#include #include #include #include using namespace std; struct student { char name[30], id[10], addr[50], ph[15], dob[10], cls[5]; struct student * next; }; void addlist(struct student ** st, char name[], char id[], char addr[], char ph[], char dob[], char cls[]); struct student * search(struct student * st, char id[]); void delete1(struct student ** st, char id[]); void viewstudents(struct student * st); int main() { FILE * f; int ch, chh, flag = 1; char name[30], id[10], addr[50], ph[15], dob[10], cls[5]; struct student * start, * sr; start = NULL; f = fopen("Student.txt", "a+"); while (!fscanf(f, "%s\t%s\t%s\t%s\t%s\t%s\n", name, id, addr, ph, dob, cls)) { addlist( & start, name, id, addr, ph, dob, cls); } cout << "Welcome to A* High School Students Management System."; while (flag) { cout << "\nPlease select your choice:\n1.Adding a new student record"; cout << "\n2.Updating an existing student record\n3.Search a student record\n4.View students record\n5.Remove a student record"; cout << "\n6.Exit."; cout << "\nYour choice:"; cin >> ch; switch (ch) { case 1: cout << "Enter \tName:\tID\tAddress:\tPhone No.:\tD.O.B:\tClass:"; cin >> name >> id >> addr >> ph >> dob >> cls; addlist( & start, name, id, addr, ph, dob, cls); break; case 2: cout << "Enter ID"; cin >> id; sr = search(start, id); if (sr != NULL) { cout << "Address:\tPhone No.:"; cin >> sr -> addr >> sr -> ph; } else cout << "\nID not exist"; break; case 3: cout << "Enter ID"; cin >> id; sr = search(start, id); if (sr != NULL) cout << "\n" << sr -> name << "\t" << sr -> id << "\t" << sr -> addr << "\t" << sr -> ph << "\t" << sr -> dob << "\t" << sr -> cls; else cout << "\nID not exist"; break; case 4: cout << "1.View a student record\n2.View all students record\n3.Your choice: "; cin >> chh; if (chh == 1) { cout << "Enter ID"; cin >> id; sr = search(start, id); if (sr == NULL) cout << "\nIDnot exist"; else cout << sr -> name, sr -> id, id, sr -> addr, sr -> ph, sr -> dob, sr -> cls; } else if (chh == 2) viewstudents(start); break; case 5: cout << "Enter ID"; cin >> id; delete1( & start, id); break; case 6: f = fopen("Student.txt", "w"); while (start != NULL) { fprintf(f, "%s\t%s\t%s\t%s\t%s\t%s\n", start -> name, start -> id, start -> addr, start -> ph, start -> dob, start -> cls); start = start -> next; } flag = 0; break; } } return 0; } void addlist(struct student ** st, char name[], char id[], char addr[], char ph[], char dob[], char cls[]) { struct student * temp, * new_node; if ( * st == NULL) { temp = new struct student; strcpy(temp -> name, name); strcpy(temp -> id, id); strcpy(temp -> addr, addr); strcpy(temp -> ph, ph); strcpy(temp -> dob, dob); strcpy(temp -> cls, cls); temp -> next = NULL; * st = temp; } else { temp = * st; while (temp -> next != NULL) temp = temp -> next; new_node = new struct student; strcpy(new_node -> name, name); strcpy(new_node -> id, id); strcpy(new_node -> addr, addr); strcpy(new_node -> ph, ph); strcpy(new_node -> dob, dob); strcpy(new_node -> cls, cls); new_node -> next = NULL; temp -> next = new_node; } } struct student * search(struct student * st, char id[]) { while (st != NULL) { if (strcmp(st -> id, id) == 0) return st; st = st -> next; } return NULL; } void delete1(struct student ** st, char id[]) { struct student * temp, * prev; temp = * st; while (temp != NULL) { if (strcmp(temp -> id, id) == 0) { if (temp == * st) * st = temp -> next; else prev -> next = temp -> next; free(temp); return; } else { prev = temp; temp = temp -> next; } } cout << "\nID not exist"; } void viewstudents(struct student * stt) { struct student * st = stt, * temp; char n[10]; int i = 1; if (stt == NULL) cout << "\nNo data"; else { while (st != NULL) //sorting { temp = st -> next; while (temp != NULL) { if (strcmp(st -> id, temp -> id) > 0) { strcpy(n, st -> name); strcpy(st -> name, temp -> name); strcpy(temp -> name, n); strcpy(n, st -> id); strcpy(st -> id, temp -> id); strcpy(temp -> id, n); strcpy(n, st -> addr); strcpy(st -> addr, temp -> addr); strcpy(temp -> addr, n); strcpy(n, st -> ph); strcpy(st -> ph, temp -> ph); strcpy(temp -> ph, n); strcpy(n, st -> dob); strcpy(st -> dob, temp -> dob); strcpy(temp -> dob, n); strcpy(n, st -> cls); strcpy(st -> cls, temp -> cls); strcpy(temp -> cls, n); } temp = temp -> next; } st = st -> next; } st = stt; cout << "\nNo.\tName\tID\tAddress\tPhone No.\tD.O.B\tClass"; while (st != NULL) { cout << "\n" << i << "\t" << st -> name << "\t" << st -> id << "\t" << st -> addr << "\t" << st -> ph << "\t" << st -> dob << "\t" << st -> cls; st = st -> next; i++; } } }
#include <iostream>
#include<malloc.h>
#include<fstream>
#include<string.h>
using namespace std;
struct student {
char name[30], id[10], addr[50], ph[15], dob[10], cls[5];
struct student * next;
};
void addlist(struct student ** st, char name[], char id[], char addr[], char ph[], char dob[], char cls[]);
struct student * search(struct student * st, char id[]);
void delete1(struct student ** st, char id[]);
void viewstudents(struct student * st);
int main() {
FILE * f;
int ch, chh, flag = 1;
char name[30], id[10], addr[50], ph[15], dob[10], cls[5];
struct student * start, * sr;
start = NULL;
f = fopen("Student.txt", "a+");
while (!fscanf(f, "%s\t%s\t%s\t%s\t%s\t%s\n", name, id, addr, ph, dob, cls)) {
addlist( & start, name, id, addr, ph, dob, cls);
}
cout << "Welcome to A* High School Students Management System.";
while (flag) {
cout << "\nPlease select your choice:\n1.Adding a new student record";
cout << "\n2.Updating an existing student record\n3.Search a student record\n4.View students record\n5.Remove a student record";
cout << "\n6.Exit.";
cout << "\nYour choice:";
cin >> ch;
switch (ch) {
case 1:
cout << "Enter \tName:\tID\tAddress:\tPhone No.:\tD.O.B:\tClass:";
cin >> name >> id >> addr >> ph >> dob >> cls;
addlist( & start, name, id, addr, ph, dob, cls);
break;
case 2:
cout << "Enter ID";
cin >> id;
sr = search(start, id);
if (sr != NULL) {
cout << "Address:\tPhone No.:";
cin >> sr -> addr >> sr -> ph;
} else
cout << "\nID not exist";
break;
case 3:
cout << "Enter ID";
cin >> id;
sr = search(start, id);
if (sr != NULL)
cout << "\n" << sr -> name << "\t" << sr -> id << "\t" << sr -> addr << "\t" << sr -> ph << "\t" << sr -> dob << "\t" << sr -> cls;
else
cout << "\nID not exist";
break;
case 4:
cout << "1.View a student record\n2.View all students record\n3.Your choice: ";
cin >> chh;
