JAVA PROGRAM Chapter 7. PC #16. 2D Array Operations with Additional Requirements Write a program that creates an ArrayList of ArrayList of Doubles. The program should ask the user to enter the filename from the keyboard and validate the file for existence. Once the file is verified, the program should load the two-dimensional ArrayList with test data from the file. Be careful, as some of the files will contain rows with different number of elements. The program should work regardless whether the input data is perfectly rectangular or ragged. The program should have the following methods: • main. Main entry point for the program. • getRowSubtotal. This method should accept a two-dimensional ArrayList as its first argument and an integer as its second argument. The second argument should be the index of the row in the ArrayList. The method should return the subtotal of the values in the specified row. • getColSubtotal. This method should accept a two-dimensional ArrayList as its first argument and an integer as its second argument. The second argument should be the index of the column in the ArrayList. The method should return the subtotal of the values in the specified column. If any of the rows has a size that is less than the column number, then that row should be skipped when calculating the column subtotal. • getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array. Demonstrate each of the methods in this program. Input1.txt 1 2 3 4 5 6 7 8 9 Input2.txt 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 Input3.txt 555.66 333.44 123.45 555.66 333.44 123.45 778.88 900.00 123.12 778.88 900.00 123.12 234.23 567.98 12.12 234.23 567.98 12.12 Input4.txt 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 Input5.txt 555.66 333.44 123.45 555.66 333.44 123.45 778.88 900.00 123.12 778.88 900.00 234.23 567.98 12.12 234.23 Input6.txt 555.66 333.44 123.45 555.66 778.88 900.00 123.12 778.88 900.00 234.23 567.98 12.12 234.23 567.98 12.12 Input7.txt 555.66 333.44 123.45 755.23 778.88 900.00 123.12 234.23 567.98 555.66 778.88 900.00 123.12 999.99 234.23 567.98 12.12 555.66 333.44 778.88 234.23 567.98 12.12 987.65 432.11 820.17 Input8.txt 555.66 778.88 900.00 234.23 567.98 333.44 555.66 123.45 755.23 888.88 778.88 900.00 123.12 999.99 777.77 778.88 900.00 123.12 999.99 777.77 666.66 778.88 900.00 123.12 999.99 777.77 555.55 444.44 778.88 900.00 123.12 999.99 777.77 333.33 222.22 111.11 est Case 1 Please enter the file name or type QUIT to exit:\n quitENTER Test Case 2 Please enter the file name or type QUIT to exit:\n QUITENTER Test Case 3 Please enter the file name or type QUIT to exit:\n qUiTENTER Test Case 4 Please enter the file name or type QUIT to exit:\n QuItENTER Test Case 5 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n quitENTER Test Case 6 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n QUITENTER Test Case 7 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n QuItENTER Test Case 8 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n qUiTENTER Test Case 9 Please enter the file name or type QUIT to exit:\n input1.txtENTER Row 0 Length: 3, Subtotal: 6.000\n Row 1 Length: 3, Subtotal: 15.000\n Row 2 Length: 3, Subtotal: 24.000\n Column 0 Height: 3, Subtotal: 12.000\n Column 1 Height: 3, Subtotal: 15.000\n Column 2 Height: 3, Subtotal: 18.000\n Array Elements: 9, Total: 45.000\n Test Case 10 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n input2.txtENTER Row 0 Length: 3, Subtotal: 1012.550\n Row 1 Length: 3, Subtotal: 1802.000\n Row 2 Length: 3, Subtotal: 814.330\n Column 0 Height: 3, Subtotal: 1568.770\n Column 1 Height: 3, Subtotal: 1801.420\n Column 2 Height: 3, Subtotal: 258.690\n Array Elements: 9, Total: 3628.880\n
