In my opinion, this is a 2D DP problem. dp(i, j) represents the minimum sum required to reach last index from index i and minimum jump allowed of size j. Let's say you are at index i in the array. Then you can either go to index i-j+1 or index i+j. So, int recur(int values[], int i, int j){ // base case. Here n is size of values array if(i==n-1) return 0; if(dp[i][j] != -1){ /* here -1 is taken as to mark never calculated state of dp. If the values [] array also contains negative values then you need to change it to something appropriate. */ return dp[i][j]; } int a = INT_MAX; int b = a; if(i>0 && (i-j+1)>=0) a = values [i-j + 1] + recur(values, i-j+1, j); if(i+j < n) b = values [i+j] + recur(values, i+j, j+1); return dp[i] [j] = min(a, b); } Time and space complexity O(n * n).
In my opinion, this is a 2D DP problem. dp(i, j) represents the minimum sum required to reach last index from index i and minimum jump allowed of size j. Let's say you are at index i in the array. Then you can either go to index i-j+1 or index i+j. So, int recur(int values[], int i, int j){ // base case. Here n is size of values array if(i==n-1) return 0; if(dp[i][j] != -1){ /* here -1 is taken as to mark never calculated state of dp. If the values [] array also contains negative values then you need to change it to something appropriate. */ return dp[i][j]; } int a = INT_MAX; int b = a; if(i>0 && (i-j+1)>=0) a = values [i-j + 1] + recur(values, i-j+1, j); if(i+j < n) b = values [i+j] + recur(values, i+j, j+1); return dp[i] [j] = min(a, b); } Time and space complexity O(n * n).
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can someone help me turn this in python code, please?
![Solution:
In my opinion, this is a 2D DP problem.
dp(i, j) represents the minimum sum required to reach last index from index i and minimum jump allowed of size j.
Let's say you are at index i in the array. Then you can either go to index i-j+1 or index i+j.
So,
int recur(int values[], int i, int j){
// base case. Here n is size of values array
if(i==n-1)
return 0;
if(dp[i] [j] != -1){
/* here -1 is taken as to mark never calculated state of dp.
If the values[] array also contains negative values then you need to change it to
something appropriate.
*/
return dp[i] [j];
}
int a = INT_MAX;
int b = a;
if(i>0 && (i-j+1)>=0)
a = values [i-j
if(i+j < n)
b = values [i+j] + recur(values, i+j, j+1);
return dp[i] [j] = min(a, b);
+ 1] + recur(values, i-j+1, j);
Time and space complexity O(n * n).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd104ea5e-59f4-47a3-a0d3-8c9f1ad3b57c%2Fa6123576-e079-40b1-813b-782a814623de%2Fwjm43v_processed.png&w=3840&q=75)
Transcribed Image Text:Solution:
In my opinion, this is a 2D DP problem.
dp(i, j) represents the minimum sum required to reach last index from index i and minimum jump allowed of size j.
Let's say you are at index i in the array. Then you can either go to index i-j+1 or index i+j.
So,
int recur(int values[], int i, int j){
// base case. Here n is size of values array
if(i==n-1)
return 0;
if(dp[i] [j] != -1){
/* here -1 is taken as to mark never calculated state of dp.
If the values[] array also contains negative values then you need to change it to
something appropriate.
*/
return dp[i] [j];
}
int a = INT_MAX;
int b = a;
if(i>0 && (i-j+1)>=0)
a = values [i-j
if(i+j < n)
b = values [i+j] + recur(values, i+j, j+1);
return dp[i] [j] = min(a, b);
+ 1] + recur(values, i-j+1, j);
Time and space complexity O(n * n).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
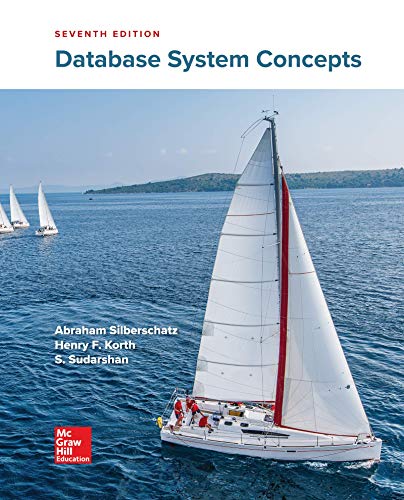
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
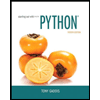
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
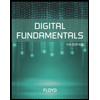
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
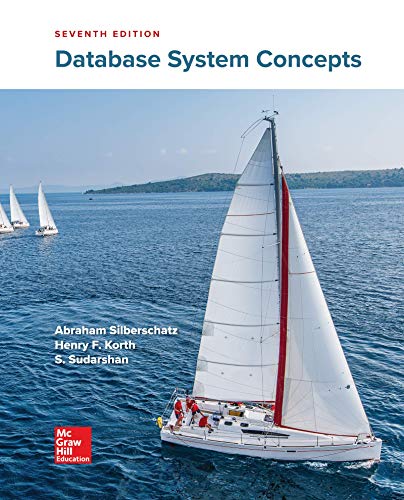
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
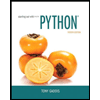
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
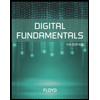
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
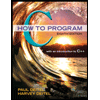
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
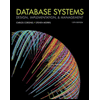
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
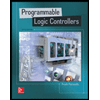
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education