Any help is appreciated, I was able to complete the the first part about login but I am not sure how to load movies and ratings into table view Using GUI and Javafx Instructions: 1) Complete the login to read from the file and match with the correct username and password 2) Switch to the secondary screen 3) Load the Movies and the ratings associated with them to Table in the GUI This is what I have Log in class: import java.io.FileNotFoundException; import java.io.IOException; import java.util.ArrayList; import javafx.event.ActionEvent; import javafx.fxml.FXML; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.control.PasswordField; import javafx.scene.control.TextField; public class PrimaryController { public static int counter = 0; @FXML private TextField txtUserName; @FXML private Button btnLogin; @FXML private Label lblErrorMsg; @FXML private PasswordField txtPassword; private void switchToSecondary() throws IOException { App.setRoot("secondary"); } @FXML private void btnLogin_click(ActionEvent event) throws FileNotFoundException, IOException { try{ System.out.println(txtUserName.getText()); System.out.println(txtPassword.getText()); Utilities uti = new Utilities(); String line = uti.readLine("logininfo.txt", counter); String[] userInfo = line.split(","); System.out.println("User Name: " +userInfo[0]); System.out.println("User Password: " +userInfo[1]); //uti.writeLine("logininfo.txt", "sample,test2"); System.out.println(line); if(counter %2 == 0) lblErrorMsg.setText("Thank you for clicking on Login button"); else lblErrorMsg.setText(""); while(line != ""){ System.out.println("User Name: " +userInfo[0]); System.out.println("User Password: " +userInfo[1]); userInfo = line.split(","); if ((txtUserName.getText().equals(userInfo[0])) && (txtPassword.getText().equals(userInfo[1]))){ App.setRoot("secondary"); } counter++; line = uti.readLine("logininfo.txt", counter); System.out.println(counter); } } catch(Exception ex){ System.out.println(ex.getMessage()); } } } import java.io.File; import java.io.FileNotFoundException; import java.io.FileWriter; import java.io.IOException; import java.util.Scanner; /** * * @author itlabs */ public class Utilities { public String readLine(String fileName, int lineNumber) throws FileNotFoundException{ String Directory = "T:\\Documents\\NetBeansProjects\\MovieRatingSystem\\src\\main\\resources\\com\\mycompany\\movieratingsystem\\"; System.out.println(Directory + fileName); try{ File file = new File(Directory+ fileName); Scanner scanner = new Scanner(file); int lineCounter = 0; String Line = ""; while(scanner.hasNextLine()){ if(lineCounter == lineNumber){ Line = scanner.nextLine(); return Line; } Line = scanner.nextLine(); System.out.println(lineCounter); lineCounter++; } return ""; } catch(Exception ex){ return ""; } } public void writeLine(String fileName, String line) throws IOException{ String Directory = "T:\\Documents\\NetBeansProjects\\MovieRatingSystem\\src\\main\\resources\\com\\mycompany\\movieratingsystem\\"; System.out.println(Directory + fileName); File file = new File(Directory + fileName); FileWriter fwrite = new FileWriter(file); fwrite.write(line); } } This is the movie and rating class that I am unclear about: import java.io.IOException; import javafx.event.ActionEvent; import javafx.fxml.FXML; import javafx.scene.control.TableView; import javafx.scene.layout.GridPane; public class SecondaryController { @FXML private TableView grdRatings; private void switchToPrimary() throws IOException { App.setRoot("primary"); } @FXML private void LoadData(ActionEvent event) { } }
Any help is appreciated, I was able to complete the the first part about login but I am not sure how to load movies and ratings into table view Using GUI and Javafx
Instructions:
1) Complete the login to read from the file and match with the correct username and password
2) Switch to the secondary screen
3) Load the Movies and the ratings associated with them to Table in the GUI
This is what I have
Log in class:
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.ArrayList;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.PasswordField;
import javafx.scene.control.TextField;
public class PrimaryController {
public static int counter = 0;
@FXML
private TextField txtUserName;
@FXML
private Button btnLogin;
@FXML
private Label lblErrorMsg;
@FXML
private PasswordField txtPassword;
private void switchToSecondary() throws IOException {
App.setRoot("secondary");
}
@FXML
private void btnLogin_click(ActionEvent event) throws FileNotFoundException, IOException {
try{
System.out.println(txtUserName.getText());
System.out.println(txtPassword.getText());
Utilities uti = new Utilities();
String line = uti.readLine("logininfo.txt", counter);
String[] userInfo = line.split(",");
System.out.println("User Name: " +userInfo[0]);
System.out.println("User Password: " +userInfo[1]);
//uti.writeLine("logininfo.txt", "sample,test2");
System.out.println(line);
if(counter %2 == 0)
lblErrorMsg.setText("Thank you for clicking on Login button");
else
lblErrorMsg.setText("");
while(line != ""){
System.out.println("User Name: " +userInfo[0]);
System.out.println("User Password: " +userInfo[1]);
userInfo = line.split(",");
if ((txtUserName.getText().equals(userInfo[0])) && (txtPassword.getText().equals(userInfo[1]))){
App.setRoot("secondary");
}
counter++;
line = uti.readLine("logininfo.txt", counter);
System.out.println(counter);
}
}
catch(Exception ex){
System.out.println(ex.getMessage());
}
}
}
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
/**
*
* @author itlabs
*/
public class Utilities {
public String readLine(String fileName, int lineNumber) throws FileNotFoundException{
String Directory = "T:\\Documents\\NetBeansProjects\\MovieRatingSystem\\src\\main\\resources\\com\\mycompany\\movieratingsystem\\";
System.out.println(Directory + fileName);
try{
File file = new File(Directory+ fileName);
Scanner scanner = new Scanner(file);
int lineCounter = 0;
String Line = "";
while(scanner.hasNextLine()){
if(lineCounter == lineNumber){
Line = scanner.nextLine();
return Line;
}
Line = scanner.nextLine();
System.out.println(lineCounter);
lineCounter++;
}
return "";
}
catch(Exception ex){
return "";
}
}
public void writeLine(String fileName, String line) throws IOException{
String Directory = "T:\\Documents\\NetBeansProjects\\MovieRatingSystem\\src\\main\\resources\\com\\mycompany\\movieratingsystem\\";
System.out.println(Directory + fileName);
File file = new File(Directory + fileName);
FileWriter fwrite = new FileWriter(file);
fwrite.write(line);
}
}
This is the movie and rating class that I am unclear about:
import java.io.IOException;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.TableView;
import javafx.scene.layout.GridPane;
public class SecondaryController {
@FXML
private TableView<?> grdRatings;
private void switchToPrimary() throws IOException {
App.setRoot("primary");
}
@FXML
private void LoadData(ActionEvent event) {
}
}

Step by step
Solved in 3 steps

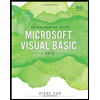
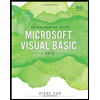