include using namespace std; class Vehicle // superclass { private: int x = 7; // only class vehicle objects can access protected: int y = 3; // only class vehicle and kid objects can access public: int get_x() // Accessed by all { return x; } }; class Car : public Vehicle // subclass or child of class vehicle AND Parent of Corvette class { private: int z = 4; // only a class car object cna access protected: int w = 2; int get_y() { return y; } int get_z() { return z; } public: int get_z3() { return z; } }; class Corvette : public Car // subclass of car class { private: int d = 8; public: int get_d() { return d; } int get_z2() { return get_z(); } }; class Mustang : public Car // Hierarchical Mustang and Corvette { private: int u = 99; public: int get_u() { return u; } }; int main() { Vehicle vehicle; //construct object of class Vehicle cout << "Private x data from class vehicle object: " << vehicle.get_x() << endl; Car car; //construct Car object cout << "The private z data of object car is: " << car.get_z3() << endl; Corvette corvette; // construct corvette object cout << "The corvette objecthas this info: z = " << corvette.get_z2() << " x= " << corvette.get_x() << " d = " << corvette.get_d() << endl; return 0; } Using C++ Similar to the code above Create classes using only 1 file (and draw the structure in your comments at the top of your program) For each class create these features: A private variable that can only be accessed by that class. A protected variable that can only be accessed by that class or children of that class. Getter and Setter functions for accessing the private data of the class. A Constructor th
#include<iostream>
using namespace std;
class Vehicle // superclass
{
private:
int x = 7; // only class vehicle objects can access
protected:
int y = 3; // only class vehicle and kid objects can access
public:
int get_x() // Accessed by all
{
return x;
}
};
class Car : public Vehicle // subclass or child of class vehicle AND Parent of Corvette class
{
private:
int z = 4; // only a class car object cna access
protected:
int w = 2;
int get_y()
{
return y;
}
int get_z()
{
return z;
}
public:
int get_z3()
{
return z;
}
};
class Corvette : public Car // subclass of car class
{
private:
int d = 8;
public:
int get_d()
{
return d;
}
int get_z2()
{
return get_z();
}
};
class Mustang : public Car // Hierarchical Mustang and Corvette
{
private:
int u = 99;
public:
int get_u()
{
return u;
}
};
int main()
{
Vehicle vehicle; //construct object of class Vehicle
cout << "Private x data from class vehicle object: " << vehicle.get_x() << endl;
Car car; //construct Car object
cout << "The private z data of object car is: " << car.get_z3() << endl;
Corvette corvette; // construct corvette object
cout << "The corvette objecthas this info: z = " << corvette.get_z2()
<< " x= " << corvette.get_x() << " d = " << corvette.get_d()
<< endl;
return 0;
}
Using C++
- Similar to the code above
- Create classes using only 1 file (and draw the structure in your comments at the top of your program)
-
- For each class create these features:
- A private variable that can only be accessed by that class.
- A protected variable that can only be accessed by that class or children of that class.
- Getter and Setter functions for accessing the private data of the class.
- A Constructor that outputs the name of the class whenever it is used to instantiate or construct a class object.
- For each class create these features:
Example: "Class A object constructed."
- Comments that describe exactly how the class is structured and what are it's features (superclass, subclass, overloading, etc).
- Add any variables or functions you deem necessary, but be sure to describe how they work using comments and the couts as required below.
-
- Multi-level Classes consisting of 3 classes (A, B, and C, with A as top superclass).
- In class A, add a set of public overloading functions for printing out different data types.
- Multi-level Classes consisting of 3 classes (A, B, and C, with A as top superclass).
Example: print(int param), print(double param), and print(string param)
-
- Multiple Classes consisting of 2 new classes (C and D as superclasses and E as subclass).
- Class C is the same class as in the Multi-level classes above.
- Multiple Classes consisting of 2 new classes (C and D as superclasses and E as subclass).
-
- Hierarachical Classes consisting of 2 new classes (D as the superclass with E, F, and G as subclasses).
- Classes D and E are the same classes as described in the Multiple Classes above
- Hierarachical Classes consisting of 2 new classes (D as the superclass with E, F, and G as subclasses).
-
- Function main() should show off all the features of the classes including:
- Use "cout" to describe exactly what is going on so that I don't have to guess and can easily grade it. If I have to hunt for features, the grade will be lower.
- Examples:
- "Object a has been instantiated from class A."
- "Observe how function overloading works in a class A object..."
- "Observe how function overloading works in a class B object, a child of class A..."
- "See how private_x can be changed directly..."
- "See how private_x can be changed by object g..."
- "Object c has been instantiated."
- "Object a has been instantiated from class A."
- Examples:
- Use "cout" to describe exactly what is going on so that I don't have to guess and can easily grade it. If I have to hunt for features, the grade will be lower.
- Function main() should show off all the features of the classes including:

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

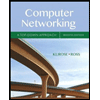
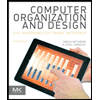
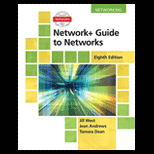
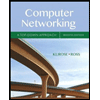
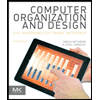
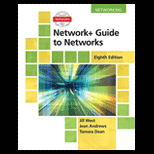
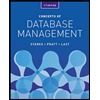
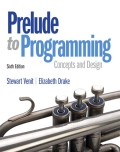
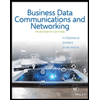