1- Create a hierarchy of Java classes as follows: MyRectangle is_a MyShape; MyOval is a MyShape. Class MyPoint: Class MyPoint is used by class MyShape to define the reference point, p(x, y), of the Java display coordinate system, as well as by all subclasses in the class hierarchy to define the points stipulated in the subclass definition. The class utilizes a color of enum reference type MyColor, and includes appropriate class constructors and methods. The class also includes draw and toString methods, as well as methods that perform point related operations, including but not limited to, shift of point position, distance to the origin and to another point, angle [in degrees] with the x-axis of the line extending from this point to another point. Enum MyColor: Enum MyColor is used by class MyShape and all subclasses in the class hierarchy to define the colors of the shapes. The enum reference type defines a set of constant colors by their red, green, blue, and opacity, components, with values in the range [o - 255]. The enum reference type includes a constructor and appropriate methods, including methods that return the corresponding the word-packed and hexadecimal representations and JavaFx Color objects of the constant colors in MyColor. Class MyShape: Class MyShape is the, non-abstract, superclass of the hierarchy, extending the Java class Object. An implementation of the class defines a reference point, p(x, y), of type MyPoint, and the color of the shape of enum reference type MyColor. The class includes appropriate class constructors and methods, including methods, including methods that perform the following operations: a. b. C. a. b. Class MyRectangle: Class MyRectangle extends class MyShape. The MyRectangle object is a rectangle of height hand width w, and a top left corner point p(x, y), and may be filled with a color of enum reference type MyColor. The class includes appropriate class constructors and methods, including methods that perform the following operations: C. a. b. C. Class MyOval: Class MyOval extends class MyShape. The MyOval object is defined by an ellipse within a rectangle of height h and width w, and a center point p(x, y). The MyOval object may be filled with a color of enum reference type MyColor. The class includes appropriate class constructors and methods, including methods that perform the following operations: له area, perimeter – return the area and perimeter of the object. These methods must be overridden in each subclass in the hierarchy. For the MyShape object, the methods return 2- zero. toString - returns the object's description as a String. This method must be overridden in each subclass in the hierarchy; 3- draw - draws the object shape. This method must be overridden in each subclass in the hierarchy. For the MyShape object, it paints the drawing canvas in the color specified. getP, getWidth, getHeight return the top left corner point, width, and height of the MyRectangle object toString returns a string representation of the MyRectangle object: top left corner point, width, height, perimeter, and area; draw-draws a MyRectangle object. getX, getY, getA, getB — return the x- and y-coordinates of the center point and abscissa of the MyOval object; toString-returns a string representation of the MyOval object: axes lengths, perimeter, and area; draw-draws a MyOval object. Use JavaFX_graphics and the class hierarchy to draw the geometric configuration comprised of a sequence of alternating concentric circles and their inscribed rectangles, as illustrated below, subject to the following additional requirements: a. The code is applicable to canvases of variable height and width; b. The dimensions of the shapes are proportional to the smallest dimension of the canvas; c. The circles and rectangles are filled with different colors of your choice, specified through an enum reference type MyColor. Explicitly specify all the classes imported and used in your code.
1- Create a hierarchy of Java classes as follows: MyRectangle is_a MyShape; MyOval is a MyShape. Class MyPoint: Class MyPoint is used by class MyShape to define the reference point, p(x, y), of the Java display coordinate system, as well as by all subclasses in the class hierarchy to define the points stipulated in the subclass definition. The class utilizes a color of enum reference type MyColor, and includes appropriate class constructors and methods. The class also includes draw and toString methods, as well as methods that perform point related operations, including but not limited to, shift of point position, distance to the origin and to another point, angle [in degrees] with the x-axis of the line extending from this point to another point. Enum MyColor: Enum MyColor is used by class MyShape and all subclasses in the class hierarchy to define the colors of the shapes. The enum reference type defines a set of constant colors by their red, green, blue, and opacity, components, with values in the range [o - 255]. The enum reference type includes a constructor and appropriate methods, including methods that return the corresponding the word-packed and hexadecimal representations and JavaFx Color objects of the constant colors in MyColor. Class MyShape: Class MyShape is the, non-abstract, superclass of the hierarchy, extending the Java class Object. An implementation of the class defines a reference point, p(x, y), of type MyPoint, and the color of the shape of enum reference type MyColor. The class includes appropriate class constructors and methods, including methods, including methods that perform the following operations: a. b. C. a. b. Class MyRectangle: Class MyRectangle extends class MyShape. The MyRectangle object is a rectangle of height hand width w, and a top left corner point p(x, y), and may be filled with a color of enum reference type MyColor. The class includes appropriate class constructors and methods, including methods that perform the following operations: C. a. b. C. Class MyOval: Class MyOval extends class MyShape. The MyOval object is defined by an ellipse within a rectangle of height h and width w, and a center point p(x, y). The MyOval object may be filled with a color of enum reference type MyColor. The class includes appropriate class constructors and methods, including methods that perform the following operations: له area, perimeter – return the area and perimeter of the object. These methods must be overridden in each subclass in the hierarchy. For the MyShape object, the methods return 2- zero. toString - returns the object's description as a String. This method must be overridden in each subclass in the hierarchy; 3- draw - draws the object shape. This method must be overridden in each subclass in the hierarchy. For the MyShape object, it paints the drawing canvas in the color specified. getP, getWidth, getHeight return the top left corner point, width, and height of the MyRectangle object toString returns a string representation of the MyRectangle object: top left corner point, width, height, perimeter, and area; draw-draws a MyRectangle object. getX, getY, getA, getB — return the x- and y-coordinates of the center point and abscissa of the MyOval object; toString-returns a string representation of the MyOval object: axes lengths, perimeter, and area; draw-draws a MyOval object. Use JavaFX_graphics and the class hierarchy to draw the geometric configuration comprised of a sequence of alternating concentric circles and their inscribed rectangles, as illustrated below, subject to the following additional requirements: a. The code is applicable to canvases of variable height and width; b. The dimensions of the shapes are proportional to the smallest dimension of the canvas; c. The circles and rectangles are filled with different colors of your choice, specified through an enum reference type MyColor. Explicitly specify all the classes imported and used in your code.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 3PE
Related questions
Question
![1-
Create a hierarchy of Java classes as follows:
MyRectangle is_a MyShape;
MyOval is a MyShape.
