In C programing(not C++ or C#), how can I complete my code for the expected output? Comments are from Teaching Assistant. Part 1 Count the occurrences of a single character in a string. Example output: $ ./lab02 foobar o 2 $ ./lab02 foobar invalid arguments Count the occurrences of an N-character string in another string. Example output: $ ./lab02 abbabb bb 2 $ ./lab02 abbabb bc 0 You may use the C library function strstr() if you wish. char *strstr(char *a, char *b) returns a pointer to the first occurrence of b if b occurs in a, or NULL if b does not occur in a
In C programing(not C++ or C#), how can I complete my code for the expected output? Comments are from Teaching Assistant. Part 1 Count the occurrences of a single character in a string. Example output: $ ./lab02 foobar o 2 $ ./lab02 foobar invalid arguments Count the occurrences of an N-character string in another string. Example output: $ ./lab02 abbabb bb 2 $ ./lab02 abbabb bc 0 You may use the C library function strstr() if you wish. char *strstr(char *a, char *b) returns a pointer to the first occurrence of b if b occurs in a, or NULL if b does not occur in a
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C programing(not C++ or C#), how can I complete my code for the expected output? Comments are from Teaching Assistant.
Part 1
- Count the occurrences of a single character in a string. Example output:
$ ./lab02 foobar o
2
$ ./lab02 foobar
invalid arguments
- Count the occurrences of an N-character string in another string. Example output:
$ ./lab02 abbabb bb
2
$ ./lab02 abbabb bc
0
You may use the C library function strstr() if you wish. char *strstr(char *a, char *b) returns a pointer to the first occurrence of b if b occurs in a, or NULL if b does not occur in a
![@vlab01 lab02-
S$ gcc -g -o lab02 lab02.c
lab02.c: In function 'main':
lab02.c:34:6: warning: implicit declaration of function 'strlen' [-Wimplicit-function-declaration]
if (strlen(needle) == 1)) {
lab02.c:34:6: warning: incompatible implicit declaration of built-in function 'strlen'
lab02.c:34:6: note: include '<string.h>' or provide a declaration of 'strlen'
lab02.c:2:1:
+#include <string.h>
int part1 (const char str[], int c)
lab02.c:34:6:
if (strlen(needle) == 1)) {
lab02.c:34:26: error: expected statement before ')' token
if (strlen(needle)
1)) {
==
A
lab02.c:38:17: error: 'str' undeclared (first use in this function)
scanf("%s", str);
lab02.c:38:17: note: each undeclared identifier is reported only once for each function it appears in
lab02.c:39:17: error: 'ch' undeclared (first use in this function); did you mean 'char'?
scanf("%s", ch);
char
lab02.c:41:12: warning: implicit declaration of function 'str_chnum' [-Wimplicit-function-declaration]
printf(str_chnum(str, ch[0]));
lab02.c:43:1: error: expected declaration or statement at end of input
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F909d4e96-ec45-4f89-986e-8cb112f8d100%2F554a4e83-eb5c-48cc-9c3f-9dc21d6450c7%2F0jwhnyy_processed.png&w=3840&q=75)
Transcribed Image Text:@vlab01 lab02-
S$ gcc -g -o lab02 lab02.c
lab02.c: In function 'main':
lab02.c:34:6: warning: implicit declaration of function 'strlen' [-Wimplicit-function-declaration]
if (strlen(needle) == 1)) {
lab02.c:34:6: warning: incompatible implicit declaration of built-in function 'strlen'
lab02.c:34:6: note: include '<string.h>' or provide a declaration of 'strlen'
lab02.c:2:1:
+#include <string.h>
int part1 (const char str[], int c)
lab02.c:34:6:
if (strlen(needle) == 1)) {
lab02.c:34:26: error: expected statement before ')' token
if (strlen(needle)
1)) {
==
A
lab02.c:38:17: error: 'str' undeclared (first use in this function)
scanf("%s", str);
lab02.c:38:17: note: each undeclared identifier is reported only once for each function it appears in
lab02.c:39:17: error: 'ch' undeclared (first use in this function); did you mean 'char'?