JAVA PROGRAM Chapter 7. PC #16. 2D Array Operations with Additional Requirements Write a program that creates an ArrayList of ArrayList of Doubles. The program should ask the user to enter the filename from the keyboard and validate the file for existence. Once the file is verified, the program should load the two-dimensional ArrayList with test data from the file. Be careful, as some of the files will contain rows with different number of elements. The program should work regardless whether the input data is perfectly rectangular or ragged. The program should have the following methods: • main. Main entry point for the program. • getRowSubtotal. This method should accept a two-dimensional ArrayList as its first argument and an integer as its second argument. The second argument should be the index of the row in the ArrayList. The method should return the subtotal of the values in the specified row. • getColSubtotal. This method should accept a two-dimensional ArrayList as its first argument and an integer as its second argument. The second argument should be the index of the column in the ArrayList. The method should return the subtotal of the values in the specified column. If any of the rows has a size that is less than the column number, then that row should be skipped when calculating the column subtotal. • getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array. Demonstrate each of the methods in this program. Input1.txt 1 2 3 4 5 6 7 8 9 Input2.txt 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 Input3.txt 555.66 333.44 123.45 555.66 333.44 123.45 778.88 900.00 123.12 778.88 900.00 123.12 234.23 567.98 12.12 234.23 567.98 12.12 Input4.txt 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 555.66 333.44 123.45 778.88 900.00 123.12 234.23 567.98 12.12 Input5.txt 555.66 333.44 123.45 555.66 333.44 123.45 778.88 900.00 123.12 778.88 900.00 234.23 567.98 12.12 234.23 Input6.txt 555.66 333.44 123.45 555.66 778.88 900.00 123.12 778.88 900.00 234.23 567.98 12.12 234.23 567.98 12.12 Input7.txt 555.66 333.44 123.45 755.23 778.88 900.00 123.12 234.23 567.98 555.66 778.88 900.00 123.12 999.99 234.23 567.98 12.12 555.66 333.44 778.88 234.23 567.98 12.12 987.65 432.11 820.17 Input8.txt 555.66 778.88 900.00 234.23 567.98 333.44 555.66 123.45 755.23 888.88 778.88 900.00 123.12 999.99 777.77 778.88 900.00 123.12 999.99 777.77 666.66 778.88 900.00 123.12 999.99 777.77 555.55 444.44 778.88 900.00 123.12 999.99 777.77 333.33 222.22 111.11 est Case 1 Please enter the file name or type QUIT to exit:\n quitENTER Test Case 2 Please enter the file name or type QUIT to exit:\n QUITENTER Test Case 3 Please enter the file name or type QUIT to exit:\n qUiTENTER Test Case 4 Please enter the file name or type QUIT to exit:\n QuItENTER Test Case 5 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n quitENTER Test Case 6 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n QUITENTER Test Case 7 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n QuItENTER Test Case 8 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n qUiTENTER Test Case 9 Please enter the file name or type QUIT to exit:\n input1.txtENTER Row 0 Length: 3, Subtotal: 6.000\n Row 1 Length: 3, Subtotal: 15.000\n Row 2 Length: 3, Subtotal: 24.000\n Column 0 Height: 3, Subtotal: 12.000\n Column 1 Height: 3, Subtotal: 15.000\n Column 2 Height: 3, Subtotal: 18.000\n Array Elements: 9, Total: 45.000\n Test Case 10 Please enter the file name or type QUIT to exit:\n badfile.txtENTER File: badfile.txt does not exist.\n Please enter the file name again or type QUIT to exit:\n input2.txtENTER Row 0 Length: 3, Subtotal: 1012.550\n Row 1 Length: 3, Subtotal: 1802.000\n Row 2 Length: 3, Subtotal: 814.330\n Column 0 Height: 3, Subtotal: 1568.770\n Column 1 Height: 3, Subtotal: 1801.420\n Column 2 Height: 3, Subtotal: 258.690\n Array Elements: 9, Total: 3628.880\n
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter7: String Manipulation
Section: Chapter Questions
Problem 6E
Related questions
Question
JAVA PROGRAM
Chapter 7. PC #16. 2D Array Operations with Additional Requirements
Write a program that creates an ArrayList of ArrayList of Doubles. The program should ask the user to enter the filename from the keyboard and validate the file for existence. Once the file is verified, the program should load the two-dimensional ArrayList with test data from the file. Be careful, as some of the files will contain rows with different number of elements. The program should work regardless whether the input data is perfectly rectangular or ragged. The program should have the following methods:
• main. Main entry point for the program.
