I would like to represent the "residuals" part of the code (see below) as a surface (meshplot), in essence a 3D plot of 2D data # Import libraries import matplotlib.pyplot as plt import glob from astropy.io import fits from astropy.wcs import WCS import numpy as np import seaborn as sns # Initial plot fig, ax = plt.subplots(figsize=(7, 7)) # Data from the FITS data_dir = glob.glob('/Users/petrderuyter/Desktop/harpn_sun_release_package_ccf_2018/2018-04-02/*.fits') #'/Users/xxxxxxxxxxxx/Desktop/harpn_sun_release_package_ccf_2018/2018-01-18/*.fits' # Empty lists for storage lams = [] fluxs = [] # Read the FITS files and extract data for file_path in data_dir: hdul = fits.open(file_path) data = hdul[1].data h1 = hdul[1].header flux = data[1] w = WCS(h1, naxis=1, relax=False, fix=False) lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0] lams.append(lam) fluxs.append(flux) ax.plot(lam, flux) # Calculate mean flux mean_ccf = np.mean(fluxs, axis=0) # New figure for the mean plot fig_mean, ax_mean = plt.subplots(figsize=(7, 7)) # Mean data plot ax_mean.plot(lams[0], mean_ccf, linewidth=1) # Set labels and title for the mean plot ax_mean.set_xlabel('RV [km/s]') ax_mean.set_ylabel('Normalized CCF') ax_mean.set_title('Mean CCF') # Calculate residuals fluxs_array = np.array(fluxs) residuals = fluxs_array - mean_ccf # Residuals data plot fig_res, ax_res = plt.subplots(figsize=(7, 7)) for i in range(len(lams)): ax_res.plot(lams[i], residuals[i], linewidth=1) # Set labels and title for the residuals plot ax_res.set_xlabel('RV [km/s]') ax_res.set_ylabel('CCF Residuals') ax_res.set_title('CCF Residuals') plt.show()
I would like to represent the "residuals" part of the code (see below) as a surface (meshplot), in essence a 3D plot of 2D data
# Import libraries
import matplotlib.pyplot as plt
import glob
from astropy.io import fits
from astropy.wcs import WCS
import numpy as np
import seaborn as sns
# Initial plot
fig, ax = plt.subplots(figsize=(7, 7))
# Data from the FITS
data_dir = glob.glob('/Users/petrderuyter/Desktop/harpn_sun_release_package_ccf_2018/2018-04-02/*.fits')
#'/Users/xxxxxxxxxxxx/Desktop/harpn_sun_release_package_ccf_2018/2018-01-18/*.fits'
# Empty lists for storage
lams = []
fluxs = []
# Read the FITS files and extract data
for file_path in data_dir:
hdul = fits.open(file_path)
data = hdul[1].data
h1 = hdul[1].header
flux = data[1]
w = WCS(h1, naxis=1, relax=False, fix=False)
lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0]
lams.append(lam)
fluxs.append(flux)
ax.plot(lam, flux)
# Calculate mean flux
mean_ccf = np.mean(fluxs, axis=0)
# New figure for the mean plot
fig_mean, ax_mean = plt.subplots(figsize=(7, 7))
# Mean data plot
ax_mean.plot(lams[0], mean_ccf, linewidth=1)
# Set labels and title for the mean plot
ax_mean.set_xlabel('RV [km/s]')
ax_mean.set_ylabel('Normalized CCF')
ax_mean.set_title('Mean CCF')
# Calculate residuals
fluxs_array = np.array(fluxs)
residuals = fluxs_array - mean_ccf
# Residuals data plot
fig_res, ax_res = plt.subplots(figsize=(7, 7))
for i in range(len(lams)):
ax_res.plot(lams[i], residuals[i], linewidth=1)
# Set labels and title for the residuals plot
ax_res.set_xlabel('RV [km/s]')
ax_res.set_ylabel('CCF Residuals')
ax_res.set_title('CCF Residuals')
plt.show()
![CCF Residuals
2500
0
-2500
-5000
-7500
-10000
-12500
-15000
0
10
CCF Residuals
20
RV [km/s]
30
40
50](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc1c1ced3-1875-44c4-84f9-6bbf6b04c124%2Fa14c940e-8a4b-4577-b616-af56654f2199%2F2hj1xvw_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

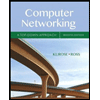
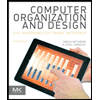
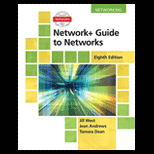
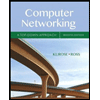
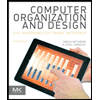
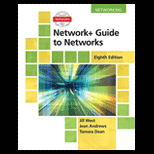
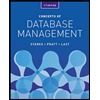
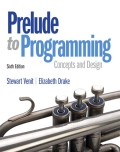
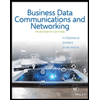