Introduction In this lab, we will be creating a graphical user interface (GUI) to allow the user to select a button that will change the color of the center panel and radio buttons that will change the color of the text in the center panel. We will need to use a variety of Swing components to accomplish this task. We will build two panels, a top panel containing three buttons and a bottom panel containing three radio buttons. Layouts will be used to add these panels to the window in the desired positions. A label with instructions will also be added to the window. Listeners will be employed to handle the events desired by the user. Task #1 Creating a GUI Import the required Java libraries. Create a class called ColorFactory that inherits from JFrame. Create named constants for a width of 500 and height of 300 for the frame. Write a default constructor that does the following: Set the title of the window to Color Factory. Set the size of the window using the constants. Specify what happens when the close button is clicked. Get the content pane of the JFrame and set the layout manager to border layout. Call the method to build the top panel (to be written as directed below). Add the panel to the north part of the content pane. Call the method to build the bottom panel (to be written as directed below). Add this panel to the south part of the content pane. Create a label that contains the message “Top buttons change the panel color and bottom radio buttons change the text color.” Add this label to the center part of the content pane. Task #2 Writing Private methods Write a private method that builds the top panel as follows: Create a panel that contains three buttons, red, orange, and yellow. Use flow layout for the panel and set the background to be white. The buttons should be labeled with the color name and also appear in that color. Set the action command of each button to be the first letter of the color name. Add a button listener that implements an action listener for each button. Create a bottom panel in the same way as the top panel above, but use radio buttons with the colors green, blue, and cyan. Task #3 Writing Inner Classes Write a private inner class called ButtonListener that implements ActionListener. It should contain an actionPerformed method to handle the button events. This event handler will handle all button events, so you must get the action command of the event and write a decision structure to determine which color to set the background of the content pane. Write another private inner class called RadioButtonListener, similar to ButtonListener. It will handle all radio button events, so you will need to check the source of the event and write a decision structure to determine which color should be used for the text of the message. Task #4 Running the GUI Program 1. Write a main method that declares and creates one instance of a ColorFactory, then use the setVisible method to show it on the screen.
Introduction
In this lab, we will be creating a graphical user interface (GUI) to allow the user to select a button that will change the color of the center panel and radio buttons that will change the color of the text in the center panel. We will need to use a variety of Swing components to accomplish this task.
We will build two panels, a top panel containing three buttons and a bottom panel containing three radio buttons. Layouts will be used to add these panels to the window in the desired positions. A label with instructions will also be added to the window. Listeners will be employed to handle the events desired by the user.
Task #1 Creating a GUI
-
Import the required Java libraries.
-
Create a class called ColorFactory that inherits from JFrame.
-
Create named constants for a width of 500 and height of 300 for the frame.
-
Write a default constructor that does the following:
-
Set the title of the window to Color Factory.
-
Set the size of the window using the constants.
-
Specify what happens when the close button is clicked.
-
Get the content pane of the JFrame and set the layout manager to border
layout.
-
Call the method to build the top panel (to be written as directed below).
-
Add the panel to the north part of the content pane.
-
Call the method to build the bottom panel (to be written as directed below).
-
Add this panel to the south part of the content pane.
-
Create a label that contains the message “Top buttons change the panel color
and bottom radio buttons change the text color.”
-
Add this label to the center part of the content pane.
-
Task #2 Writing Private methods
-
Write a private method that builds the top panel as follows:
-
Create a panel that contains three buttons, red, orange, and yellow.
-
Use flow layout for the panel and set the background to be white.
-
The buttons should be labeled with the color name and also appear in that
color.
-
Set the action command of each button to be the first letter of the color name.
-
Add a button listener that implements an action listener for each button.
-
-
Create a bottom panel in the same way as the top panel above, but use radio buttons with the colors green, blue, and cyan.
Task #3 Writing Inner Classes
-
Write a private inner class called ButtonListener that implements ActionListener. It should contain an actionPerformed method to handle the button events. This event handler will handle all button events, so you must get the action command of the event and write a decision structure to determine which color to set the background of the content pane.
-
Write another private inner class called RadioButtonListener, similar to ButtonListener. It will handle all radio button events, so you will need to check the source of the event and write a decision structure to determine which color should be used for the text of the message.
Task #4 Running the GUI Program
1. Write a main method that declares and creates one instance of a ColorFactory, then use the setVisible method to show it on the screen.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

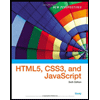