How do I write the code for a simple animation in java following the example below?
How do I write the code for a simple animation in java following the example below?
In informal language, here is an example description of the animation for the "Simple Animation" example above:
Create red rectangle R with corner at (200,200), width 50 and height 100 |
Create blue oval C with center at (500,100), radius 60 and 30 |
R appears at time t=1 and disappears at time t=100 |
C appears at time t=6 and disappears at time t=100 |
R moves from (200,200) to (300,300) from time t=10 to t=50 |
C moves from (500,100) to (500,400) from time t=20 to t=70 |
C changes from blue to green from time t=50 to t=80 |
R moves from (300,300) to (200,200) from time t=70 to t=100 |
R changes width from 50 to 25 from time t=51 to t=70 |
We use a time reference for each step in the animation. This time reference is usually unitless, which helps us in describing animation without mentioning its absolute speed. Further, the animation must adhere to certain constraints. For example, one cannot have move the same rectangle to the left and right during overlapping time intervals.
Our application may then use such a description to:
-
"Show" the animation in various ways. For example interactively play it, describe it on a time line, or even export the animation as a movie.
-
Allow a user to edit it or change speed.
-
Produce a verbose description for what the animation will look like (for the visually impaired).
-
Other possibilities...
The Easy Animator Application
We will build this application progressively using the classic Model-View-Controller architecture. We will focus on the model of this application. You are also not being told about how exactly the application will receive a description from the user (it would not be relevant to the model).
Here are some aspects to think about:
-
What does the model represent? Remember: this is an animation application, so think "application model" here.
-
The model should be able to support various kinds of 2D shapes, although currently we have described only rectangles and ovals.
-
The model should support adding various kinds of animations to shapes, such as moving, changing color, changing shape, etc. For now we will concentrate only on moving, scaling and changing color (see above animations for examples).
-
Remember to think from not only the implementor’s perspective (the person that is implementing the model) but also the client perspective (the person or class that is using the model).
How is the animation seen?
One way the application may show the animation is by actually playing it, similar to the moving images above. Another way would be to produce a text description of the animation. Here is what a description might look like:
Shapes: |
Name: R |
Type: rectangle |
Min corner: (200.0,200.0), Width: 50.0, Height: 100.0, Color: (1.0,0.0,0.0) |
Appears at t=1 |
Disappears at t=100 |
Name: C |
Type: oval |
Center: (500.0,100.0), X radius: 60.0, Y radius: 30.0, Color: (0.0,0.0,1.0) |
Appears at t=6 |
Disappears at t=100 |
Shape R moves from (200.0,200.0) to (300.0,300.0) from t=10 to t=50 |
Shape C moves from (500.0,100.0) to (500.0,400.0) from t=20 to t=70 |
Shape C changes color from (0.0,0.0,1.0) to (0.0,1.0,0.0) from t=50 to t=80 |
Shape R scales from Width: 50.0, Height: 100.0 to Width: 25.0, Height: 100.0 from t=51 to t=70 |
Shape R moves from (300.0,300.0) to (200.0,200.0) from t=70 to t=100 |
In short, it first describes the shapes that are part of the animation and their details. Next it describes how these shapes will move as the animation proceeds from start to finish. You may think of this output as a “read-back” of the animation, perhaps for devices that cannot show the animation, or for users who are visually impaired who have screen readers.
What to do
-
Design a model to represent an animation. This model may consist of one or more interfaces, abstract classes, concrete classes, enums, etc. Consider carefully what operations it should support. Create a high-level UML class diagram to represent your design.
-
Think about and include in the model interface operations that you think are relevant and the model should offer.
-
Document your model well. Be sure to document clearly what each method does, what purpose it serves and why it belongs in the model.
-
Implement your model. Only if you do not know how to implement the method because it requires details of animation not provided yet, you may leave the method body blank for now. This will convey to us that you think the method ought to be part of the model.
-
Test your model.
-
Implement the text output rendering of your model according to the format given in the previous section (How is the animation seen?), so we can visualize your data.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

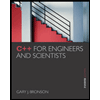
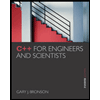