
while(Curr != Tail)
{
if(tempstr == Curr->Wordobject) return true;
Curr=Curr->Next;
}
return false;
}
// Member Function: Uppercase
// Description: Makes a string upper case letters.
// Parameter Description: const string word: Word to be changed to
// uppercase.
string WordCounter::Uppercase(string word)
{
int wlength = word.length();
for (int index=0; index < wlength; index++)
word[index] = toupper(word[index]);
return word;
}
===================================================================
wordcounter.h
#ifndef WORDCOUNTER_H
#define WORDCOUNTER_H
#include <iostream>
#include <string>
using namespace std;
// Class WordCounter: Allows the user to a WordCounter *
// that catalogs the words and prints out summary of total *
// words and total unique words in alphabetical order. *
class WordCounter {
public:
WordCounter(); // Constructor
void Addword(const string word); // Adds a word to list
void Printsummary(); // Prints the report
struct WordStruct {
int Counter;
string Wordobject;
struct WordStruct * Prev;
struct WordStruct * Next;
};
private:
struct WordStruct * Curr; // Points to the current record
struct WordStruct * Head; // The Head of the list
struct WordStruct * Tail; // The Tail of the list
bool Findword(const string word); // Finds if a word exists in the list
string Uppercase(string word); // Converts the word to upper case
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 9 images

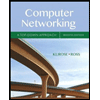
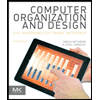
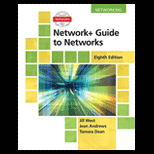
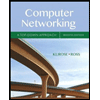
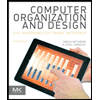
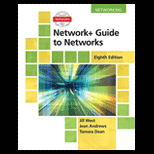
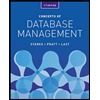
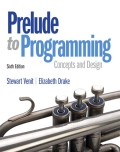
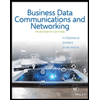