• getRowSubtotal. This method should accept a two-dimensional ArrayList as its first argument and an integer as its second argument. The second argument should be the index of the row in the ArrayList. The method should return the subtotal of the values in the specified row.
• getColSubtotal. This method should accept a two-dimensional ArrayList as its first argument and an integer as its second argument. The second argument should be the index of the column in the ArrayList. The method should return the subtotal of the values in the specified column. If any of the rows has a size that is less than the column number, then that row should be skipped when calculating the column subtotal.
• getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array.
Demonstrate each of the methods in this program.
Input1.txt
1 2 3
4 5 6
7 8 9
4 5 6
7 8 9
Input2.txt
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
778.88 900.00 123.12
234.23 567.98 12.12
Input3.txt
555.66 333.44 123.45 555.66 333.44 123.45
778.88 900.00 123.12 778.88 900.00 123.12
234.23 567.98 12.12 234.23 567.98 12.12
778.88 900.00 123.12 778.88 900.00 123.12
234.23 567.98 12.12 234.23 567.98 12.12
Input4.txt
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
778.88 900.00 123.12
234.23 567.98 12.12
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
555.66 333.44 123.45
778.88 900.00 123.12
234.23 567.98 12.12
Input5.txt
555.66 333.44 123.45 555.66 333.44 123.45
778.88 900.00 123.12 778.88 900.00
234.23 567.98 12.12 234.23
778.88 900.00 123.12 778.88 900.00
234.23 567.98 12.12 234.23
Input6.txt
555.66 333.44 123.45 555.66
778.88 900.00 123.12 778.88 900.00
234.23 567.98 12.12 234.23 567.98 12.12
778.88 900.00 123.12 778.88 900.00
234.23 567.98 12.12 234.23 567.98 12.12
Input7.txt
555.66 333.44 123.45 755.23
778.88 900.00 123.12
234.23 567.98
555.66
778.88 900.00 123.12 999.99
234.23 567.98 12.12
555.66 333.44
778.88
234.23 567.98 12.12 987.65 432.11
820.17
778.88 900.00 123.12
234.23 567.98
555.66
778.88 900.00 123.12 999.99
234.23 567.98 12.12
555.66 333.44
778.88
234.23 567.98 12.12 987.65 432.11
820.17
Input8.txt
555.66
778.88 900.00
234.23 567.98 333.44
555.66 123.45 755.23 888.88
778.88 900.00 123.12 999.99 777.77
778.88 900.00 123.12 999.99 777.77 666.66
778.88 900.00 123.12 999.99 777.77 555.55 444.44
778.88 900.00 123.12 999.99 777.77 333.33 222.22 111.11
778.88 900.00
234.23 567.98 333.44
555.66 123.45 755.23 888.88
778.88 900.00 123.12 999.99 777.77
778.88 900.00 123.12 999.99 777.77 666.66
778.88 900.00 123.12 999.99 777.77 555.55 444.44
778.88 900.00 123.12 999.99 777.77 333.33 222.22 111.11
est Case 1
Please enter the file name or type QUIT to exit:\n
quitENTER
quitENTER
Test Case 2
Please enter the file name or type QUIT to exit:\n
QUITENTER
QUITENTER
Test Case 3
Please enter the file name or type QUIT to exit:\n
qUiTENTER
qUiTENTER
Test Case 4
Please enter the file name or type QUIT to exit:\n
QuItENTER
QuItENTER
Test Case 5
Please enter the file name or type QUIT to exit:\n
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
quitENTER
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
quitENTER
Test Case 6
Please enter the file name or type QUIT to exit:\n
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
QUITENTER
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
QUITENTER
Test Case 7
Please enter the file name or type QUIT to exit:\n
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
QuItENTER
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
QuItENTER
Test Case 8
