do the following in the code The shell environment should contain shell=/myshell where /myshell is the full path for the shell executable (not a hardwired path back to your directory, but the one from which it was executed). #!/bin/bash flag=0 echo "Welcome to myshell" while [[ $flag == 0 ]] do read -p "myshell > " str #spilliting the text IFS=' ' #setting space as delimiter read -ra ARR <<<"$str" #reading str as an array as tokens separated by IFS command=0 # >>>>>>>>>>>>>>>>>>>>>>>>>> 1. cd <<<<<<<<<<<<<<<<<<<<<<<<< if [[ ${ARR[0]} == "cd" ]] then command=1 word=$(echo ${ARR[@]} | wc -w) # after cd hav no argument then report to current directory if [[ $word == 1 ]] then cd $PWD else if [[ -d ${ARR[1]} ]] then cd ${ARR[1]} else echo "[${ARR[1]}] path is not correct" fi fi fi #>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> 2. cls<<<<<<<<<<<<<<<<<<<<<<<< if [[ ${ARR[0]} == "cls" ]] then command=1 word=$(echo ${ARR[@]} | wc -w) # if cls have no argument then clear the screen if [[ $word == 1 ]] then clear else echo "Error: [${ARR[@]}] is wrong command." fi fi # >>>>>>>>>>>>>>>>>>>>>>>>>>>>>>3. dir<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<< if [[ ${ARR[0]} == "dir" ]] then command=1 word=$(echo ${ARR[@]} | wc -w) # if my cp have no argument if [[ $word == 1 ]] then dir else dir ${ARR[1]} fi fi # >>>>>>>>>>>>>>>>>>>>>>>>>>>> 4. copy <<<<<<<<<<<<<<<<<<<<<<<<<<< if [[ ${ARR[0]} == "copy" ]] then command=1 word=$(echo ${ARR[@]} | wc -w) # if my cp have no argument if [[ $word != 3 ]] then echo "add valid source folder name and destination folder name after mycp" else if [[ -d ${ARR[1]} ]]; then rsync -a ${ARR[1]} ${ARR[2]} echo "Success: folder [${ARR[1]}] is copied to folder [${ARR[2]}]." else echo "Error: ${ARR[1]} folder does not exist." fi fi fi # >>>>>>>>>>>>>>>>>>>>>>>>>>>> 5. print <<<<<<<<<<<<<<<<<<<<<<<<<<< if [[ ${ARR[0]} == "print" ]] then command=1 word=$(echo ${ARR[@]} | wc -w) # if print have no argument if [[ $word == 1 ]] then echo else p=0 for i in ${ARR[@]}; do if [[ $p == 0 ]] then p=1 else printf "$i " fi done echo fi fi # >>>>>>>>>>>>>>>>>>>>>>>>>>>> 6. md <<<<<<<<<<<<<<<<<<<<<<<<<<< if [[ ${ARR[0]} == "md" ]] then command=1 word=$(echo ${ARR[@]} | wc -w) # md to create folder if [[ $word == 1 ]] then echo "Error: you have not given folder path" else if [[ ! -d ${ARR[1]} ]] then mkdir -p ${ARR[1]} echo "Success: [${ARR[1]}] folder created" else echo "Error: [${ARR[1]}] folder is present" fi fi fi # >>>>>>>>>>>>>>>>>>>>>>>>>>>> 7. rd <<<<<<<<<<<<<<<<<<<<<<<<<<< if [[ ${ARR[0]} == "rd" ]] then command=1 word=$(echo ${ARR[@]} | wc -w) # md to create folder if [[ $word == 1 ]] then echo "Error: you have not given folder path" else if [[ -d ${ARR[1]} ]] then rmdir -p ${ARR[1]} echo "Success: [${ARR[1]}] folder deleted" else echo "Error: [${ARR[1]}] folder is not present" fi fi fi # 8. exit from current shell and return to original shell if [[ ${ARR[0]} == "quit" ]] then flag=1 command=1 fi # 9. command given wrong then if [[ $command == 0 ]] then echo "Command not found" fi
- do the following in the code
The shell environment should contain shell=<pathname>/myshell where <pathname>/myshell is the full path for the shell executable (not a hardwired path back to your directory, but the one from which it was executed).
