import java.awt.*;import javax.swing.*;import java.util.*; // Model classclass FrogModel { private int xc; private int yc; public FrogModel() { // Initialize frog's coordinates randomly Random rng = new Random(); // Creating an instance of Random xc = rng.nextInt(200); yc = rng.nextInt(200); } public int getX() { return xc; } public int getY() { return yc; } public void leap() { // Update frog's coordinates randomly Random rng = new Random(); // Creating an instance of Random xc = rng.nextInt(200); yc = rng.nextInt(200); }} // View classclass FrogView extends JPanel { private FrogModel model; public FrogView(FrogModel model) { this.model = model; setBackground(Color.BLUE); } @Override protected void paintComponent(Graphics gc) { super.paintComponent(gc); gc.setColor(Color.GREEN); gc.fillOval(model.getX(), model.getY(), 10, 10); // Adjust size as needed }} // Controller classclass FrogController { private FrogModel model; private FrogView view; public FrogController(FrogModel model, FrogView view) { this.model = model; this.view = view; } public void leap() { model.leap(); view.repaint(); }} // Main classpublic class FroggerMVC { public static void main(String[] args) { FrogModel model = new FrogModel(); FrogView view = new FrogView(model); FrogController controller = new FrogController(model, view); // Set up GUI JFrame frame = new JFrame("Frogger"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(view); frame.pack(); frame.setLocationRelativeTo(null); frame.setVisible(true); // Leap button setup JButton leapButton = new JButton("Leap"); leapButton.addActionListener(e -> controller.leap()); frame.getContentPane().add(leapButton, BorderLayout.SOUTH); }} i am trying ti implent the code but getting " hello world" please check whats the problem i m getting ?
import java.awt.*;
import javax.swing.*;
import java.util.*;
// Model class
class FrogModel {
private int xc;
private int yc;
public FrogModel() {
// Initialize frog's coordinates randomly
Random rng = new Random(); // Creating an instance of Random
xc = rng.nextInt(200);
yc = rng.nextInt(200);
}
public int getX() {
return xc;
}
public int getY() {
return yc;
}
public void leap() {
// Update frog's coordinates randomly
Random rng = new Random(); // Creating an instance of Random
xc = rng.nextInt(200);
yc = rng.nextInt(200);
}
}
// View class
class FrogView extends JPanel {
private FrogModel model;
public FrogView(FrogModel model) {
this.model = model;
setBackground(Color.BLUE);
}
@Override
protected void paintComponent(Graphics gc) {
super.paintComponent(gc);
gc.setColor(Color.GREEN);
gc.fillOval(model.getX(), model.getY(), 10, 10); // Adjust size as needed
}
}
// Controller class
class FrogController {
private FrogModel model;
private FrogView view;
public FrogController(FrogModel model, FrogView view) {
this.model = model;
this.view = view;
}
public void leap() {
model.leap();
view.repaint();
}
}
// Main class
public class FroggerMVC {
public static void main(String[] args) {
FrogModel model = new FrogModel();
FrogView view = new FrogView(model);
FrogController controller = new FrogController(model, view);
// Set up GUI
JFrame frame = new JFrame("Frogger");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(view);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
// Leap button setup
JButton leapButton = new JButton("Leap");
leapButton.addActionListener(e -> controller.leap());
frame.getContentPane().add(leapButton, BorderLayout.SOUTH);
}
}
i am trying ti implent the code but getting " hello world" please check whats the problem i m getting ?

Step by step
Solved in 1 steps

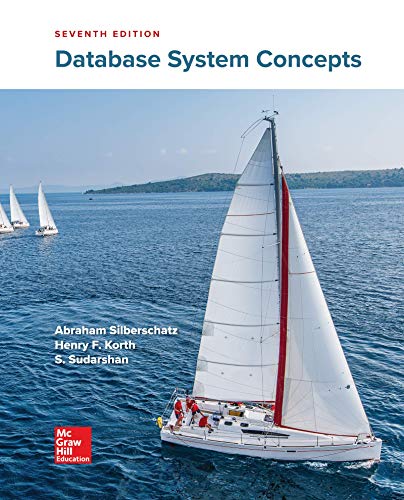
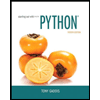
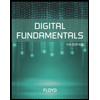
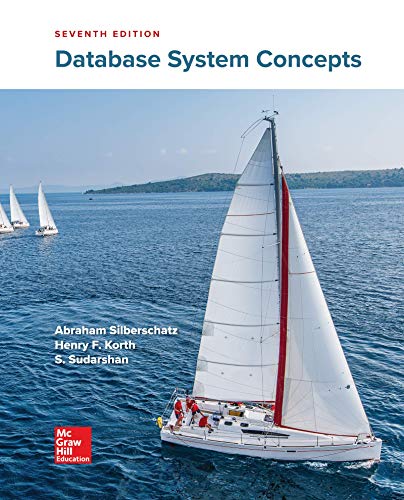
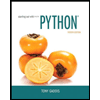
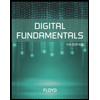
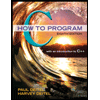
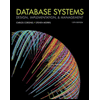
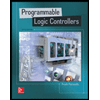