C++ Write a program that takes as input an arithmetic expression followed by a semicolon ;. The program outputs whether the expression contains matching grouping symbols. For example, the arithmetic expressions {25 + (3 – 6) * 8} and 7 + 8 * 2 contains matching grouping symbols. However, the expression 5 + {(13 + 7) / 8 - 2 * 9 does not contain matching grouping symbols. If the expression contains matching grouping symbols, the program output should contain the following text: Expression has matching grouping symbol If the expression does not contain matching grouping symbols, the program output should contain the following text: Expression does not have matching grouping symbols
C++
Write a program that takes as input an arithmetic expression followed by a semicolon ;.
The program outputs whether the expression contains matching grouping symbols.
For example, the arithmetic expressions {25 + (3 – 6) * 8} and 7 + 8 * 2 contains matching grouping symbols.
However, the expression 5 + {(13 + 7) / 8 - 2 * 9 does not contain matching grouping symbols.
If the expression contains matching grouping symbols, the program output should contain the following text:
Expression has matching grouping symbol
If the expression does not contain matching grouping symbols, the program output should contain the following text:
Expression does not have matching grouping symbols.


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

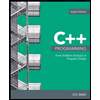
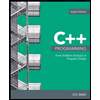