Implement a class that calculates mathematic expressions. The input passed to this Calculator is in infix notation, which gets converted to postfix internally, then the postfix expression is evaluated (we evaluate in postfix instead of infix because of how much simpler it is). More details about infix to postfix conversion can be found in the video lectures.
Implement a class that calculates mathematic expressions. The input passed to this Calculator is in infix notation, which gets converted to postfix internally, then the postfix expression is evaluated (we evaluate in postfix instead of infix because of how much simpler it is). More details about infix to postfix conversion can be found in the video lectures.
Chapter8: Advanced Method Concepts
Section: Chapter Questions
Problem 8RQ
Related questions
Question
First class was posted in this question, third class will be posted in a different question, please refer to it, thank you: https://www.bartleby.com/questions-and-answers/computer-science-question/34b2a175-1365-4989-a811-33c7e8b66a7b
Do not change the function names or given starter code in the script. Each class has different requirements, read them carefully. Do not use the exec or eval functions, nor are you allowed to use regular expressions (re module). All methods that output a string must return the string, not print it. If you are unable to complete a method, use the pass statement to avoid syntax errors.
Section 2: The Calculator class
Implement a class that calculates mathematic expressions. The input passed to this Calculator is in
infix notation, which gets converted to postfix internally, then the postfix expression is evaluated
(we evaluate in postfix instead of infix because of how much simpler it is). More details about
infix to postfix conversion can be found in the video lectures.
This calculator should support numeric values, five arithmetic operators (+, –, *, /, ^), and
parenthesis. Follow the PEMDAS order of operations (you can define precedence of operators
with a dictionary or a helper method). Note that exponentiation is ** in Python.
You can assume that expressions will have tokens (operators, operands) separated by a single space.
For the case of negative numbers, you can assume the negative sign will be prefixed to the number.
The str.split() method can be helpful to isolate tokens. Expressions are considered invalid if they
meet any of the following criteria:
• Contains unsupported operators 4 $ 5
• Contains consecutive operators 4 * + 5
• Has missing operands 4 +
• Has missing operators 4 5
• Has unbalanced parenthesis ) 4 + 5 ( or ( 4 + 5 ) )
• Tries to do implied multiplication 3(5) instead of 3 * ( 5 )
• Includes a space before a negative number 4 * - 5 instead of 4 * -5
Make sure to have proper encapsulation of your code by using proper variable scopes and writing
other helper methods to generalize your code for processes such as string processing and input
validation. Do not forget to document your code.
As a suggestion, start by implementing your methods assuming the expressions are always valid,
that way you have the logic implemented and you only need to work on validating the expressions.
Attributes
Type Name Description
str __expr The expression this calculator will evaluate
Methods
Type Name Description
None setExpr(self, new_expr) Sets the expression for the calculator to evaluate
str getExpr(self) Getter method for the private expression attribute
bool _isNumber(self, aSring) Returns True if aSring can be converted to a float
str _getPostfix(self, expr) Converts an expression from infix to postfix
float calculate(self) Calculates the expression stored in this calculator
Implement a class that calculates mathematic expressions. The input passed to this Calculator is in
infix notation, which gets converted to postfix internally, then the postfix expression is evaluated
(we evaluate in postfix instead of infix because of how much simpler it is). More details about
infix to postfix conversion can be found in the video lectures.
This calculator should support numeric values, five arithmetic operators (+, –, *, /, ^), and
parenthesis. Follow the PEMDAS order of operations (you can define precedence of operators
with a dictionary or a helper method). Note that exponentiation is ** in Python.
You can assume that expressions will have tokens (operators, operands) separated by a single space.
For the case of negative numbers, you can assume the negative sign will be prefixed to the number.
The str.split() method can be helpful to isolate tokens. Expressions are considered invalid if they
meet any of the following criteria:
• Contains unsupported operators 4 $ 5
• Contains consecutive operators 4 * + 5
• Has missing operands 4 +
• Has missing operators 4 5
• Has unbalanced parenthesis ) 4 + 5 ( or ( 4 + 5 ) )
• Tries to do implied multiplication 3(5) instead of 3 * ( 5 )
• Includes a space before a negative number 4 * - 5 instead of 4 * -5
Make sure to have proper encapsulation of your code by using proper variable scopes and writing
other helper methods to generalize your code for processes such as string processing and input
validation. Do not forget to document your code.
As a suggestion, start by implementing your methods assuming the expressions are always valid,
that way you have the logic implemented and you only need to work on validating the expressions.
Attributes
Type Name Description
str __expr The expression this calculator will evaluate
Methods
Type Name Description
None setExpr(self, new_expr) Sets the expression for the calculator to evaluate
str getExpr(self) Getter method for the private expression attribute
bool _isNumber(self, aSring) Returns True if aSring can be converted to a float
str _getPostfix(self, expr) Converts an expression from infix to postfix
float calculate(self) Calculates the expression stored in this calculator
setExpr(self, new_expr)
Sets the expression for the calculator to evaluate. This method is already implemented for you.
Input (excluding self)
str new_expr The new expression (in infix) for the calculator to evaluate
getExpr(self)
Property method for getting the expression in the calculator. This method is already implemented
for you.
Output
str The value stored in __expr
_isNumber(self, txt)
Returns True if txt is a string that can be converted to a float, False otherwise. Note that the type
conversion float('4.56') returns 4.56 but float('4 56') raises an exception. A try/except
block could be useful here.
Input (excluding self)
str txt The string to check if it represents a number
Output
bool True if txt can be successfully casted to a float, False otherwise
_getPostfix(self, expr)
Converts an expression from infix to postfix. All numbers in the output must be represented as a
float. You must use the Stack defined in section 1 in this method, otherwise your code will not
receive credit. (Stack applications video lecture can be helpful here).
Input (excluding self)
str expr The expression in infix form
Output
str The expression in postfix form
None None is returned if the input is an invalid expression
calculate(self)
A property method that evaluates the infix expression saved in self.__expr. First convert the
expression to postfix, then use a stack to evaluate the output postfix expression. You must use the
Stack defined in section 1 in this method, otherwise your code will not receive credit.
Input (excluding self)
str txt The string to check if it represents a number
Output
float The result of the expression
None None is returned if the input is an invalid expression or if expression can’t be
computed
A property method that evaluates the infix expression saved in self.__expr. First convert the
expression to postfix, then use a stack to evaluate the output postfix expression. You must use the
Stack defined in section 1 in this method, otherwise your code will not receive credit.
Input (excluding self)
str txt The string to check if it represents a number
Output
float The result of the expression
None None is returned if the input is an invalid expression or if expression can’t be
computed
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
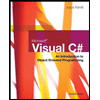
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
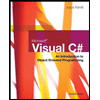
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,