#!/bin/bash
flag=0
echo "Welcome to myshell"
while [[ $flag == 0 ]]
do
read -p "myshell > " str
#spilliting the text
IFS=' ' #setting space as delimiter
read -ra ARR <<<"$str" #reading str as an array as tokens separated by IFS
command=0
# >>>>>>>>>>>>>>>>>>>>>>>>>> 1. cd <<<<<<<<<<<<<<<<<<<<<<<<<
if [[ ${ARR[0]} == "cd" ]]
then
command=1
word=$(echo ${ARR[@]} | wc -w)
# after cd hav no argument then report to current directory
if [[ $word == 1 ]]
then
cd $PWD
else
if [[ -d ${ARR[1]} ]]
then
cd ${ARR[1]}
else
echo "[${ARR[1]}] path is not correct"
fi
fi
fi
#>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> 2. cls<<<<<<<<<<<<<<<<<<<<<<<<
if [[ ${ARR[0]} == "cls" ]]
then
command=1
word=$(echo ${ARR[@]} | wc -w)
# if cls have no argument then clear the screen
if [[ $word == 1 ]]
then
clear
else
echo "Error: [${ARR[@]}] is wrong command."
fi
fi
# >>>>>>>>>>>>>>>>>>>>>>>>>>>>>>3. dir<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
if [[ ${ARR[0]} == "dir" ]]
then
command=1
word=$(echo ${ARR[@]} | wc -w)
# if my cp have no argument
if [[ $word == 1 ]]
then
dir
else
dir ${ARR[1]}
fi
fi
# >>>>>>>>>>>>>>>>>>>>>>>>>>>> 4. copy <<<<<<<<<<<<<<<<<<<<<<<<<<<
if [[ ${ARR[0]} == "copy" ]]
then
command=1
word=$(echo ${ARR[@]} | wc -w)
# if my cp have no argument
if [[ $word != 3 ]]
then
echo "add valid source folder name and destination folder name after mycp"
else
if [[ -d ${ARR[1]} ]];
then
rsync -a ${ARR[1]} ${ARR[2]}
echo "Success: folder [${ARR[1]}] is copied to folder [${ARR[2]}]."
else
echo "Error: ${ARR[1]} folder does not exist."
fi
fi
fi
# >>>>>>>>>>>>>>>>>>>>>>>>>>>> 5. print <<<<<<<<<<<<<<<<<<<<<<<<<<<
if [[ ${ARR[0]} == "print" ]]
then
command=1
word=$(echo ${ARR[@]} | wc -w)
# if print have no argument
if [[ $word == 1 ]]
then
echo
else
p=0
for i in ${ARR[@]};
do
if [[ $p == 0 ]]
then
p=1
else
printf "$i "
fi
done
echo
fi
fi
# >>>>>>>>>>>>>>>>>>>>>>>>>>>> 6. md <<<<<<<<<<<<<<<<<<<<<<<<<<<
if [[ ${ARR[0]} == "md" ]]
then
command=1
word=$(echo ${ARR[@]} | wc -w)
# md to create folder
if [[ $word == 1 ]]
then
echo "Error: you have not given folder path"
else
if [[ ! -d ${ARR[1]} ]]
then
mkdir -p ${ARR[1]}
echo "Success: [${ARR[1]}] folder created"
else
echo "Error: [${ARR[1]}] folder is present"
fi
fi
fi
# >>>>>>>>>>>>>>>>>>>>>>>>>>>> 7. rd <<<<<<<<<<<<<<<<<<<<<<<<<<<
if [[ ${ARR[0]} == "rd" ]]
then
command=1
word=$(echo ${ARR[@]} | wc -w)
# md to create folder
if [[ $word == 1 ]]
then
echo "Error: you have not given folder path"
else
if [[ -d ${ARR[1]} ]]
then
rmdir -p ${ARR[1]}
echo "Success: [${ARR[1]}] folder deleted"
else
echo "Error: [${ARR[1]}] folder is not present"
fi
fi
fi
# 8. exit from current shell and return to original shell
if [[ ${ARR[0]} == "quit" ]]
then
flag=1
command=1
fi
# 9. command given wrong then
if [[ $command == 0 ]]
then
echo "Command not found"
fi
done

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

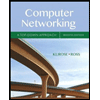
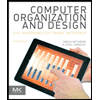
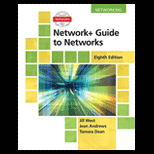
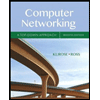
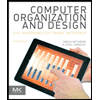
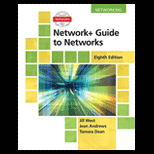
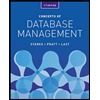
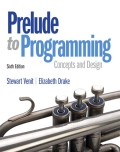
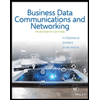