Help fix my Java Code: This is my current calendar:[ fig : 1] --------------------------------- Display an annual calendar in the header section place buttons that will move forward and backward by a year place a button in the header that will allow the user to return to the current day (or current month What the calendar needs to look like: [ fig 2 ] My current code is in seperate files the js file, html file, and css file. The code needs to stay in the seperate files. How do I modify my current code to display the new calendar? js file code: var thisDay = new Date(); var currentDay = thisDay; /* Write the calendar to the element with the id "calendar" */ document.getElementById("calendar").innerHTML = createAnnualCalendar(currentDay); function createAnnualCalendar(calDate) { var calendarHTML = ""; calendarHTML += putControlBar(); calendarHTML += ""; for (var i = 0; i < 12; i++) { calDate.setMonth(i); calendarHTML += "" + createCalendar(calDate) + ""; } calendarHTML += ""; return calendarHTML; } function createCalendar(calDate) { var calendarHTML = ""; calendarHTML += calCaption(calDate); calendarHTML += calWeekdayRow(); calendarHTML += calDays(calDate); return calendarHTML; } function calCaption(calDate) { var monthName = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; var thisMonth = calDate.getMonth(); var thisYear = calDate.getFullYear(); return "" + monthName[thisMonth] + " " + thisYear + ""; } function calWeekdayRow() { var dayName = ["S", "M", "T", "W", "T", "F", "S"]; var rowHTML = ""; for (var i = 0; i < dayName.length; i++) { rowHTML += "" + dayName[i] + ""; } rowHTML += ""; return rowHTML; } function daysInMonth(calDate) { var dayCount = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]; var thisYear = calDate.getFullYear(); var thisMonth = calDate.getMonth(); if (thisYear % 4 === 0) { dayCount[1] = 29; if ((thisYear % 100 != 0) || (thisYear % 400 === 0)) { dayCount[1] = 29; } } return dayCount[thisMonth]; } function calDays(calDate) { var day = new Date(calDate.getFullYear(), calDate.getMonth(), 1); var weekDay = day.getDay(); var htmlCode = ""; for (var i = 0; i < weekDay; i++) { htmlCode += "" } var totalDays = daysInMonth(calDate); var highlightDay = calDate.getDate(); for (var i = 1; i <= totalDays; i++) { day.setDate(i); weekDay = day.getDay(); if (weekDay === 0) { htmlCode += ""; } if (i === highlightDay) { if (weekDay === 0 || weekDay === 6) { htmlCode += "" + i + ""; } else { htmlCode += "" + i + ""; } } else { if (weekDay === 0 || weekDay === 6) { htmlCode += "" + i + ""; } else { htmlCode += "" + i + ""; } } if (i == totalDays) { if (weekDay < 6) { for (var x = weekDay; x < 6; x++) { htmlCode += ""; } } } if (weekDay === 6) htmlCode += ""; } return htmlCode; } function putControlBar() { var cbarHtml = ""; cbarHtml += " "; cbarHtml += " Previous Year "; cbarHtml += " < "; cbarHtml += " Today "; cbarHtml += " > "; cbarHtml += " Next Year "; cbarHtml += " "; return cbarHtml; } function moveYearBackward() { var curYear = currentDay.getFullYear(); var newDate = new Date(curYear - 1, 0, 1); currentDay = newDate; document.getElementById("calendar").innerHTML = createAnnualCalendar(currentDay); } function moveYearForward() { var curYear = currentDay.getFullYear(); var newDate = new Date(curYear + 1, 0, 1); currentDay = newDate; document.getElementById("calendar").innerHTML = createAnnualCalendar(currentDay); } function moveToCurrent() { currentDay = new Date(); document.getElementById("calendar").innerHTML = createAnnualCalendar(currentDay); } html file code: css file code: td, th { text-align: center; } caption { color: white; background-color: black; } #controlbar { background-color: antiquewhite; width: 200px; } #calendar_table { background-color: antiquewhite; width: 200px; } #calendar_today { color: red; background-color: powderblue; } .calendar_weekdays { background-color: grey; color: white; } .weekend { background-color: powderblue; } .padcell { background-color: antiquewhite; } caption { color: white; background-color: black; padding: 0; margin: 0; }
Help fix my Java Code:
This is my current calendar:[ fig : 1]
---------------------------------
- Display an annual calendar
- in the header section place buttons that will move forward and backward by a year
- place a button in the header that will allow the user to return to the current day (or current month
What the calendar needs to look like: [ fig 2 ]
My current code is in seperate files the js file, html file, and css file. The code needs to stay in the seperate files. How do I modify my current code to display the new calendar?
