Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string - title : string - id : int - count : static int + Book() + Book(string, string) + setAuthor(string) : void + setTitle(string) : void + print() : void + setID() : void
In C++
- Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf.
- Here are their UML diagrams
Book class UML
Book |
- author : string - title : string - id : int - count : static int |
+ Book() + Book(string, string) + setAuthor(string) : void + setTitle(string) : void + print() : void + setID() : void |
And the Bookshelf class UML
Bookshelf |
- book1 : Book - book2 : Book - book3 : Book |
+ Bookshelf() + Bookshelf(Book, Book, Book) + setBook1(Book) : void + setBook2(Book) : void + setBook3(Book) : void + print() : void |
Some additional information:
-
-
The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters .
-
The Book class will have a static member variable named count. You will use this variable to assign each book a unique ID number. Refer to this week’s sample code (Dog and Collar) for help with this.
-
The Bookshelf class has two constructors. So, when you first create a Bookshelf object, you can choose which three books it will hold (by creating three Book objects, and using them during the Bookshelf declaration), or you can choose to have them set to default values (by using the Bookshelf default constructor).
-
You will also make use of the const keyword to indicate which methods are not modifying the object.
-
Demonstrate the use of your Bookshelf class by creating two objects of Bookshelf, each one using a different constructor. With the Bookshelf created using the default constructor, use the setters for each Book to set the Book objects to non default values.
-
Potential sample run:
Here are the books on the shelf:
Title: The Martian, Author: Andy Weir, ID: 1000
Title: Before the Coffee Gets Cold, Author: Toshikazu Kawaguchi, ID: 1001
Title: Cat's Cradle, Author: Kurt Vonnegut, ID: 1002
Here are the books on the shelf:
Title: Cannery Row, Author: John Steinbeck, ID: 1012
Title: City of Thieves, Author: David Benioff, ID: 1013
Title: The Shadow of the Wind, Author: Carlos Ruiz Zafon, ID: 1014
-
Note: Your sample run may have different ID numbers for the books. That’s okay, as long as each book has its own unique ID that is set automatically when the book is created.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

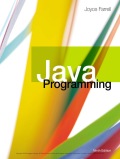
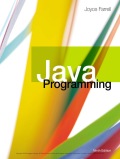