scanf("%s", ch);
char
lab02.c:41:12: warning: implicit declaration of function 'str_chnum' [-Wimplicit-function-declaration]
printf(str_chnum(str, ch[0]));
lab02.c:43:1: error: expected declaration or statement at end of input
}
![1 #include <stdio.h>
2 int part1 (const char str[], int c)
3
4
int i;
int count
7
for (i
= 0; str[i] != '\0'; i++)
8
if (str[i]
count++;
10
return (count);
11 }
12
13 int part2 (const char str], int c)
{
int i;
14
15
16
int count = 0;
17
for (i = 0; str[i] != '\0'; i++)
(str[i]
count++;
return (count);
18
19
if
C)
20
21
22 }
23
char **argv)
24 int main(int argc.
25 {
argv[1];// foober
argv[2];// o
26
27
28
29
//sudo code
30
//Create pointers to argv[1] and argv[2]
31
32
//check if argv[2] is 1 character
char *needle
if (strlen(needle)
argv[2];
1)) {
33
34
35
36
//if its 1 character., call function part1()
//if its more than 1 character, call function part2(
37
str);
ch);
38
scanf("%s'
39
scanf("%s'
40
printf(str_chnum(str, ch[0]));
return (0);
41
42
43 }](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F909d4e96-ec45-4f89-986e-8cb112f8d100%2F554a4e83-eb5c-48cc-9c3f-9dc21d6450c7%2Fl4ikg0v_processed.png&w=3840&q=75)
Transcribed Image Text:1 #include <stdio.h>
2 int part1 (const char str[], int c)
3
4
int i;
int count
7
for (i
= 0; str[i] != '\0'; i++)
8
if (str[i]
count++;
10
return (count);
11 }
12
13 int part2 (const char str], int c)
{
int i;
14
15
16
int count = 0;
17
for (i = 0; str[i] != '\0'; i++)
(str[i]
count++;
return (count);
18
19
if
C)
20
21
22 }
23
char **argv)
24 int main(int argc.
25 {
argv[1];// foober
argv[2];// o
26
27
28
29
//sudo code
30
//Create pointers to argv[1] and argv[2]
31
32
//check if argv[2] is 1 character
char *needle
if (strlen(needle)
argv[2];
1)) {
33
34
35
36
//if its 1 character., call function part1()
//if its more than 1 character, call function part2(
37
str);
ch);
38
scanf("%s'
39
scanf("%s'
40
printf(str_chnum(str, ch[0]));
return (0);
41
42
43 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
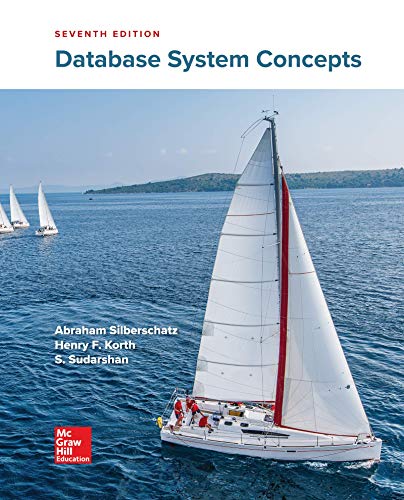
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
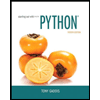
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
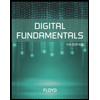
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
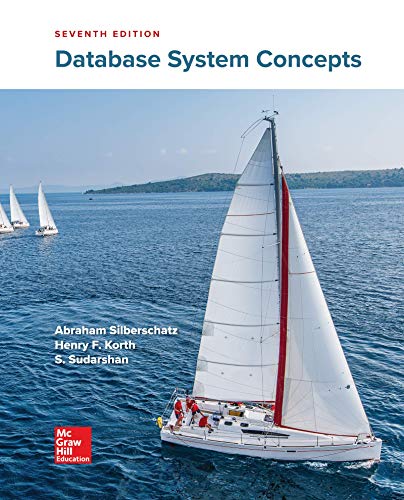
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
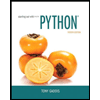
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
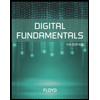
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
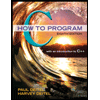
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
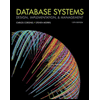
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
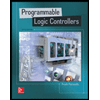
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education