js file code:
var thisDay = new Date();
var currentDay = thisDay;
/* Write the calendar to the element with the id "calendar" */
document.getElementById("calendar").innerHTML = createAnnualCalendar(currentDay);
function createAnnualCalendar(calDate) {
var calendarHTML = "";
calendarHTML += putControlBar();
calendarHTML += "<table id='calendar_table'>";
for (var i = 0; i < 12; i++) {
calDate.setMonth(i);
calendarHTML += "<tr><td>" + createCalendar(calDate) + "</td></tr>";
}
calendarHTML += "</table>";
return calendarHTML;
}
function createCalendar(calDate) {
var calendarHTML = "";
calendarHTML += calCaption(calDate);
calendarHTML += calWeekdayRow();
calendarHTML += calDays(calDate);
return calendarHTML;
}
function calCaption(calDate) {
var monthName = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
var thisMonth = calDate.getMonth();
var thisYear = calDate.getFullYear();
return "<caption>" + monthName[thisMonth] + " " + thisYear + "</caption>";
}
function calWeekdayRow() {
var dayName = ["S", "M", "T", "W", "T", "F", "S"];
var rowHTML = "<tr>";
for (var i = 0; i < dayName.length; i++) {
rowHTML += "<th class='calendar_weekdays'>" + dayName[i] + "</th>";
}
rowHTML += "</tr>";
return rowHTML;
}
function daysInMonth(calDate) {
var dayCount = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31];
var thisYear = calDate.getFullYear();
var thisMonth = calDate.getMonth();
if (thisYear % 4 === 0) {
dayCount[1] = 29;
if ((thisYear % 100 != 0) || (thisYear % 400 === 0)) {
dayCount[1] = 29;
}
}
return dayCount[thisMonth];
}
function calDays(calDate) {
var day = new Date(calDate.getFullYear(), calDate.getMonth(), 1);
var weekDay = day.getDay();
var htmlCode = "<tr>";
for (var i = 0; i < weekDay; i++) {
htmlCode += "<td class='padcell'></td>"
}
var totalDays = daysInMonth(calDate);
var highlightDay = calDate.getDate();
for (var i = 1; i <= totalDays; i++) {
day.setDate(i);
weekDay = day.getDay();
if (weekDay === 0) {
htmlCode += "<tr>";
}
if (i === highlightDay) {
if (weekDay === 0 || weekDay === 6) {
htmlCode += "<td class='calendar_dates weekend' id='calendar_today'>" + i + "</td>";
} else {
htmlCode += "<td class='calendar_dates' id='calendar_today'>" + i + "</td>";
}
} else {
if (weekDay === 0 || weekDay === 6) {
htmlCode += "<td class='calendar_dates weekend'>" + i + "</td>";
} else {
htmlCode += "<td class='calendar_dates'>" + i + "</td>";
}
}
if (i == totalDays) {
if (weekDay < 6) {
for (var x = weekDay; x < 6; x++) {
htmlCode += "<td class='padcell'></td>";
}
}
}
if (weekDay === 6) htmlCode += "</tr>";
}
return htmlCode;
}
function putControlBar() {
var cbarHtml = "";
cbarHtml += "<table id='controlbar'> <tr>";
cbarHtml += "<td> <button id='prevYearBtn' onclick='moveYearBackward()'>Previous Year</button> </td>";
cbarHtml += "<td> <button id='prevMonthBtn' onclick='moveMonth(-1)'><</button> </td>";
cbarHtml += "<td> <button id='todayBtn' onclick='moveToCurrent()'>Today</button> </td>";
cbarHtml += "<td> <button id='nextMonthBtn' onclick='moveMonth(1)'>></button> </td>";
cbarHtml += "<td> <button id='nextYearBtn' onclick='moveYearForward()'>Next Year</button> </td>";
cbarHtml += "</tr> </table>";
return cbarHtml;
}
function moveYearBackward() {
var curYear = currentDay.getFullYear();
var newDate = new Date(curYear - 1, 0, 1);
currentDay = newDate;
document.getElementById("calendar").innerHTML = createAnnualCalendar(currentDay);
}
function moveYearForward() {
var curYear = currentDay.getFullYear();
var newDate = new Date(curYear + 1, 0, 1);
currentDay = newDate;
document.getElementById("calendar").innerHTML = createAnnualCalendar(currentDay);
}
function moveToCurrent() {
currentDay = new Date();
document.getElementById("calendar").innerHTML = createAnnualCalendar(currentDay);
}
html file code:
<html>
<head>
<link href="calendar.css" rel="stylesheet" />
<script src="newcalendar.js" defer></script>
</head>
<body>
<div id="calendar"></div>
</body>
</html>
css file code:
td, th {
text-align: center;
}
caption {
color: white;
background-color: black;
}
#controlbar {
background-color: antiquewhite;
width: 200px;
}
#calendar_table {
background-color: antiquewhite;
width: 200px;
}
#calendar_today {
color: red;
background-color: powderblue;
}
.calendar_weekdays {
background-color: grey;
color: white;
}
.weekend {
background-color: powderblue;
}
.padcell {
background-color: antiquewhite;
}
caption {
color: white;
background-color: black;
padding: 0;
margin: 0;
}



Step by step
Solved in 5 steps with 8 images

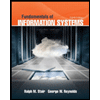
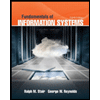