Create a base class called Insect. All insects have a color and a size. The size is measured in millimeters (use a double data type). Provide a default constructor that initializes the size to zero and outputs the message “Invoking the default Insect constructor” and another constructor that allows the color and size to be set by the client. This other constructor should also output the message “Invoking the 2-argument Insect constructor.” Also create a destructor for this class that outputs the message “Invoking the default Insect destructor.” Your Insect class should have a function called Eat that cannot be implemented. That is, it should be declared as a purely virtual function. Your class should also have Get and Set methods to allow the color and size to be accessed. From the Insect class, derive Ant, Grasshopper, Beetle, and Termite classes. The derived classes should each have constructors and destructors that output an appropriate message (e.g., the Ant constructor outputs “Invoking Ant constructor,” and the Ant destructor outputs “Invoking Ant destructor”). The constructor of each derived class should allow the color and size of the Insect to be set (think member initialization list). The derived classes should each have a member function called Eat that overrides the Insect Eat version. Ant Eat should output “As an ant, I eat everything,” Grasshopper Eat should output “As a grasshopper, I eat grass and leaves,” Beetle Eat should output “As a beetle, I eat plants and other small insects,” and Termite Eat should output “As a termite, I eat wood.” Write a main function that uses the Insect and derived classes as needed to do the following. You must perform the actions below in the sequence described (i.e., do not take a shortcut around using dynamic memory allocation/deallocation and virtual methods since they are the whole point of the lab). a.Use the uniform_real_distribution class (described in section 13-6 of your textbook) to generate a random size in millimeters based on the insect selected. The range of sizes for each insect are as follows: Ant: .01 to 1.0 millimeters Grasshopper: 10.5 to 50.0 millimeters Beetle: 40.0 to 75.5 millimeters Termite: 1.5 to 5.5 millimeters b.Prompt the user to make an Insect selection [e.g. (1) for Ant, (2) for Grasshopper, (3) for Beetle, and (4) for Termite] and to enter a color for the insect. Dynamically create an Ant, Grasshopper, Beetle, or Termite object (depending on what the user entered) and initialize it with a constructor to which is passed its color and size. Save the object (use an array called “insects” – credit will not be awarded if you use a vector for this). You should hardcode the size of the array to hold 5 elements. That is, you don’t need to dynamically create this array unless you want to. If you do dynamically allocate it, though, be sure to deallocate its memory properly. c.Repeat steps a. and b. 4 more You do not know what insects the user will select or in what order, so you must figure out how to create and store the appropriate objects. Notice that there are 4 insects from which to choose, but the user will make 5 selections. Therefore, one insect could be chosen twice. d.After the user has entered all 5 selections, execute another loop that cycles through the 5 selections and invokes the Eat function and also displays the color and size of the insect. If you have done it properly, each of your outputs will correspond to the type of Insect the user selected in the order he or she entered them. Your input screen should look like the pictures below:
- Create a base class called Insect. All insects have a color and a size. The size is measured in millimeters (use a double data type). Provide a default constructor that initializes the size to zero and outputs the message “Invoking the default Insect constructor” and another constructor that allows the color and size to be set by the client. This other constructor should also output the message “Invoking the 2-argument Insect constructor.” Also create a destructor for this class that outputs the message “Invoking the default Insect destructor.” Your Insect class should have a function called Eat that cannot be implemented. That is, it should be declared as a purely virtual function. Your class should also have Get and Set methods to allow the color and size to be accessed.
- From the Insect class, derive Ant, Grasshopper, Beetle, and Termite classes. The derived classes should each have constructors and destructors that output an appropriate message (e.g., the Ant constructor outputs “Invoking Ant constructor,” and the Ant destructor outputs “Invoking Ant destructor”). The constructor of each derived class should allow the color and size of the Insect to be set (think member initialization list). The derived classes should each have a member function called Eat that overrides the Insect Eat version. Ant Eat should output “As an ant, I eat everything,” Grasshopper Eat should output “As a grasshopper, I eat grass and leaves,” Beetle Eat should output “As a beetle, I eat plants and other small insects,” and Termite Eat should output “As a termite, I eat wood.”
- Write a main function that uses the Insect and derived classes as needed to do the following. You must perform the actions below in the sequence described (i.e., do not take a shortcut around using dynamic memory allocation/deallocation and virtual methods since they are the whole point of the lab).
a.Use the uniform_real_distribution class (described in section 13-6 of your textbook) to generate a random size in millimeters based on the insect selected.
The range of sizes for each insect are as follows:
Ant: .01 to 1.0 millimeters
Grasshopper: 10.5 to 50.0 millimeters
Beetle: 40.0 to 75.5 millimeters
Termite: 1.5 to 5.5 millimeters
b.Prompt the user to make an Insect selection [e.g. (1) for Ant, (2) for Grasshopper, (3) for Beetle, and (4) for Termite] and to enter a color for the insect. Dynamically create an Ant, Grasshopper, Beetle, or Termite object (depending on what the user entered) and initialize it with a constructor to which is passed its color and size. Save the object (use an array called “insects” – credit will not be awarded if you use a
c.Repeat steps a. and b. 4 more You do not know what insects the user will select or in what order, so you must figure out how to create and store the appropriate objects. Notice that there are 4 insects from which to choose, but the user will make 5 selections. Therefore, one insect could be chosen twice.
d.After the user has entered all 5 selections, execute another loop that cycles through the 5 selections and invokes the Eat function and also displays the color and size of the insect. If you have done it properly, each of your outputs will correspond to the type of Insect the user selected in the order he or she entered them.
Your input screen should look like the pictures below:


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

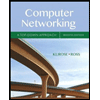
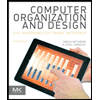
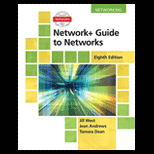
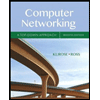
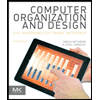
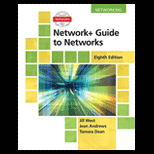
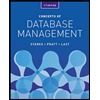
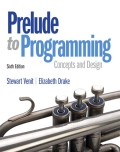
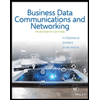