In the following C program, identify what is wrong with code and correct it. This program reads addresses from an input.txt file and sorts the adresses by order of zip codes into an output.txt file. Please also see the following error codes, thank you main.c: #include "contacts.h" #include #include int main(int argc, char **argv) { size_t size; FILE *fp_in, *fp_out; errno_t err; if (argc != 3) { printf("Usage: %s infile outfile", argv[0]); return 0; } err = fopen_s(&fp_in, argv[1], "r"); if (err != 0) { perror("Failed opening file for reading"); } err = fopen_s(&fp_out, argv[2], "w+"); if (err != 0) { perror("Failed opening file for writing"); } contact **c = alloc_contacts(MAX_CONTACTS); size = get_contacts(c, fp_in); if (size == 0) perror("get_contacts failed"); sort_contacts(c, size); print_contacts(c, size, fp_out); free_alloc(c, size); if (fp_in) { err = fclose(fp_in); if (err != 0) { perror("File could not be closed"); } } if (fp_out) { err = fclose(fp_out); if (err != 0) { perror("File could not be closed"); } } return 1; } contacts.c: #include "contacts.h" #include #include /*Creates up to 50 structures*/ void create_data(user_data *users[]) { char new_user[100]; int i = 0; user_data *temp; /*Gets input until no info left*/ while (gets(new_user) != NULL && i < 50) { /*Dynamically Allocates new structure, then copies to array of pointers to structures*/ temp = (user_data*)malloc(sizeof(user_data)); /*Get info from file*/ strcpy(temp->name, new_user); gets(temp->address); gets(temp->city); gets(temp->zipcode); users[i++] = temp; }; users[i] = NULL; } /*Sorts data according the zipcode*/ void sort_data(user_data *users[]) { user_data *temp; /*Sorts smallest number to beginning*/ for (int i = 0; users[i] != NULL; i++) for (int j = i+1; users[j] != NULL; j++) if (strcmp(users[i]->zipcode, users[j]->zipcode) > 0) { temp = users[j]; users[j] = users[i]; users[i] = temp; } } /*Outputs data*/ void print_data(user_data *users[]) { for (int i = 0; users[i] != NULL; i++) printf("%s\n%s\n%s\n%s\n", users[i]->name, users[i]->address, users[i]->city, users[i]->zipcode); } /*Frees dynamically allocated memory*/ void free_mem(user_data *users[]) { for (int i = 0; users[i] != NULL; i++) free((void*)users[i]); } contacts.h: #define CONTACTS_H_INCLUDED typedef struct user_data{ char name[100]; char address[100]; char city[100]; char zipcode[100]; } user_data; void create_data(user_data *users[]); void sort_data(user_data *users[]); void print_data(user_data *users[]); void free_mem(user_data *users[]);
This program reads addresses from an input.txt file and sorts the adresses by order of zip codes into an output.txt file.
Please also see the following error codes, thank you
main.c:
#include "contacts.h"
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char **argv) {
size_t size;
FILE *fp_in, *fp_out;
errno_t err;
if (argc != 3) {
printf("Usage: %s infile outfile", argv[0]);
return 0;
}
err = fopen_s(&fp_in, argv[1], "r");
if (err != 0) {
perror("Failed opening file for reading");
}
err = fopen_s(&fp_out, argv[2], "w+");
if (err != 0) {
perror("Failed opening file for writing");
}
contact **c = alloc_contacts(MAX_CONTACTS);
size = get_contacts(c, fp_in);
if (size == 0)
perror("get_contacts failed");
sort_contacts(c, size);
print_contacts(c, size, fp_out);
free_alloc(c, size);
if (fp_in) {
err = fclose(fp_in);
if (err != 0) {
perror("File could not be closed");
}
}
if (fp_out) {
err = fclose(fp_out);
if (err != 0) {
perror("File could not be closed");
}
}
return 1;
}
contacts.c:
#include "contacts.h"
#include <stdio.h>
#include <stdlib.h>
/*Creates up to 50 structures*/
void create_data(user_data *users[])
{
char new_user[100];
int i = 0;
user_data *temp;
/*Gets input until no info left*/
while (gets(new_user) != NULL && i < 50)
{
/*Dynamically Allocates new structure, then copies to array of pointers to structures*/
temp = (user_data*)malloc(sizeof(user_data));
/*Get info from file*/
strcpy(temp->name, new_user);
gets(temp->address);
gets(temp->city);
gets(temp->zipcode);
users[i++] = temp;
};
users[i] = NULL;
}
/*Sorts data according the zipcode*/
void sort_data(user_data *users[])
{
user_data *temp;
/*Sorts smallest number to beginning*/
for (int i = 0; users[i] != NULL; i++)
for (int j = i+1; users[j] != NULL; j++)
if (strcmp(users[i]->zipcode, users[j]->zipcode) > 0)
{
temp = users[j];
users[j] = users[i];
users[i] = temp;
}
}
/*Outputs data*/
void print_data(user_data *users[])
{
for (int i = 0; users[i] != NULL; i++)
printf("%s\n%s\n%s\n%s\n", users[i]->name, users[i]->address, users[i]->city, users[i]->zipcode);
}
/*Frees dynamically allocated memory*/
void free_mem(user_data *users[])
{
for (int i = 0; users[i] != NULL; i++)
free((void*)users[i]);
}
contacts.h:
#define CONTACTS_H_INCLUDED
typedef struct user_data{
char name[100];
char address[100];
char city[100];
char zipcode[100];
} user_data;
void create_data(user_data *users[]);
void sort_data(user_data *users[]);
void print_data(user_data *users[]);
void free_mem(user_data *users[]);


Step by step
Solved in 2 steps

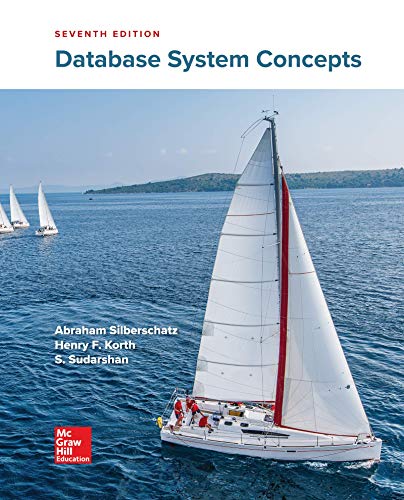
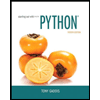
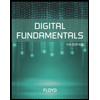
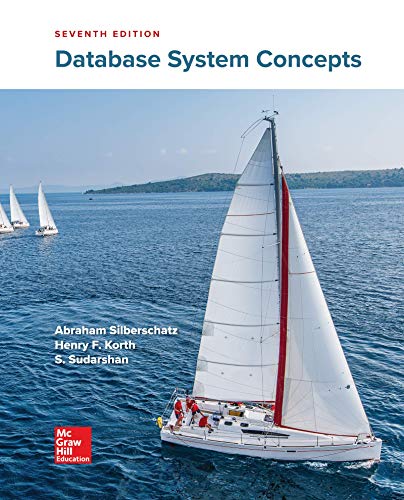
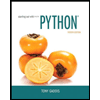
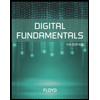
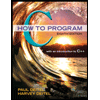
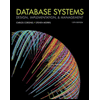
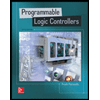