In java programming language Design a class named Account that contains: • A private double data field named annualInterestRate that stores the current interest rate. Assume all accounts have the same interest rate (i.e. static member data). • A private int data field named nextAccountNb that stores the next account number to be assigned (default 300). Assume all accounts use this data member to assign the account number while creating an account (i.e. static member data). • A private int data field named accounNb for the account. • A private String data field named f_name for first name. • A private String data field named l_name for last name. • A private double data field named balance for the account. • A private Date data field named dateCreated that stores the date when the account was created. (usejava.util.Dateclass) • A constructor that creates an account for a given first name, last name, and initial balance. It sets up a new account number, first name, last name, balance, date of account creation, and advances nextAccountNb value by 1. • Appropriate accessor and mutator methods for accountNb, f_name, l_name, balance, annualInterestRate, and dateCreated. • A method named getMonthlyInterestRate() that returns the monthly interest rate. • A method named withdraw that withdraws a specified amount from the account. • A method named deposit that deposits a specified amount to the account. You should include appropriate data validation checks, e.g. balance should not be negative, name can include spaces, etc. Implement the class. Write a test program named SwanaBank that performs the following: - Displays a welcome message “Welcome to SWANA Bank” - Sets up an interest rate of 4.5% and displays the interest rate as today’s interest rate. - Prompts the user for first name, last name. Create an account with an initial balance of $100. - Displays the account details including monthly interest on the current balance. Allows user to deposit and withdraw as many times as they want. - Finally, ends with a good bye message. Your test program should access (i.e. get or set method) the static field annualInterestRate before creating accounts, and via an account object. Please refer to the screen shot below for precise details. Here is a sample screen shot: Welcome to SWANA Bank Today's Interest Rate is = 4.5% Enter first name: Malleswara Rao Enter last name: Talla Your account details are: Name is: Malleswara Rao Talla Account Number is 300 Current balance is $100.0 Interest Rate is = 4.5% Monthly interest for this balance is $0.375 This account was created on Sun Oct 24 10:42:51 EDT 2010 Enter your choice (0: deposit, 1: withdraw, 2: quit): 0 Enter the amount in $300 Enter your choice (0: deposit, 1: withdraw, 2: quit): 0 Enter the amount in $100 Enter your choice (0: deposit, 1: withdraw, 2: quit): 1 Enter the amount in $50 Enter your choice (0: deposit, 1: withdraw, 2: quit): 1 Enter the amount in $25 Enter your choice (0: deposit, 1: withdraw, 2: quit): 2 New balance is $425.0 Good Bye!
In java
Design a class named Account that contains:
• A private double data field named annualInterestRate that stores the current interest rate.
Assume all accounts have the same interest rate (i.e. static member data).
• A private int data field named nextAccountNb that stores the next account number to be
assigned (default 300). Assume all accounts use this data member to assign the account number while creating an account (i.e. static member data).
• A private int data field named accounNb for the account.
• A private String data field named f_name for first name.
• A private String data field named l_name for last name.
• A private double data field named balance for the account.
• A private Date data field named dateCreated that stores the date when the account was
created. (usejava.util.Dateclass)
• A constructor that creates an account for a given first name, last name, and initial balance. It sets up a new account number, first name, last name, balance, date of account creation, and advances nextAccountNb value by 1.
• Appropriate accessor and mutator methods for accountNb, f_name, l_name, balance, annualInterestRate, and dateCreated.
• A method named getMonthlyInterestRate() that returns the monthly interest rate.
• A method named withdraw that withdraws a specified amount from the account.
• A method named deposit that deposits a specified amount to the account.
You should include appropriate data validation checks, e.g. balance should not be negative, name can include spaces, etc.
Implement the class. Write a test program named SwanaBank that performs the following:
- Displays a welcome message “Welcome to SWANA Bank”
- Sets up an interest rate of 4.5% and displays the interest rate as today’s interest rate.
- Prompts the user for first name, last name. Create an account with an initial balance of
$100.
- Displays the account details including monthly interest on the current balance.
Allows user to deposit and withdraw as many times as they want.
- Finally, ends with a good bye message.
Your test program should access (i.e. get or set method) the static field annualInterestRate before creating accounts, and via an account object. Please refer to the screen shot below for precise details.
Here is a sample screen shot:
Welcome to SWANA Bank Today's Interest Rate is = 4.5%
Enter first name: Malleswara Rao Enter last name: Talla
Your account details are:
Name is: Malleswara Rao Talla
Account Number is 300
Current balance is $100.0
Interest Rate is = 4.5%
Monthly interest for this balance is $0.375
This account was created on Sun Oct 24 10:42:51 EDT 2010
Enter your choice (0: deposit, 1: withdraw, 2: quit): 0 Enter the amount in $300
Enter your choice (0: deposit, 1: withdraw, 2: quit): 0 Enter the amount in $100
Enter your choice (0: deposit, 1: withdraw, 2: quit): 1 Enter the amount in $50
Enter your choice (0: deposit, 1: withdraw, 2: quit): 1 Enter the amount in $25
Enter your choice (0: deposit, 1: withdraw, 2: quit): 2
New balance is $425.0 Good Bye!

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

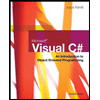
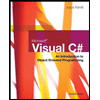