JAVA Program Modify this program with further modidications: it should not use a while loop. Instead try to write a recursive method that doesn't copy the string over and over again. I have provided the failed test cases. import java.util.Scanner; class Main { public static boolean isPalindrome(String str, int start, int end) { if (start >= end) { return true; } else if (str.charAt(start) == str.charAt(end)) { return isPalindrome(str, start + 1, end - 1); } else { return false; } } public static void main(String[] args) { Scanner sc = new Scanner(System.in); String str; do { System.out.println("Please enter a string to test for palindrome or type QUIT to exit:"); str = sc.nextLine().toLowerCase(); if (str.equals("quit")) { break; } else { if (isPalindrome(str, 0, str.length() - 1)) { System.out.println("The input is a palindrome."); } else { System.out.println("The input is not a palindrome."); } } } while (true); } } Chapter 16. PC #5. Palindrome Detector (page 1073) A palindrome is any word, phrase, or sentence that reads the same forward and backward. Here are some well-known palindromes: Able was I, ere I saw Elba A man, a plan, a canal, Panama Desserts, I stressed Kayak Write a boolean method that uses recursion to determine whether a String argument is a palindrome. The method should return true if the argument reads the same forward and backward. Demonstrate the method in a program. The program should ask the user to enter a string, which is checked for palindrome property. The program displays whether the given input is a palindrome or not, then prompts the user to enter another string. If the user enters QUIT (case insensitive, then exit the program). Test Case 1 Please enter a string to test for palindrome or type QUIT to exit:\n Desserts, I stressedENTER The input is a palindrome.\n Please enter a string to test for palindrome or type QUIT to exit:\n KayakENTER The input is a palindrome.\n Please enter a string to test for palindrome or type QUIT to exit:\n quitENTER Test Case 2 Please enter a string to test for palindrome or type QUIT to exit:\n dadENTER The input is a palindrome.\n Please enter a string to test for palindrome or type QUIT to exit:\n quitENTER Test Case 3 Please enter a string to test for palindrome or type QUIT to exit:\n momENTER The input is a palindrome.\n Please enter a string to test for palindrome or type QUIT to exit:\n QuitENTER Test Case 4 Please enter a string to test for palindrome or type QUIT to exit:\n helloENTER The input is not a palindrome.\n Please enter a string to test for palindrome or type QUIT to exit:\n quiTENTER Test Case 5 Please enter a string to test for palindrome or type QUIT to exit:\n b,a,dENTER The input is not a palindrome.\n Please enter a string to test for palindrome or type QUIT to exit:\n quitENTER Test Case 6 Please enter a string to test for palindrome or type QUIT to exit:\n Able was I, ere I saw ElbaENTER The input is a palindrome.\n Please enter a string to test for palindrome or type QUIT to exit:\n quitENTER
import java.util.Scanner;
class Main {
public static boolean isPalindrome(String str, int start, int end) {
if (start >= end) {
return true;
} else if (str.charAt(start) == str.charAt(end)) {
return isPalindrome(str, start + 1, end - 1);
} else {
return false;
}
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str;
do {
System.out.println("Please enter a string to test for palindrome or type QUIT to exit:");
str = sc.nextLine().toLowerCase();
if (str.equals("quit")) {
break;
} else {
if (isPalindrome(str, 0, str.length() - 1)) {
System.out.println("The input is a palindrome.");
} else {
System.out.println("The input is not a palindrome.");
}
}
} while (true);
}
}
Test Case 1
Desserts, I stressedENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
KayakENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 2
dadENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 3
momENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
QuitENTER
Test Case 4
helloENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quiTENTER
Test Case 5
b,a,dENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 6
Able was I, ere I saw ElbaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 7
A man, a plan, a canal, PanamaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 8
@abENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
ab@ENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
@aaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
aa@ENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 9
abbaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
abcaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
aabcaaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
abaceedabaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER



Step by step
Solved in 3 steps with 3 images

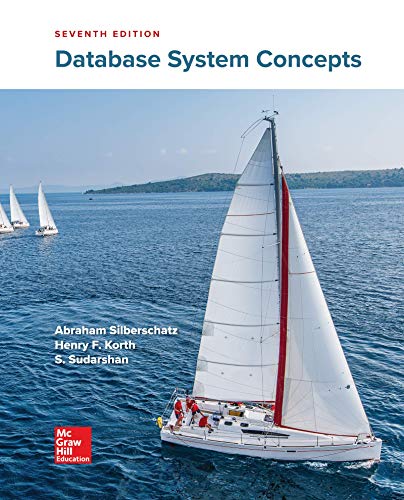
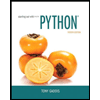
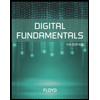
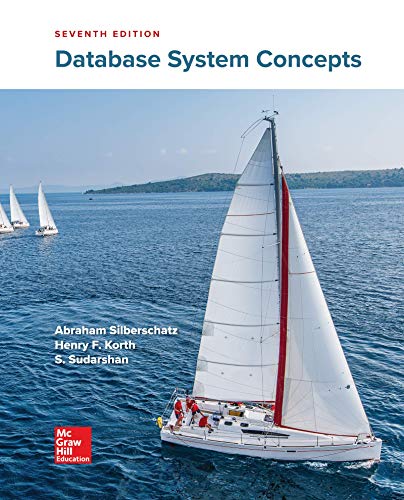
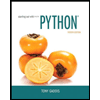
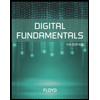
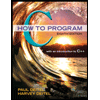
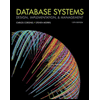
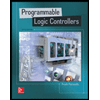