Class MyPoint:
Class MyPoint is used by class MyShape to define the reference point, p(x, y), of the Java
display coordinate system, as well as by all subclasses in the class hierarchy to define the points
stipulated in the subclass definition. The class utilizes a color of enum reference type MyColor,
and includes appropriate class constructors and methods. The class also includes draw and
toString methods, as well as methods that perform point related operations, including but not
limited to, shift of point position, distance to the origin and to another point, angle [in degrees]
with the x-axis of the line extending from this point to another point.
Enum MyColor:
Enum MyColor is used by class MyShape and all subclasses in the class hierarchy to define
the colors of the shapes. The enum reference type defines a set of constant colors by their red,
green, blue, and opacity, components, with values in the range [o - 255]. The enum reference
type includes a constructor and appropriate methods, including methods that return the
corresponding the word-packed and hexadecimal representations and JavaFx Color objects of
the constant colors in MyColor.
Class MyShape:
Class MyShape is the, non-abstract, superclass of the hierarchy, extending the Java class
Object. An implementation of the class defines a reference point, p(x, y), of type MyPoint, and
the color of the shape of enum reference type MyColor. The class includes appropriate class
constructors and methods, including methods, including methods that perform the following
operations:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Feb62ec4b-b625-4332-acd2-c86081f32c3c%2F9bc5a278-73c9-4690-8197-7b4aa4bf8903%2Fq4gx6c5_processed.png&w=3840&q=75)
Transcribed Image Text:1-
Create a hierarchy of Java classes as follows:
MyRectangle is_a MyShape;
MyOval is a MyShape.
Class MyPoint:
Class MyPoint is used by class MyShape to define the reference point, p(x, y), of the Java
display coordinate system, as well as by all subclasses in the class hierarchy to define the points
stipulated in the subclass definition. The class utilizes a color of enum reference type MyColor,
and includes appropriate class constructors and methods. The class also includes draw and
toString methods, as well as methods that perform point related operations, including but not
limited to, shift of point position, distance to the origin and to another point, angle [in degrees]
with the x-axis of the line extending from this point to another point.
Enum MyColor:
Enum MyColor is used by class MyShape and all subclasses in the class hierarchy to define
the colors of the shapes. The enum reference type defines a set of constant colors by their red,
green, blue, and opacity, components, with values in the range [o - 255]. The enum reference
type includes a constructor and appropriate methods, including methods that return the
corresponding the word-packed and hexadecimal representations and JavaFx Color objects of
the constant colors in MyColor.
Class MyShape:
Class MyShape is the, non-abstract, superclass of the hierarchy, extending the Java class
Object. An implementation of the class defines a reference point, p(x, y), of type MyPoint, and
the color of the shape of enum reference type MyColor. The class includes appropriate class
constructors and methods, including methods, including methods that perform the following
operations:

Transcribed Image Text:a.
b.
C.
a.
b.
Class MyRectangle:
Class MyRectangle extends class MyShape. The MyRectangle object is a rectangle of
height hand width w, and a top left corner point p(x, y), and may be filled with a color of enum
reference type MyColor. The class includes appropriate class constructors and methods,
including methods that perform the following operations:
C.
a.
b.
C.
Class MyOval:
Class MyOval extends class MyShape. The MyOval object is defined by an ellipse within a
rectangle of height h and width w, and a center point p(x, y). The MyOval object may be filled
with a color of enum reference type MyColor. The class includes appropriate class constructors
and methods, including methods that perform the following operations:
له
area, perimeter – return the area and perimeter of the object. These methods must be
overridden in each subclass in the hierarchy. For the MyShape object, the methods return
2-
zero.
toString - returns the object's description as a String. This method must be overridden in
each subclass in the hierarchy;
3-
draw - draws the object shape. This method must be overridden in each subclass in the
hierarchy. For the MyShape object, it paints the drawing canvas in the color specified.
getP, getWidth, getHeight return the top left corner point, width, and height of the
MyRectangle object
toString returns a string representation of the MyRectangle object: top left corner
point, width, height, perimeter, and area;
draw-draws a MyRectangle object.
getX, getY, getA, getB — return the x- and y-coordinates of the center point and abscissa of
the MyOval object;
toString-returns a string representation of the MyOval object: axes lengths, perimeter,
and area;
draw-draws a MyOval object.
Use JavaFX_graphics and the class hierarchy to draw the geometric configuration
comprised of a sequence of alternating concentric circles and their inscribed rectangles, as
illustrated below, subject to the following additional requirements:
a. The code is applicable to canvases of variable height and width;
b. The dimensions of the shapes are proportional to the smallest dimension of the canvas;
c. The circles and rectangles are filled with different colors of your choice, specified
through an enum reference type MyColor.
Explicitly specify all the classes imported and used in your code.
Expert Solution

Trending now
This is a popular solution!
Step by step
Solved in 10 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
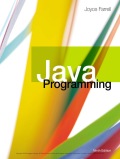
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
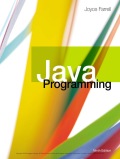
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT