Assume vector VDB represents a student database. The structure of the Student records is given below. struct Student ( int id; // student id //global GPA double gpa; string major; // values such as MATH, MAGIC, CSCI, etc. } Students who have more than one major have multiple entries in the vector. For instance, assume student 1234 is concentrating in MATH and MAGIC. Therefore, the records [1234, 3.90, "MAGIC"] and [1234, 3.90, "MATH") will appear in vDB. PAD noints) Write a method to find the students enrolled in a given major. The function's prototype is void selectByMajor (vector& VDB, vector& vSolution, string majorValue); where vSolution is the vector holding the selected students whose major matches majorValue. points) Write a function to create a vector holding only students who appear in the first list (v1) but not the second (v2). For example, assume vector v1 and v2 hold MAGIC and MATH majors respectively. The function will produce a solution vector including students who exclusively study MATH and do not concentrate in MAGIC. The prototype follows. vector selectMinus (vector& v1, vector v2); The following is a sample of the app's operation and is provided for you to visualize the nature of the data and the app. Please, DO NOT WRITE anything. Your functions are not responsible for printing anything, they must not include any "cout <<" statements.
Assume vector VDB represents a student database. The structure of the Student records is given below. struct Student ( int id; // student id //global GPA double gpa; string major; // values such as MATH, MAGIC, CSCI, etc. } Students who have more than one major have multiple entries in the vector. For instance, assume student 1234 is concentrating in MATH and MAGIC. Therefore, the records [1234, 3.90, "MAGIC"] and [1234, 3.90, "MATH") will appear in vDB. PAD noints) Write a method to find the students enrolled in a given major. The function's prototype is void selectByMajor (vector& VDB, vector& vSolution, string majorValue); where vSolution is the vector holding the selected students whose major matches majorValue. points) Write a function to create a vector holding only students who appear in the first list (v1) but not the second (v2). For example, assume vector v1 and v2 hold MAGIC and MATH majors respectively. The function will produce a solution vector including students who exclusively study MATH and do not concentrate in MAGIC. The prototype follows. vector selectMinus (vector& v1, vector v2); The following is a sample of the app's operation and is provided for you to visualize the nature of the data and the app. Please, DO NOT WRITE anything. Your functions are not responsible for printing anything, they must not include any "cout <<" statements.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
please answer in c++
![Assume vector<Student> VDB represents a student database. The structure of the Student records is given below.
struct Student
(
int
id;
// student id
double gpa;
//global GPA
string major;
// values such as MATH, MAGIC, CSCI, etc.
}
Students who have more than one major have multiple entries in the vector. For instance, assume student 1234 is
concentrating in MATH and MAGIC. Therefore, the records [1234, 3.90, "MAGIC"] and [1234, 3.90, "MATH") will appear
in vDB.
PADE
points) Write a method to find the students enrolled in a given major. The function's prototype is
void selectByMajor (vector<Student>& VDB, vector<Student>& vSolution, string majorValue);
where vSolution is the vector holding the selected students whose major matches majorValue.
points) Write a function to create a vector holding only students who appear in the first list (v1) but not the
second (v2). For example, assume vector v1 and v2 hold MAGIC and MATH majors respectively. The function will produce a
solution vector including students who exclusively study MATH and do not concentrate in MAGIC. The prototype follows.
vector<Student> selectMinus (vector<Student>& v1, vector<Student> v2);
The following is a sample of the app's operation and is provided for you to visualize the nature of the data and the app. Please,
DO NOT WRITE anything. Your functions are not responsible for printing anything, they must not include any "cout <<"
statements.
