C++ Programming: Instructions The program in Example 5-6 implements the Number Guessing Game. However, in that program, the user is given as many tries as needed to guess the correct number. Rewrite the program so that the user has no more than five tries to guess the correct number. Your program should print an appropriate message, such as “You win!” or “You lose!”. Code Given: //Flag-controlled while loop. //Number guessing game. #include #include #include using namespace std; int main() { int num; //variable to store the random number int guess; //variable to store the number guessed by the user bool isGuessed; //boolean variable to control the loop srand(time(0)); num = rand() % 100; isGuessed = false; while (!isGuessed) { cout << "Enter an integer greater" <<" than or equal to 0 and " <<"less than 100: "; cin >> guess; cout << endl; if (guess == num) { cout << "You win! " << endl; isGuessed = true; } elseif (guess < num) cout << "Your guess is lower than the " <<"number.\n Guess again!" << endl; elseif (guess > num) cout << "Your guess is higher than " <<"the number.\n Guess again!" << endl; elseif (guess != num) cout << "Your guess is wrong. " <<"Guess again!" << endl; elseif (guess != num) cout << "Your guess is wrong. " <<"Guess again!" << endl; elseif (guess != num) cout << "Your guess is wrong. " <<"Guess again!" << endl; else (guess != num); cout << "You lose!"; } //end while return0; }
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
C++
Instructions
The program in Example 5-6 implements the Number Guessing Game. However, in that program, the user is given as many tries as needed to guess the correct number.
Rewrite the program so that the user has no more than five tries to guess the correct number.
Your program should print an appropriate message, such as “You win!” or “You lose!”.
Code Given:

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

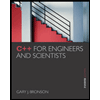
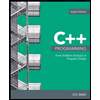
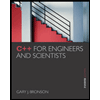
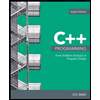