Ask the user for an account balance. Show, in descending order, all the accounts that have a balance greater than what the user input. Each entry is int, string, long, double, boolean (name length, name, credit card number, balance, cashback). i need the display produced from the code to be in descending order, it is displaying the opposite it also needs to produce yes or no instead of boolean true/ false this is my current code: import java.io.*; import java.util.*; public class AccountBalance { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); System.out.println("Enter a balance"); double input = keyboard.nextDouble(); // Create an ArrayList to store Account objects ArrayList accounts = new ArrayList<>(); try { // Open the binary file for reading DataInputStream inputstream = new DataInputStream(new FileInputStream("accounts-with-names.dat")); // Read the data from the file and store it in the ArrayList while (inputstream.available() > 0) { // Read name length from the file int nameLength = inputstream.readInt(); // Initialize char array to store name characters char[] nameChars = new char[nameLength]; // Read name characters from the file for (int i = 0; i < nameLength; i++) { nameChars[i] = inputstream.readChar(); } // Convert char array to String String name = new String(nameChars); // Read card number, balance, and cashback from the file long cardNumber = inputstream.readLong(); double balance = inputstream.readDouble(); boolean cashback = inputstream.readBoolean(); // Create a new Account object and add it to the ArrayList accounts.add(new Account(name, cardNumber, balance, cashback)); } // Sort the accounts by balance Collections.sort(accounts); // Print out the accounts with balance greater than or equal to input amount System.out.printf("Accounts with a balance of at least $%.2f (sorted by balance)%n", input); System.out.printf("%20s%20s%10s%12s%n", "Name", "Account Number", "Balance", "Cash Back?"); int count = 0; for (Account acc : accounts) { if (acc.getBalance() >= input) { System.out.printf("%20s%20d%10.2f%12s%n", acc.getName(), acc.getCardNumber(), acc.getBalance(), acc.hasCashback()); count++; } } // Print out the number of results found System.out.printf("%d results%n", count); // Close the input stream inputstream.close(); } catch (IOException e) { e.printStackTrace(); } } } class Account implements Comparable { private String name; private long cardNumber; private double balance; private boolean cashback; public Account(String name, long cardNumber, double balance, boolean cashback) { this.name = name; this.cardNumber = cardNumber; this.balance = balance; this.cashback = cashback; } public String getName() { return name; } public long getCardNumber() { return cardNumber; } public double getBalance() { return balance; } public boolean hasCashback() { return cashback; } @Override public int compareTo(Account other) { return Double.compare(this.balance, other.balance); } } this is the outcome it produces Enter a balance\n 9000ENTER Accounts with a balance of at least $9000.00 (sorted by balance)\n Name Account Number Balance Cash Back\n Jordan Rylstone 201715141501700 9135.90 true\n Tiphanie Oland 5100172198301454 9315.15 false\n Reube Worsnop 3551244602153760 9409.97 true\n Eachelle Balderstone 30526110612015 9866.30 false\n Stanislaw Dhenin 4405942746261912 9869.27 false\n Paco Verty 4508271490627227 9890.51 false\n Brand Hallam 3573877643495486 9985.21 false\n 7 results\n this is what i need it to display Enter a balance\n 9000ENTER Accounts with a balance of at least $9000.00 (sorted by balance)\n Name Account Number Balance Cash Back\n Brand Hallam 3573877643495486 9985.21 No\n Paco Verty 4508271490627227 9890.51 No\n Stanislaw Dhenin 4405942746261912 9869.27 No\n Eachelle Balderstone 30526110612015 9866.30 No\n Reube Worsnop 3551244602153760 9409.97 Yes\n Tiphanie Oland 5100172198301454 9315.15 No\n Jordan Rylstone 201715141501700 9135.90 Yes\n 7 results\n can a java expert help with these two changes
Ask the user for an account balance. Show, in descending order, all the accounts that have a balance greater than what the user input.
i need the display produced from the code to be in descending order, it is displaying the opposite
it also needs to produce yes or no instead of boolean true/ false
this is my current code:
import java.io.*;
import java.util.*;
public class AccountBalance {
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
System.out.println("Enter a balance");
double input = keyboard.nextDouble();
// Create an ArrayList to store Account objects
ArrayList<Account> accounts = new ArrayList<>();
try {
// Open the binary file for reading
DataInputStream inputstream = new DataInputStream(new FileInputStream("accounts-with-names.dat"));
// Read the data from the file and store it in the ArrayList
while (inputstream.available() > 0) {
// Read name length from the file
int nameLength = inputstream.readInt();
// Initialize char array to store name characters
char[] nameChars = new char[nameLength];
// Read name characters from the file
for (int i = 0; i < nameLength; i++) {
nameChars[i] = inputstream.readChar();
}
// Convert char array to String
String name = new String(nameChars);
// Read card number, balance, and cashback from the file
long cardNumber = inputstream.readLong();
double balance = inputstream.readDouble();
boolean cashback = inputstream.readBoolean();
// Create a new Account object and add it to the ArrayList
accounts.add(new Account(name, cardNumber, balance, cashback));
}
// Sort the accounts by balance
Collections.sort(accounts);
// Print out the accounts with balance greater than or equal to input amount
System.out.printf("Accounts with a balance of at least $%.2f (sorted by balance)%n", input);
System.out.printf("%20s%20s%10s%12s%n", "Name", "Account Number", "Balance", "Cash Back?");
int count = 0;
for (Account acc : accounts) {
if (acc.getBalance() >= input) {
System.out.printf("%20s%20d%10.2f%12s%n", acc.getName(), acc.getCardNumber(),
acc.getBalance(), acc.hasCashback());
count++;
}
}
// Print out the number of results found
System.out.printf("%d results%n", count);
// Close the input stream
inputstream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class Account implements Comparable<Account> {
private String name;
private long cardNumber;
private double balance;
private boolean cashback;
public Account(String name, long cardNumber, double balance, boolean cashback) {
this.name = name;
this.cardNumber = cardNumber;
this.balance = balance;
this.cashback = cashback;
}
public String getName() {
return name;
}
public long getCardNumber() {
return cardNumber;
}
public double getBalance() {
return balance;
}
public boolean hasCashback() {
return cashback;
}
@Override
public int compareTo(Account other) {
return Double.compare(this.balance, other.balance);
}
}
this is the outcome it produces
9000ENTER
Accounts with a balance of at least $9000.00 (sorted by balance)\n
Name Account Number Balance Cash Back\n
Jordan Rylstone 201715141501700 9135.90 true\n
Tiphanie Oland 5100172198301454 9315.15 false\n
Reube Worsnop 3551244602153760 9409.97 true\n
Eachelle Balderstone 30526110612015 9866.30 false\n
Stanislaw Dhenin 4405942746261912 9869.27 false\n
Paco Verty 4508271490627227 9890.51 false\n
Brand Hallam 3573877643495486 9985.21 false\n
7 results\n
this is what i need it to display
9000ENTER
Accounts with a balance of at least $9000.00 (sorted by balance)\n
Name Account Number Balance Cash Back\n
Brand Hallam 3573877643495486 9985.21 No\n
Paco Verty 4508271490627227 9890.51 No\n
Stanislaw Dhenin 4405942746261912 9869.27 No\n
Eachelle Balderstone 30526110612015 9866.30 No\n
Reube Worsnop 3551244602153760 9409.97 Yes\n
Tiphanie Oland 5100172198301454 9315.15 No\n
Jordan Rylstone 201715141501700 9135.90 Yes\n
7 results\n
can a java expert help with these two changes

Here, we have a working JAVA code.
We need to solve this issue: display produced from the code to be in descending order, it is displaying the opposite
I have provided updated JAVA code with explanation and output.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

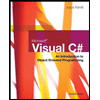
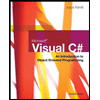