JAVA PROGRAM Lab #2. Chapter 7. PC #11. Array Operations (Page 491) Write a program that accepts a file name from command line, then initializes an array with test data using that text file as an input. The file should contain floating point numbers (use double data type). The program should also have the following methods: * getTotal. This method should accept a one-dimensional array as its argument and return the total of the values in the array. * getAverage. This method should accept a one-dimensional array as its argument and return the average of the values in the array. * getHighest. This method should accept a one-dimensional array as its argument and return the highest value in the array. * getLowest. This method should accept a one-dimensional array as its argument and return the lowest value in the array. PLEASE FIX AND MODIFY THIS JAVA PROGRAM. THIS PROGRAM DOES NOT WORK IN HYPERGERADE. IT ONLY PASSSES 2 OUT OF 4 TEST CASES. I NEED IT TO PASS 4 OUT OF 4 TEST CASES. FIX IT PLEASE. IT IS IMPORTANT AND URGENT FOR ME. THE PICTURES THAT I PROVIDED PROOF THAT WHEN I UPLOAD IT TO HYPERGRADES IT FAILS TEST CASSES. THE PROGRAM SHOULD OUTPUT THE TEST CASES WHICH IS DOWN BELOW WHEN I UPLOAD IT TO HYPERGRADE. THANK YOU. import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.List; public class ArrayOperations { public static void main(String[] args) { if (args.length != 1) { System.err.println("Usage: java ArrayOperations "); System.exit(1); // Exit with an error code } String fileName = args[0]; double[] data = readDataFromFile(fileName); if (data != null) { System.out.printf("Total: %.3f%n", getTotal(data)); System.out.printf("Average: %.3f%n", getAverage(data)); System.out.printf("Highest: %.3f%n", getHighest(data)); System.out.printf("Lowest: %.3f%n", getLowest(data)); } } public static double[] readDataFromFile(String fileName) { List dataList = new ArrayList<>(); try (BufferedReader br = new BufferedReader(new FileReader(fileName))) { String line; while ((line = br.readLine()) != null) { line = line.trim(); if (!line.isEmpty()) { dataList.add(Double.parseDouble(line)); } } } catch (IOException e) { System.err.println("File: " + fileName + " does not exist."); return null; } catch (NumberFormatException e) { System.err.println("Invalid data format in the file: " + e.getMessage()); return null; } double[] data = new double[dataList.size()]; for (int i = 0; i < dataList.size(); i++) { data[i] = dataList.get(i); } return data; } public static double getTotal(double[] arr) { double total = 0; for (double num : arr) { total += num; } return total; } public static double getAverage(double[] arr) { if (arr.length == 0) { return 0; } return getTotal(arr) / arr.length; } public static double getHighest(double[] arr) { double highest = Double.NEGATIVE_INFINITY; for (double num : arr) { if (num > highest) { highest = num; } } return highest; } public static double getLowest(double[] arr) { double lowest = Double.POSITIVE_INFINITY; for (double num : arr) { if (num < lowest) { lowest = num; } } return lowest; } } input1.txt -283.760 -456.167 19.815 -322.301 344.949 -850.533 -672.360 -188.767 646.462 -118.775 808.613 -746.865 -370.432 219.607 -166.298 -508.636 -989.128 -205.020 -928.165 -180.699 300.753 316.036 709.371 -886.860 -585.388 -554.302 -394.801 -970.233 905.941 787.854 -181.240 74.665 802.453 -951.292 -510.656 -203.999 -276.199 -350.575 398.501 -519.799 469.199 592.120 713.424 155.967 585.481 -780.846 387.700 457.806 560.933 -343.916 486.806 -43.184 237.494 191.488 309.275 -64.415 -206.333 -377.696 -409.553 -734.282 -777.221 -318.800 -695.745 40.631 -384.036 -937.134 -380.501 -77.083 756.524 -720.959 -579.412 -215.301 542.097 -402.541 468.721 151.050 573.296 -342.210 758.038 200.691 882.294 8.042 -87.760 634.811 -777.953 767.795 570.716 594.012 596.648 900.010 -370.754 985.007 785.562 -838.952 -71.182 72.845 -500.759 698.946 527.167 1.082 Test Case 1 Command Line arguments: double_input1.txt Total: -1,813.080\n Average: -18.131\n Highest: 985.007\n Lowest: -989.128\n Test Case 2 Command Line arguments: double_input2.txt Total: -331,368.178\n Average: -165.684\n Highest: 9,994.439\n Lowest: -9,988.269\n Test Case 3 Command Line arguments: double_input3.txt File: double_input3.txt does not exist.