Please enter the file name or type QUIT to exit:\n
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
qUiTENTER
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
qUiTENTER
Test Case 9
Please enter the file name or type QUIT to exit:\n
input1.txtENTER
Row 0 Length: 3, Subtotal: 6.000\n
Row 1 Length: 3, Subtotal: 15.000\n
Row 2 Length: 3, Subtotal: 24.000\n
Column 0 Height: 3, Subtotal: 12.000\n
Column 1 Height: 3, Subtotal: 15.000\n
Column 2 Height: 3, Subtotal: 18.000\n
Array Elements: 9, Total: 45.000\n
input1.txtENTER
Row 0 Length: 3, Subtotal: 6.000\n
Row 1 Length: 3, Subtotal: 15.000\n
Row 2 Length: 3, Subtotal: 24.000\n
Column 0 Height: 3, Subtotal: 12.000\n
Column 1 Height: 3, Subtotal: 15.000\n
Column 2 Height: 3, Subtotal: 18.000\n
Array Elements: 9, Total: 45.000\n
Test Case 10
Please enter the file name or type QUIT to exit:\n
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
input2.txtENTER
Row 0 Length: 3, Subtotal: 1012.550\n
Row 1 Length: 3, Subtotal: 1802.000\n
Row 2 Length: 3, Subtotal: 814.330\n
Column 0 Height: 3, Subtotal: 1568.770\n
Column 1 Height: 3, Subtotal: 1801.420\n
Column 2 Height: 3, Subtotal: 258.690\n
Array Elements: 9, Total: 3628.880\n
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
input2.txtENTER
Row 0 Length: 3, Subtotal: 1012.550\n
Row 1 Length: 3, Subtotal: 1802.000\n
Row 2 Length: 3, Subtotal: 814.330\n
Column 0 Height: 3, Subtotal: 1568.770\n
Column 1 Height: 3, Subtotal: 1801.420\n
Column 2 Height: 3, Subtotal: 258.690\n
Array Elements: 9, Total: 3628.880\n

Transcribed Image Text:Test Case 14
Please enter the file name or type QUIT to exit:
input6.txt ENTER
RowLength: 4, Subtotal: 1568.210
Row 1 Length: 5, Subtotal: 3480.880
Row 2 Length: 6, Subtotal: 1628.660
Column Height: 3, Subtotal: 1568.770 n
Column 1 Height: 3, Subtotal: 1801.420
Column 2 Height: 3, Subtotal: 258.690
Column 3 Height: 3, Subtotal: 1568.770
Column 4 Height: 2, Subtotal: 1467.980
Column 5 Height: 1, Subtotal: 12.120
Array Elements: 15, Total: 6677.750
Test Case 15
Please enter the file name or type QUIT to exit:
input7.txt ENTER
RowLength: 4, Subtotal: 1767.780 n
Row 1 Length: 3, Subtotal: 1802.000 n
Row 2 Length: 2, Subtotal: 802.210
Row 3 Length: 1, Subtotal: 555.660n
Row 4 Length: 4, Subtotal: 2801.990
Row 5 Length: 3, Subtotal: 814.330
Row 6 Length: 2, Subtotal: 889.100
Row 7 Length: 1, Subtotal: 778.880
Row 8 Length: 5, Subtotal: 2234.090
820.170
Column Height: 10, Subtotal: 5526.480
Row 9 Length: 1, Subtotal:
0
Column 1 Height: 7, Subtotal: 4170.820
Column 2 Height: 5, Subtotal: 393.930 \
Column 3 Height: 3, Subtotal: 2742.870 n
Column 4 Height: 1, Subtotal: 432.110
Array Elements: 26, Total: 13266.210
Test Case 16
Please enter the file name or type QUIT to exit:
input8.txt ENTER
RowLength: 1, Subtotal: 555.660
Row 1 Length: 2, Subtotal: 1678.880
Row 2 Length: 3, Subtotal: 1135.650
Row 3 Length: 4, Subtotal: 2323.220
Row 4 Length: 5, Subtotal: 3579.760
Row 5 Length: 6, Subtotal: 4246.420
Row 6 Length: 7, Subtotal: 4579.750
Row 7 Length: 8, Subtotal: 4246.420 n
Column Height: 8, Subtotal: 5239.950
Column 1 Height: 7, Subtotal: 5191.430
Column 2 Height: 6, Subtotal: 1581.150
Column 3 Height: 5, Subtotal: 4888.840
Column 4 Height: 4, Subtotal: 3111.080
Column 5 Height: 3, Subtotal: 1555.540
Column 6 Height: 2, Subtotal: 666.660
Column 7 Height: 1, Subtotal: 111.110
Array Elements: 36, Total: 22345.760

Transcribed Image Text:Test Case 11
Please enter the file name or type QUIT to exit: n
badfile.txt ENTER
File: badfile.txt does not exist.