MAGIC majors
Student [ ID: 100, GPA: 3.1, Major: MAGIC ]
Student [ ID: 300, GPA: 3.3, Major: MAGIC ]
Student [ ID: 400, GPA: 3.4, Major: MAGIC ]
Student ID: 600, GPA: 3.6, Major: MAGIC ]
Student [ ID: 700, GPA: 3.7, Major: MAGIC ]
Student [ ID: 800, GPA: 3.8, Major: MAGIC ]
Student [ ID: 900, GPA: 3.9, Major: MAGIC ]
MATH majors
Student [ ID: 200, GPA: 3.2, Major: MATH ]
Student [ ID: 400, GPA: 3.4, Major: MATH]
Student [ ID: 500, GPA: 3.5, Major: MATH ]
Student [ ID: 700, GPA: 3.7, Major: MATH ]
MAGIC Minus MATH
Student [ ID: 100, GPA: 3.1, Major: MAGIC ]
Student [ ID: 300, GPA: 3.3, Major: MAGIC ]
Student [ID: 600, GPA: 3.6, Major: MAGIC ]
Student [ ID: 800, GPA: 3.8, Major: MAGIC ]
Student [ ID: 900, GPA: 3.9, Major: MAGIC ]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffe2257a7-ec8b-4cb2-8972-c13e17aa7768%2F83d91c20-88c0-432f-9e91-95ade165c3f1%2Fcr1qbd_processed.png&w=3840&q=75)
Transcribed Image Text:Assume vector<Student> VDB represents a student database. The structure of the Student records is given below.
struct Student
(
int
id;
// student id
double gpa;
//global GPA
string major;
// values such as MATH, MAGIC, CSCI, etc.
}
Students who have more than one major have multiple entries in the vector. For instance, assume student 1234 is
concentrating in MATH and MAGIC. Therefore, the records [1234, 3.90, "MAGIC"] and [1234, 3.90, "MATH") will appear
in vDB.
PADE
points) Write a method to find the students enrolled in a given major. The function's prototype is
void selectByMajor (vector<Student>& VDB, vector<Student>& vSolution, string majorValue);
where vSolution is the vector holding the selected students whose major matches majorValue.
points) Write a function to create a vector holding only students who appear in the first list (v1) but not the
second (v2). For example, assume vector v1 and v2 hold MAGIC and MATH majors respectively. The function will produce a
solution vector including students who exclusively study MATH and do not concentrate in MAGIC. The prototype follows.
vector<Student> selectMinus (vector<Student>& v1, vector<Student> v2);
The following is a sample of the app's operation and is provided for you to visualize the nature of the data and the app. Please,
DO NOT WRITE anything. Your functions are not responsible for printing anything, they must not include any "cout <<"
statements.
MAGIC majors
Student [ ID: 100, GPA: 3.1, Major: MAGIC ]
Student [ ID: 300, GPA: 3.3, Major: MAGIC ]
Student [ ID: 400, GPA: 3.4, Major: MAGIC ]
Student ID: 600, GPA: 3.6, Major: MAGIC ]
Student [ ID: 700, GPA: 3.7, Major: MAGIC ]
Student [ ID: 800, GPA: 3.8, Major: MAGIC ]
Student [ ID: 900, GPA: 3.9, Major: MAGIC ]
MATH majors
Student [ ID: 200, GPA: 3.2, Major: MATH ]
Student [ ID: 400, GPA: 3.4, Major: MATH]
Student [ ID: 500, GPA: 3.5, Major: MATH ]
Student [ ID: 700, GPA: 3.7, Major: MATH ]
MAGIC Minus MATH
Student [ ID: 100, GPA: 3.1, Major: MAGIC ]
Student [ ID: 300, GPA: 3.3, Major: MAGIC ]
Student [ID: 600, GPA: 3.6, Major: MAGIC ]
Student [ ID: 800, GPA: 3.8, Major: MAGIC ]
Student [ ID: 900, GPA: 3.9, Major: MAGIC ]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Recommended textbooks for you
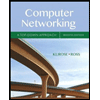
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
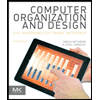
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
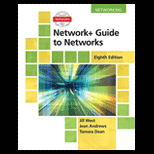
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
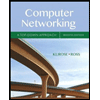
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
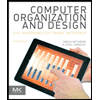
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
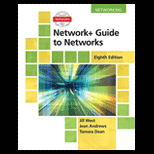
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
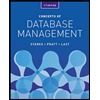
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
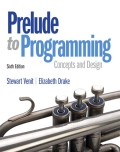
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
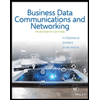
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY