In geometry, polygons are defined as plane (two-dimensional) and closed shapes that are formed by three or more straight lines joined with each other. Write a java program to develop an OOP solution presenting a regular polygon object. A regular polygon has a name, n number of sides with the same length. The perimeter of a regular polygon can be found by computing the total length of all n sides. A polygon can be a triangle that also has a height value. Polygon Triangle Create the following classes and interface: A. Interface Geometry includes a single method called perimeter () that returns a double value presenting the perimeter of a shape. B. Implement class Polygon as follows: • Include the required instance variables as described above, and a constructor method with arguments. Add two get methods to return number of sides and value of side length. • Implement the method perimeter() from the Geometry interface as the perimeter value of a polygon. • Implement area () method that returns value 0.0; • Override tostring() method to describe a polygon object; see Figure 2. C. Implement the Triangle subclass includes: • Its own instance variable(s), a constructor with argument, and get/set methods for each instance variable. • override area () method to compute and returns the area value of a triangle. Area = ;xbasexheight 2 Write the code for the tester class "PolygonTester.java" which has the following two methods: 1. readShapes () method that accepts a parameter holding a scanner object of file type and returns an arrayList of polygon objects. It reads each line from the input file, constructs and adds the corresponding a general polygon or a triangle type into the arrayList. Knowing that a triangle is a polygon with three sides; see Figure 1 for a sample input file, “shapes.txt".
In geometry, polygons are defined as plane (two-dimensional) and closed shapes that are formed
by three or more straight lines joined with each other. Write a java program to develop an OOP
solution presenting a regular polygon object. A regular polygon has a name, n number of sides
with the same length. The perimeter of a regular polygon can be found by computing the total
length of all n sides. A polygon can be a triangle that also has a height value.
Create the following classes and interface:
A. Interface Geometry includes a single method called perimeter() that returns a double
value presenting the perimeter of a shape.
B. Implement class Polygon as follows:
Include the required instance variables as described above, and a constructor method with
arguments. Add two get methods to return number of sides and value of side length.
Implement the method perimeter() from the Geometry interface as the perimeter
value of a polygon.
Implement area() method that returns value 0.0;
Override toString() method to describe a polygon object; see Figure 2.
C. Implement the Triangle subclass includes:
Its own instance variable(s), a constructor with argument, and get/set methods for each
instance variable.
override area() method to compute and returns the area value of a triangle.
Write the code for the tester class “PolygonTester.java” which has the following two methods:
1. readShapes() method that accepts a parameter holding a scanner object of file type
and returns an arrayList of polygon objects. It reads each line from the input file, constructs
and adds the corresponding a general polygon or a triangle type into the arrayList. Knowing
that a triangle is a polygon with three sides; see Figure 1 for a sample input file,
“shapes.txt”.



Step by step
Solved in 4 steps with 6 images

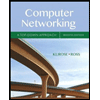
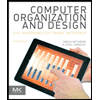
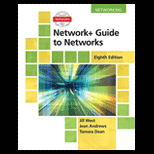
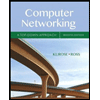
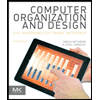
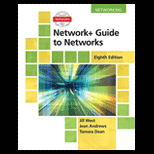
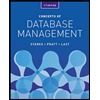
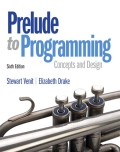
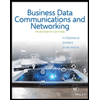