Please enter the file name again or type QUIT to exit:
badfile2.txt ENTER
File: badfile2.txt does not exist.
Please enter the file name again or type QUIT to exit:
input3, txt
.txt ENTER
RowLength: 6, Subtotal: 2025.100 \n
Row 1 Length: 6, Subtotal: 3604.000
Row 2 Length: 6, Subtotal: 1628.660
.000 n
Column Height: 3, Subtotal: 1568.770
Column 1 Height: 3, Subtotal: 1801.420 n
Column 2 Height: 3, Subtotal: 258.690
Column 3 Height: 3, Subtotal: 1568.7705
Column 4 Height: 3, Subtotal: 1801.420
Column 5 Height: 3, Subtotal: 258.690
Array Elements: 18, Total: 7257.760
Test Case 12
Please enter the file name or type QUIT to exit:
badfile.txt ENTER
File: badfile.txt does not exist.
Please enter the file name again or type QUIT to exit: M
badfile2.txt ENTER
File: badfile2.txt does not exist.n
Tato
Please enter the file name again or type QUIT to exit: M
2002
badfile3.txt ENTER
PAVA
File: badfile3.txt does not exist. n
Please enter the file name again or type QUIT to exit:
tut
input4.txt ENTER
Row
Length: 3, Subtotal: 1012.550
Row 1 1 Length: 3, Subtotal: 1802.000
Row 2 Length: 3, Subtotal: 814.330
Row 3 Length: 3, Subtotal: 1012.550
Row 4 Length: 3, Subtotal: 1802.000
Row 5 Length: 3, Subtotal: 814.330[
Row 6 Length: 3, Subtotal: 1012.550
Row 7 Length: 3,
3,
Subtotal: 1802.000
02.0000
Row 8 Length: 3, Subtotal: 814.330
Column Height: 9, Subtotal: 4706.310n
Column 1 Height: 9, Subtotal: 5404.260
Column 2 Height: 9, Subtotal: 776.070
Array Elements: 27, Total: 10886.640
Test Case 13
Please enter the file name or type QUIT to exit:
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit: m
badfile2.txt ENTER
Dagr
File: badfile2.txt does not exist.\n
A
Please enter the file name again or type QUIT to exit:
con ene
badfile3.txt [ENTER
e badle tv
File: badfile3.txt does not exist.\n
A
Please enter the file name again or type QUIT to exit:
2002
badfile4.txt ENTER
File: badfile4.txt does not exist.
Please enter the file name again or type QUIT to exit:
inputs.txt
tyt [ENTER
ENTER
RowLength: 6, Subtotal: 2025.100 m
Row 1 Length: 5, Subtotal: 3480.880
Row 2 Length: 4, Subtotal: 1848.560
Height: 3, Subtotal: 1568.770
Column
Column 1 Height: 3, Subtotal: 1801.420 n
Column 2 Height: 3, Subtotal: 258.69€
Column 3 Height: 3, Subtotal: 1568.770
Column 4 Height: 2, Subtotal: 1233.440
Column 5 Height: 1, Subtotal: 123.450
Array Elements: 15, Total: 6554.540
Test Case 14
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
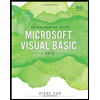
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
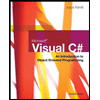
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
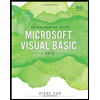
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
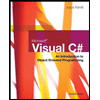
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage