(Java) CHECK IF FINAL OUTPUT MATCHES SAMPLE OUTPUT! Make the 2 following classes: Employee.java EmployeeTest.java Copy and paste the below starter code into Employee.java: /** * Employee.java extends Person.java * @author * CIS 36B, Activity 11.1 */ public class Employee extends Person { private static int numEmployees = 0; private double salary; private String title; /** * Default constructor for the * Employee class. Calls the * default constructor of the * superclass and also initializes * salary and title to default * values */ public Employee() { } /** * Multi-argument constructor for the * Employee class. Calls the * multi-argument constructor of the * superclass and also initializes * salary and title to the * specified values */ public Employee(String name, int age, String gender, Address a, double salary, String title) { } /** * Returns the annual salary * @return the salary */ public double getSalary() { return -1.0; } /** * Returns the title (position) * of the employee in the company * @return the title */ public String getTitle() { return ""; } /** * Returns the total number of employees * @return the number of employees */ public static int getNumEmployees() { return -1; } /** * Updates the salary with a new value * @param salary the new salary */ public void setSalary(double salary) { } /** * Updates the title of the employee * @param title the new title */ public void setTitle(String title) { } /** * Increments numEmployees */ public static void updateNumEmployees() { } /** * To String method for an employee * Calls the toString method of the * superclass and also appends the title * and salary information */ @Override public String toString() { return ""; } } Notice that this class is a subclass of Person (it extends Person). Your job is to implement the method bodies of the above methods, as described in the method javadoc comments When you have implemented all methods, copy and paste the below code into EmployeeTest.java, and make sure your output works as shown. /** * EmployeeTest.java * @author * CIS 36B, Activity 11.1 */ import java.util.Scanner; public class EmployeeTest { public static void main(String[] args) { Scanner input = new Scanner(System.in); String name, gender, street, title; int age, number; double salary; Employee employee; System.out.print("Welcome!\n\nEnter your name: "); name = input.nextLine(); System.out.print("Enter your age: "); age = input.nextInt(); System.out.print("Enter your gender: "); gender = input.next(); System.out.print("Enter the street number of your address: "); number = input.nextInt(); System.out.print("Enter the name of your street: "); input.nextLine(); street = input.nextLine(); Address address = new Address(number, street); System.out.print("Enter your title in the company: "); title = input.nextLine(); System.out.print("Enter your annual salary: "); salary = input.nextDouble(); employee = new Employee(name, age, gender, address, salary, title); Employee.updateNumEmployees(); System.out.println("\nYour Summary:\n" + employee); input.close(); } } When your program works as shown in the sample output, upload Employee.java to Canvas. Sample Output: Welcome! Enter your name: Rajya Chahal Enter your age: 32 Enter your gender: F Enter the street number of your address: 21899 Enter the name of your street: Summit Rd Enter your title in the company: Vice President of Operations Enter your annual salary: 185000 Your Summary: Name: Rajya Chahal Age: 32 Gender: F Address: 21899 Summit Rd Salary: $185000.0 Title: Vice President of Operations
(Java) CHECK IF FINAL OUTPUT MATCHES SAMPLE OUTPUT! Make the 2 following classes: Employee.java EmployeeTest.java Copy and paste the below starter code into Employee.java: /** * Employee.java extends Person.java * @author * CIS 36B, Activity 11.1 */ public class Employee extends Person { private static int numEmployees = 0; private double salary; private String title; /** * Default constructor for the * Employee class. Calls the * default constructor of the * superclass and also initializes * salary and title to default * values */ public Employee() { } /** * Multi-argument constructor for the * Employee class. Calls the * multi-argument constructor of the * superclass and also initializes * salary and title to the * specified values */ public Employee(String name, int age, String gender, Address a, double salary, String title) { } /** * Returns the annual salary * @return the salary */ public double getSalary() { return -1.0; } /** * Returns the title (position) * of the employee in the company * @return the title */ public String getTitle() { return ""; } /** * Returns the total number of employees * @return the number of employees */ public static int getNumEmployees() { return -1; } /** * Updates the salary with a new value * @param salary the new salary */ public void setSalary(double salary) { } /** * Updates the title of the employee * @param title the new title */ public void setTitle(String title) { } /** * Increments numEmployees */ public static void updateNumEmployees() { } /** * To String method for an employee * Calls the toString method of the * superclass and also appends the title * and salary information */ @Override public String toString() { return ""; } } Notice that this class is a subclass of Person (it extends Person). Your job is to implement the method bodies of the above methods, as described in the method javadoc comments When you have implemented all methods, copy and paste the below code into EmployeeTest.java, and make sure your output works as shown. /** * EmployeeTest.java * @author * CIS 36B, Activity 11.1 */ import java.util.Scanner; public class EmployeeTest { public static void main(String[] args) { Scanner input = new Scanner(System.in); String name, gender, street, title; int age, number; double salary; Employee employee; System.out.print("Welcome!