In this programming challenge, you will create a simple trivia game for two players. The program will work like this: • Read the contents of the trivia.txt file into an ArrayList . • Starting with player 1, each player gets a turn at answering one trivia question. (For instance, if there are 10 questions, 5 for each player.) When a question is displayed, four possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, he or she earns a point. • After answers have been selected for all of the questions, the program displays the number of points earned by each player and declares the player with the highest number of points the winner, same as the sample execution. You are to design a Question class to hold the data for a trivia question. The Question class should have String fields for the following data: • A trivia question • Possible answer 1 • Possible answer 2 • Possible answer 3 • Possible answer 4 • The number of the correct answer (1, 2, 3, or 4) The Question class should have appropriate constructor(s), accessor, and mutator methods. Also, you are to design a class, Player , to handle the players information. The program should create an ArrayList of Question objects, one for each trivia question. You must design a class, TriviaGame , to run a trivia game, and provide the outputs similar to the sample execution. In this program, you must read the questions, possible answers and the correct answer as lines of text from the text file, “trivia.txt”, provided and store them into an array of Question . To read from an external file, you must use a Scanner object, in the same way, you read inputs from the keyboard. The only difference is that you must indicate the text file name as an argument when you create the Scanner object. Below is a sample code snapshot for reading from a file: import java.io.IOException; import java.io.File; import java.util.Scanner; public class ReadFromFile { public static void main(String args[]) throws IOException { File file = new File("myFile.txt"); Scanner inputFile = new Scanner(file); String st1 = inputFile.nextLine(); String st2 = inputFile.nextLine(); inputFile.close(); } }.
In this
in java please

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 7 images

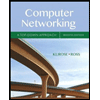
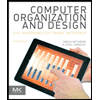
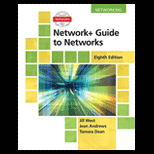
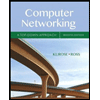
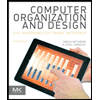
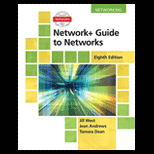
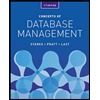
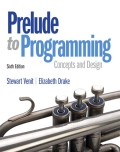
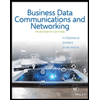