For this exercise, you are given 3 Object class objects and 3 Ball class objects. In theory, all three Objects are the same and all 3 Ball objects are the same, but based on the way they are created and the way that Java evaluates equality, not all three objects will be equal. Your task is to start by printing out the 6 Object and Ball objects. Remember, the Object class has a toString and since a Ball object extends the Object class, we should see similarities between the Object and Ball class. After printing them out, you should notice which objects are the same. Write 2 true and false statements for each group, one using == and one using .equals. Will these be the same? ========================================= public class TestEquals { public static void main(String[] args) { System.out.println("** Object **"); Object obj1 = new Object(); Object obj2 = new Object(); Object obj3 = obj1; // Print out all three objects // Print a true statement using 2 objects and == // Print a false statement using 2 objects and == // Print a true statement using 2 objects and .equals() // Print a false statement using 2 objects and .equals() System.out.println("** Ball **"); Ball ball1 = new Ball("Red"); Ball ball2 = new Ball("Red"); Ball ball3 = ball1; // Print out all three objects // Print a true statement using 2 objects and == // Print a false statement using 2 objects and == // Print a true statement using 2 objects and .equals() // Print a false statement using 2 objects and .equals() } } ========================================= public class Ball { private String color; public Ball(String color){ this.color = color; } public String getColor(){ return color; }
For this exercise, you are given 3 Object class objects and 3 Ball class objects. In theory, all three Objects are the same and all 3 Ball objects are the same, but based on the way they are created and the way that Java evaluates equality, not all three objects will be equal.
Your task is to start by printing out the 6 Object and Ball objects. Remember, the Object class has a toString and since a Ball object extends the Object class, we should see similarities between the Object and Ball class.
After printing them out, you should notice which objects are the same. Write 2 true and false statements for each group, one using == and one using .equals. Will these be the same?
public class TestEquals
{
public static void main(String[] args)
{
System.out.println("** Object **");
Object obj1 = new Object();
Object obj2 = new Object();
Object obj3 = obj1;
// Print out all three objects
// Print a true statement using 2 objects and ==
// Print a false statement using 2 objects and ==
// Print a true statement using 2 objects and .equals()
// Print a false statement using 2 objects and .equals()
System.out.println("** Ball **");
Ball ball1 = new Ball("Red");
Ball ball2 = new Ball("Red");
Ball ball3 = ball1;
// Print out all three objects
// Print a true statement using 2 objects and ==
// Print a false statement using 2 objects and ==
// Print a true statement using 2 objects and .equals()
// Print a false statement using 2 objects and .equals()
}
}
=========================================
public class Ball
{
private String color;
public Ball(String color){
this.color = color;
}
public String getColor(){
return color;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

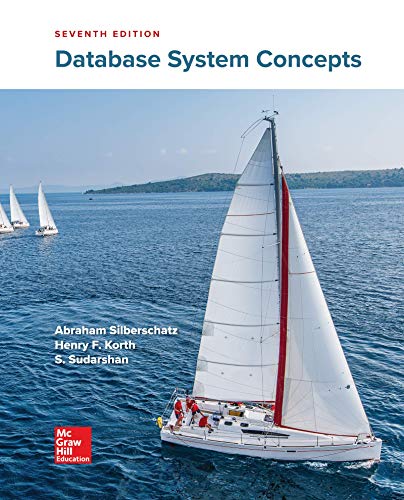
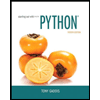
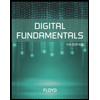
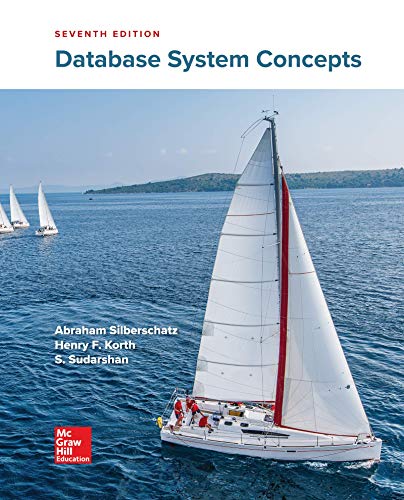
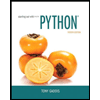
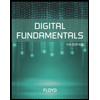
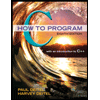
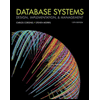
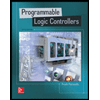