HOW DO I GET THE PROGRAM TO READ THE INFORMATION FROM US.txt AND THEN PUT IT INTO AN ARRAY SO THAT THE REST OF CODE WORKS AND THE END RESULT IS THIS
import java.util.*;
import java.io.*;
class PopulationData {
private int[] population;
// Constructor with array object as parameter
public PopulationData(int[] population) {
this.population = population;
}
// Method to calculate average annual change in population
public int averageAnnualChange() {
int sum = 0;
for (int i = 0; i < population.length - 1; i++) {
sum += population[i + 1] - population[i];
}
return sum / (population.length - 1);
}
// Method to find year with greatest increase in population
public int yearWithGreatestIncrease() {
int maxIncrease = 0;
int maxYear = 0;
for (int i = 0; i < population.length - 1; i++) {
int increase = population[i + 1] - population[i];
if (increase > maxIncrease) {
maxIncrease = increase;
maxYear = i + 1 + 1950; // convert index to year
}
}
return maxYear;
}
// Method to find year with smallest increase in population
public int yearWithSmallestIncrease() {
double minIncrease = Double.MAX_VALUE;
int minYear = 0;
for (int i = 0; i < population.length - 1; i++) {
int increase = population[i + 1] - population[i];
if (increase < minIncrease) {
minIncrease = increase;
minYear = i + 1 + 1950; // convert index to year
}
}
return minYear;
}
// Method to find population in a specific year
public int populationInYear(int year) {
return population[year - 1950]; // convert year to index
}
// Method to display or return population in a specific period
public void populationInPeriod(int startYear, int endYear) {
System.out.printf("The population in thousands from %d through %d:\n", startYear, endYear);
for (int i = startYear - 1950; i <= endYear - 1950; i++) { // convert years to indices
System.out.printf("%.0f ", population[i]);
}
System.out.println();
}
}
public class PopulationDemo {
private static final int START_YEAR = 1950;
private static final int END_YEAR = 1990;
public static void main(String[] args) throws IOException {
int numYears = 1990 - 1950 + 1;
File file = new File("US.txt");
FileReader inputFile = new FileReader(file);
BufferedReader in = new BufferedReader(inputFile);
int[] population = new int [numYears];
int i = 0;
String s =in.readLine();
while(s!=null)
{
population[i] = Integer.parseInt(s); //this is line 19
s = in.readLine();
}
System.out.println(population[i]);
PopulationData p = new PopulationData(population);
System.out.printf("In population from %d through %d\n", START_YEAR, END_YEAR);
System.out.println("-------------------------------------------------------");
System.out.printf("The number of year: %d\n", population.length);
System.out.printf("The average annual change was %.2f\n", p.averageAnnualChange());
System.out.printf("The year with the greatest increase was %d. The population was %.0f thousands.\n",
p.yearWithGreatestIncrease(), p.populationInYear(p.yearWithGreatestIncrease()));
System.out.printf("The year with the smallest increase was %d. The population was %.0f thousands.\n",
p.yearWithSmallestIncrease(), p.populationInYear(p.yearWithSmallestIncrease()));
Scanner scanner = new Scanner(System.in);
String choice = "y";
while (choice.equals("y")) {
System.out.print("\nPlease enter a year you are looking for: ");
int year = scanner.nextInt();
if (year >= START_YEAR && year <= END_YEAR) {
System.out.printf("The population in %d was %.0f thousands.\n", year, p.populationInYear(year));
} else {
System.out.printf("Invalid year. Please enter a year between %d and %d.\n", START_YEAR, END_YEAR);
}
System.out.print("Do you want to continue searching? (y/n): ");
choice = scanner.next();
}
System.out.println();
p.populationInPeriod(START_YEAR, END_YEAR);
}
}
US.txt
151868
153982
156393
158956
161884
165069
168088
171187
174149
177135
179979
182992
185771
188483
191141
193526
195576
197457
199399
201385
203984
206827
209284
211357
213342
215465
217563
219760
222095
224567
227225
229466
231664
233792
235825
237924
240133
242289
244499
246819
249623
HOW DO I GET THE PROGRAM TO READ THE INFORMATION FROM US.txt AND THEN PUT IT INTO AN ARRAY SO THAT THE REST OF CODE WORKS AND THE END RESULT IS THIS


Step by step
Solved in 3 steps with 1 images

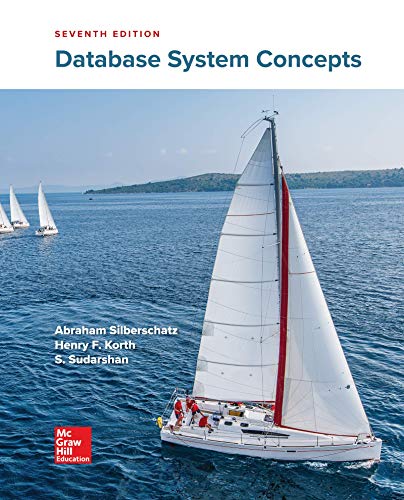
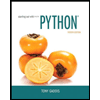
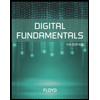
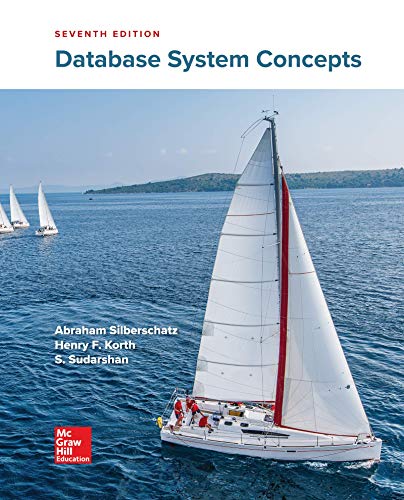
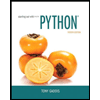
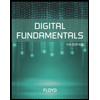
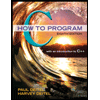
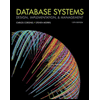
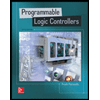