if (chh == 1) {
cout << "Enter ID";
cin >> id;
sr = search(start, id);
if (sr == NULL)
cout << "\nIDnot exist";
else
cout << sr -> name, sr -> id, id, sr -> addr, sr -> ph, sr -> dob, sr -> cls;
} else if (chh == 2)
viewstudents(start);
break;
case 5:
cout << "Enter ID";
cin >> id;
delete1( & start, id);
break;
case 6:
f = fopen("Student.txt", "w");
while (start != NULL) {
fprintf(f, "%s\t%s\t%s\t%s\t%s\t%s\n", start -> name, start -> id, start -> addr, start -> ph, start -> dob, start -> cls);
start = start -> next;
}
flag = 0;
break;
}
}
return 0;
}
void addlist(struct student ** st, char name[], char id[], char addr[], char ph[], char dob[], char cls[]) {
struct student * temp, * new_node;
if ( * st == NULL) {
temp = new struct student;
strcpy(temp -> name, name);
strcpy(temp -> id, id);
strcpy(temp -> addr, addr);
strcpy(temp -> ph, ph);
strcpy(temp -> dob, dob);
strcpy(temp -> cls, cls);
temp -> next = NULL;
* st = temp;
} else {
temp = * st;
while (temp -> next != NULL)
temp = temp -> next;
new_node = new struct student;
strcpy(new_node -> name, name);
strcpy(new_node -> id, id);
strcpy(new_node -> addr, addr);
strcpy(new_node -> ph, ph);
strcpy(new_node -> dob, dob);
strcpy(new_node -> cls, cls);
new_node -> next = NULL;
temp -> next = new_node;
}
}
struct student * search(struct student * st, char id[]) {
while (st != NULL) {
if (strcmp(st -> id, id) == 0)
return st;
st = st -> next;
}
return NULL;
}
void delete1(struct student ** st, char id[]) {
struct student * temp, * prev;
temp = * st;
while (temp != NULL) {
if (strcmp(temp -> id, id) == 0) {
if (temp == * st)
*
st = temp -> next;
else
prev -> next = temp -> next;
free(temp);
return;
} else {
prev = temp;
temp = temp -> next;
}
}
cout << "\nID not exist";
}
void viewstudents(struct student * stt) {
struct student * st = stt, * temp;
char n[10];
int i = 1;
if (stt == NULL)
cout << "\nNo data";
else {
while (st != NULL) //sorting
{
temp = st -> next;
while (temp != NULL) {
if (strcmp(st -> id, temp -> id) > 0) {
strcpy(n, st -> name);
strcpy(st -> name, temp -> name);
strcpy(temp -> name, n);
strcpy(n, st -> id);
strcpy(st -> id, temp -> id);
strcpy(temp -> id, n);
strcpy(n, st -> addr);
strcpy(st -> addr, temp -> addr);
strcpy(temp -> addr, n);
strcpy(n, st -> ph);
strcpy(st -> ph, temp -> ph);
strcpy(temp -> ph, n);
strcpy(n, st -> dob);
strcpy(st -> dob, temp -> dob);
strcpy(temp -> dob, n);
strcpy(n, st -> cls);
strcpy(st -> cls, temp -> cls);
strcpy(temp -> cls, n);
}
temp = temp -> next;
}
st = st -> next;
}
st = stt;
cout << "\nNo.\tName\tID\tAddress\tPhone No.\tD.O.B\tClass";
while (st != NULL) {
cout << "\n" << i << "\t" << st -> name << "\t" << st -> id << "\t" << st -> addr << "\t" << st -> ph << "\t" << st -> dob << "\t" << st -> cls;
st = st -> next;
i++;
}
}
}


Step by step
Solved in 2 steps

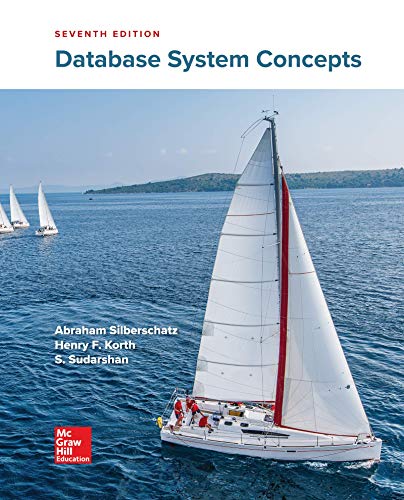
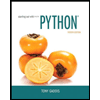
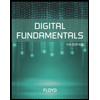
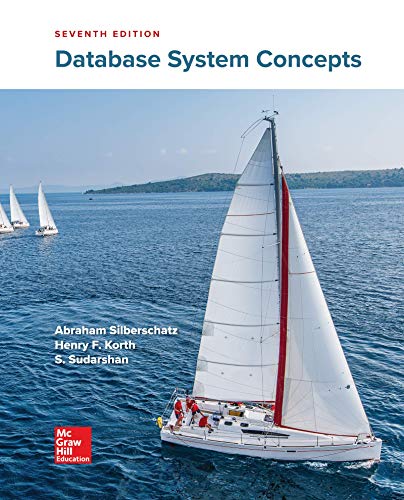
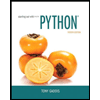
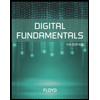
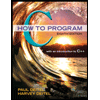
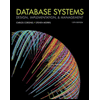
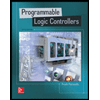