* Design a class ourVector that mimics the javaVector. The class has the following * properties: - A private variable size that keeps track of how many elements in the array - A reference to an array of integers - An increment by how much to increase wjen it's full - A default constructor that creates an array of 5 with increment 10; - A constructor that accepts the initial capacity and creates an array of that capacity and an increment of twice the capacity - A constructor that accepts two integers, the first for the capacity and the second for increment. - Capacity, the length of the array - int size(); A method that returns the size of the array - boolean isEmpty(); returns true if it's empty and false if not. - void addBack(int e); adds e to the back of the array - void addFront(int e); adds e to the front of the array. - Override String toString(); to return the content of the array in the form [-1, -4, -6] - int elementAt(int ind); returns the elements at index ind, if valid. - int removeBack(); removes the element in the back of the array and returns it (update size) - int removeFront(); removes the element in the front of the array and returns it (update size) - void add(int ind, int e); adds e to the index ind. - int remove(int ind); removes element at index ind and returns it. */ public class ourVector { //private variables private int size; //Let's use .length fro capacity no need for another variable private int increment; private int[] V; //V is a reference //constructors public ourVector() { V = new int[5]; size = 0; increment = 10; } public ourVector(int capacity) { V = new int[capacity]; size = 0; increment = 2*capacity; } public ourVector(int capacity, int incr) { V = new int[capacity]; size = 0; increment = incr; } //methods public int size() { return size; } public boolean isEmpty() { return (size == 0); //if size is 0 return true else return false } //WH TODO: Finish the resize method and test it private void resize() { //create a temporary array temp with capacity V.length+increment //copy all elements from V to temp //assign temp to V. return; } public int capacity() { return V.length; } public void addBack(int e) { //test first if it's not full if(size == V.length) { System.out.println("The vector is full resizing"); this.resize(); } //insert e at index size V[size] = e; size++; } public void add(int e) { this.addBack(e); } //HW TODO: fix toString so that the last comma does not appear //toString to return the array as a string in the form [-1, -5, -7] public String toString() { if(this.isEmpty()) { return "[]"; } String st = "["; for(int i =0; i
* Design a class ourVector that mimics the javaVector. The class has the following * properties: - A private variable size that keeps track of how many elements in the array - A reference to an array of integers - An increment by how much to increase wjen it's full - A default constructor that creates an array of 5 with increment 10; - A constructor that accepts the initial capacity and creates an array of that capacity and an increment of twice the capacity - A constructor that accepts two integers, the first for the capacity and the second for increment. - Capacity, the length of the array - int size(); A method that returns the size of the array - boolean isEmpty(); returns true if it's empty and false if not. - void addBack(int e); adds e to the back of the array - void addFront(int e); adds e to the front of the array. - Override String toString(); to return the content of the array in the form [-1, -4, -6] - int elementAt(int ind); returns the elements at index ind, if valid. - int removeBack(); removes the element in the back of the array and returns it (update size) - int removeFront(); removes the element in the front of the array and returns it (update size) - void add(int ind, int e); adds e to the index ind. - int remove(int ind); removes element at index ind and returns it. */ public class ourVector { //private variables private int size; //Let's use .length fro capacity no need for another variable private int increment; private int[] V; //V is a reference //constructors public ourVector() { V = new int[5]; size = 0; increment = 10; } public ourVector(int capacity) { V = new int[capacity]; size = 0; increment = 2*capacity; } public ourVector(int capacity, int incr) { V = new int[capacity]; size = 0; increment = incr; } //methods public int size() { return size; } public boolean isEmpty() { return (size == 0); //if size is 0 return true else return false } //WH TODO: Finish the resize method and test it private void resize() { //create a temporary array temp with capacity V.length+increment //copy all elements from V to temp //assign temp to V. return; } public int capacity() { return V.length; } public void addBack(int e) { //test first if it's not full if(size == V.length) { System.out.println("The vector is full resizing"); this.resize(); } //insert e at index size V[size] = e; size++; } public void add(int e) { this.addBack(e); } //HW TODO: fix toString so that the last comma does not appear //toString to return the array as a string in the form [-1, -5, -7] public String toString() { if(this.isEmpty()) { return "[]"; } String st = "["; for(int i =0; i
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 1CP: In previous chapters, you have created programs for the Greenville Idol competition. Now create a...
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
* Design a class ourVector that mimics the javaVector. The class has the following
* properties:
- A private variable size that keeps track of how many elements in the array
- A reference to an array of integers
- An increment by how much to increase wjen it's full
- A default constructor that creates an array of 5 with increment 10;
- A constructor that accepts the initial capacity and creates an array of that capacity
and an increment of twice the capacity
- A constructor that accepts two integers, the first for the capacity and the second for
increment.
- Capacity, the length of the array
- int size(); A method that returns the size of the array
- boolean isEmpty(); returns true if it's empty and false if not.
- void addBack(int e); adds e to the back of the array
- void addFront(int e); adds e to the front of the array.
- Override String toString(); to return the content of the array in the form
[-1, -4, -6]
- int elementAt(int ind); returns the elements at index ind, if valid.
- int removeBack(); removes the element in the back of the array and returns it (update size)
- int removeFront(); removes the element in the front of the array and returns it (update size)
- void add(int ind, int e); adds e to the index ind.
- int remove(int ind); removes element at index ind and returns it.
*/
public class ourVector {
//private variables
private int size;
//Let's use .length fro capacity no need for another variable
private int increment;
private int[] V; //V is a reference
//constructors
public ourVector() {
V = new int[5];
size = 0;
increment = 10;
}
public ourVector(int capacity) {
V = new int[capacity];
size = 0;
increment = 2*capacity;
}
public ourVector(int capacity, int incr) {
V = new int[capacity];
size = 0;
increment = incr;
}
//methods
public int size() {
return size;
}
public boolean isEmpty() {
return (size == 0); //if size is 0 return true else return false
}
//WH TODO: Finish the resize method and test it
private void resize() {
//create a temporary array temp with capacity V.length+increment
//copy all elements from V to temp
//assign temp to V.
return;
}
public int capacity() {
return V.length;
}
public void addBack(int e) {
//test first if it's not full
if(size == V.length) {
System.out.println("The vector is full resizing");
this.resize();
}
//insert e at index size
V[size] = e;
size++;
}
public void add(int e) {
this.addBack(e);
}
//HW TODO: fix toString so that the last comma does not appear
//toString to return the array as a string in the form [-1, -5, -7]
public String toString() {
if(this.isEmpty()) {
return "[]";
}
String st = "[";
for(int i =0; i<size; i++ ) {
st += V[i] + ", ";
}
//close it with a closing bracket
st += "]";
return st;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
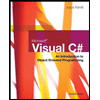
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
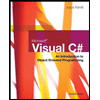
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,