\n Test Case 4 Usage: java ArrayOperations \n
JAVA PROGRAM
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class ArrayOperations {
public static void main(String[] args) {
if (args.length != 1) {
System.err.println("Usage: java ArrayOperations <filename>");
System.exit(1); // Exit with an error code
}
String fileName = args[0];
double[] data = readDataFromFile(fileName);
if (data != null) {
System.out.printf("Total: %.3f%n", getTotal(data));
System.out.printf("Average: %.3f%n", getAverage(data));
System.out.printf("Highest: %.3f%n", getHighest(data));
System.out.printf("Lowest: %.3f%n", getLowest(data));
}
}
public static double[] readDataFromFile(String fileName) {
List<Double> dataList = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = br.readLine()) != null) {
line = line.trim();
if (!line.isEmpty()) {
dataList.add(Double.parseDouble(line));
}
}
} catch (IOException e) {
System.err.println("File: " + fileName + " does not exist.");
return null;
} catch (NumberFormatException e) {
System.err.println("Invalid data format in the file: " + e.getMessage());
return null;
}
double[] data = new double[dataList.size()];
for (int i = 0; i < dataList.size(); i++) {
data[i] = dataList.get(i);
}
return data;
}
public static double getTotal(double[] arr) {
double total = 0;
for (double num : arr) {
total += num;
}
return total;
}
public static double getAverage(double[] arr) {
if (arr.length == 0) {
return 0;
}
return getTotal(arr) / arr.length;
}
public static double getHighest(double[] arr) {
double highest = Double.NEGATIVE_INFINITY;
for (double num : arr) {
if (num > highest) {
highest = num;
}
}
return highest;
}
public static double getLowest(double[] arr) {
double lowest = Double.POSITIVE_INFINITY;
for (double num : arr) {
if (num < lowest) {
lowest = num;
}
}
return lowest;
}
}
input1.txt
-283.760
-456.167
19.815
-322.301
344.949
-850.533
-672.360
-188.767
646.462
-118.775
808.613
-746.865
-370.432
219.607
-166.298
-508.636
-989.128
-205.020
-928.165
-180.699
300.753
316.036
709.371
-886.860
-585.388
-554.302
-394.801
-970.233
905.941
787.854
-181.240
74.665
802.453
-951.292
-510.656
-203.999
-276.199
-350.575
398.501
-519.799
469.199
592.120
713.424
155.967
585.481
-780.846
387.700
457.806
560.933
-343.916
486.806
-43.184
237.494
191.488
309.275
-64.415
-206.333
-377.696
-409.553
-734.282
-777.221
-318.800
-695.745
40.631
-384.036
-937.134
-380.501
-77.083
756.524
-720.959
-579.412
-215.301
542.097
-402.541
468.721
151.050
573.296
-342.210
758.038
200.691
882.294
8.042
-87.760
634.811
-777.953
767.795
570.716
594.012
596.648
900.010
-370.754
985.007
785.562
-838.952
-71.182
72.845
-500.759
698.946
527.167
1.082
Test Case 1
Average: -18.131\n
Highest: 985.007\n
Lowest: -989.128\n
Test Case 2
Average: -165.684\n
Highest: 9,994.439\n
Lowest: -9,988.269\n
Test Case 3
Test Case 4

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

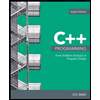
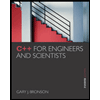
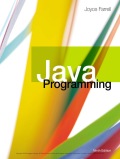
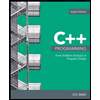
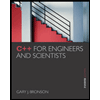
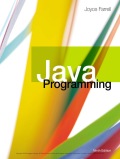
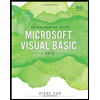