\n\nEnter your name: "); name = input.nextLine(); System.out.print("Enter your age: "); age = input.nextInt(); System.out.print("Enter your gender: "); gender = input.next(); System.out.print("Enter the street number of your address: "); number = input.nextInt(); System.out.print("Enter the name of your street: "); input.nextLine(); street = input.nextLine(); Address address = new Address(number, street); System.out.print("Enter your title in the company: "); title = input.nextLine(); System.out.print("Enter your annual salary: "); salary = input.nextDouble(); employee = new Employee(name, age, gender, address, salary, title); Employee.updateNumEmployees(); System.out.println("\nYour Summary:\n" + employee); input.close(); } } When your program works as shown in the sample output, upload Employee.java to Canvas. Sample Output: Welcome! Enter your name: Rajya Chahal Enter your age: 32 Enter your gender: F Enter the street number of your address: 21899 Enter the name of your street: Summit Rd Enter your title in the company: Vice President of Operations Enter your annual salary: 185000 Your Summary: Name: Rajya Chahal Age: 32 Gender: F Address: 21899 Summit Rd Salary: $185000.0 Title: Vice President of Operations
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
(Java)
CHECK IF FINAL OUTPUT MATCHES SAMPLE OUTPUT!
Make the 2 following classes:
Employee.java
EmployeeTest.java
- Copy and paste the below starter code into Employee.java:
/**
* Employee.java extends Person.java
* @author
* CIS 36B, Activity 11.1
*/
public class Employee extends Person {
private static int numEmployees = 0;
private double salary;
private String title;
/**
* Default constructor for the
* Employee class. Calls the
* default constructor of the
* superclass and also initializes
* salary and title to default
* values
*/
public Employee() {
}
/**
* Multi-argument constructor for the
* Employee class. Calls the
* multi-argument constructor of the
* superclass and also initializes
* salary and title to the
* specified values
*/
public Employee(String name, int age, String gender, Address a, double salary, String title) {
}
/**
* Returns the annual salary
* @return the salary
*/
public double getSalary() {
return -1.0;
}
/**
* Returns the title (position)
* of the employee in the company
* @return the title
*/
public String getTitle() {
return "";
}
/**
* Returns the total number of employees
* @return the number of employees
*/
public static int getNumEmployees() {
return -1;
}
/**
* Updates the salary with a new value
* @param salary the new salary
*/
public void setSalary(double salary) {
}
/**
* Updates the title of the employee
* @param title the new title
*/
public void setTitle(String title) {
}
/**
* Increments numEmployees
*/
public static void updateNumEmployees() {
}
/**
* To String method for an employee
* Calls the toString method of the
* superclass and also appends the title
* and salary information
*/
@Override public String toString() {
return "";
}
}
* Employee.java extends Person.java
* @author
* CIS 36B, Activity 11.1
*/
public class Employee extends Person {
private static int numEmployees = 0;
private double salary;
private String title;
/**
* Default constructor for the
* Employee class. Calls the
* default constructor of the
* superclass and also initializes
* salary and title to default
* values
*/
public Employee() {
}
/**
* Multi-argument constructor for the
* Employee class. Calls the
* multi-argument constructor of the
* superclass and also initializes
* salary and title to the
* specified values
*/
public Employee(String name, int age, String gender, Address a, double salary, String title) {
}
/**
* Returns the annual salary
* @return the salary
*/
public double getSalary() {
return -1.0;
}
/**
* Returns the title (position)
* of the employee in the company
* @return the title
*/
public String getTitle() {
return "";
}
/**
* Returns the total number of employees
* @return the number of employees
*/
public static int getNumEmployees() {
return -1;
}
/**
* Updates the salary with a new value
* @param salary the new salary
*/
public void setSalary(double salary) {
}
/**
* Updates the title of the employee
* @param title the new title
*/
public void setTitle(String title) {
}
/**
* Increments numEmployees
*/
public static void updateNumEmployees() {
}
/**
* To String method for an employee
* Calls the toString method of the
* superclass and also appends the title
* and salary information
*/
@Override public String toString() {
return "";
}
}
- Notice that this class is a subclass of Person (it extends Person).
- Your job is to implement the method bodies of the above methods, as described in the method javadoc comments
- When you have implemented all methods, copy and paste the below code into EmployeeTest.java, and make sure your output works as shown.
/**
* EmployeeTest.java
* @author
* CIS 36B, Activity 11.1
*/
import java.util.Scanner;
public class EmployeeTest {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
String name, gender, street, title;
int age, number;
double salary;
Employee employee;
System.out.print("Welcome!\n\nEnter your name: ");
name = input.nextLine();
System.out.print("Enter your age: ");
age = input.nextInt();
System.out.print("Enter your gender: ");
gender = input.next();
System.out.print("Enter the street number of your address: ");
number = input.nextInt();
System.out.print("Enter the name of your street: ");
input.nextLine();
street = input.nextLine();
Address address = new Address(number, street);
System.out.print("Enter your title in the company: ");
title = input.nextLine();
System.out.print("Enter your annual salary: ");
salary = input.nextDouble();
employee = new Employee(name, age, gender, address, salary, title);
Employee.updateNumEmployees();
System.out.println("\nYour Summary:\n" + employee);
input.close();
}
}
* EmployeeTest.java
* @author
* CIS 36B, Activity 11.1
*/
import java.util.Scanner;
public class EmployeeTest {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
String name, gender, street, title;
int age, number;
double salary;
Employee employee;
System.out.print("Welcome!\n\nEnter your name: ");
name = input.nextLine();
System.out.print("Enter your age: ");
age = input.nextInt();
System.out.print("Enter your gender: ");
gender = input.next();
System.out.print("Enter the street number of your address: ");
number = input.nextInt();
System.out.print("Enter the name of your street: ");
input.nextLine();
street = input.nextLine();
Address address = new Address(number, street);
System.out.print("Enter your title in the company: ");
title = input.nextLine();
System.out.print("Enter your annual salary: ");
salary = input.nextDouble();
employee = new Employee(name, age, gender, address, salary, title);
Employee.updateNumEmployees();
System.out.println("\nYour Summary:\n" + employee);
input.close();
}
}
- When your program works as shown in the sample output, upload Employee.java to Canvas.
Sample Output:
Welcome!
Enter your name: Rajya Chahal
Enter your age: 32
Enter your gender: F
Enter the street number of your address: 21899
Enter the name of your street: Summit Rd
Enter your title in the company: Vice President of Operations
Enter your annual salary: 185000
Your Summary:
Name: Rajya Chahal
Age: 32
Gender: F
Address: 21899 Summit Rd
Salary: $185000.0
Title: Vice President of Operations
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
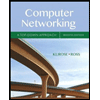
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
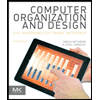
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
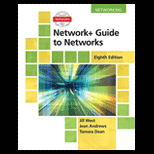
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
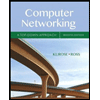
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
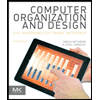
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
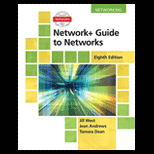
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
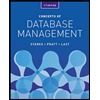
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
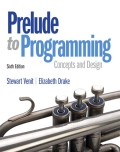
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
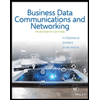
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY