Modify the sample code to prompt the user for a name. Have the program search the existing list for the entered name. If the name is in the list, display the corresponding phone number; otherwise display this message, The name is not in the current phone directory. #include #include using namespace std; const int MAXNAME = 30; const int MAXTEL = 15; struct Tele_typ { char name[MAXNAME]; char phone_no[MAXTEL]; Tele_typ* nextaddr; }; void display(Tele_typ*); int main() { Tele_typ t1 = { " Cage, Mark", "(555) 898-2392" }; Tele_typ t2 = { " Dolan, Nick", "(555) 682-3104" }; Tele_typ t3 = { " Lennon, John", "(555) 718-4581" }; Tele_typ* first; first = &t1; t1.nextaddr = &t2; t2.nextaddr = &t3; t3.nextaddr = NULL; display(first); return 0; } void display(Tele_typ * contents) // of type Tele_typ { while (contents != NULL) { cout.setf(ios::left); cout.width(25); cout << '\n' << contents->name; cout.width(20); cout << contents->phone_no; contents = contents->nextaddr; }cout << endl; return; }
Modify the sample code to prompt the user for a name. Have the
program search the existing list for the entered name. If the
name is in the list, display the corresponding phone number;
otherwise display this message,
The name is not in the current phone directory.
#include<iostream>
#include<iomanip>
using namespace std;
const int MAXNAME = 30;
const int MAXTEL = 15;
struct Tele_typ
{
char name[MAXNAME];
char phone_no[MAXTEL];
Tele_typ* nextaddr;
};
void display(Tele_typ*);
int main()
{
Tele_typ t1 = { " Cage, Mark", "(555) 898-2392" };
Tele_typ t2 = { " Dolan, Nick", "(555) 682-3104" };
Tele_typ t3 = { " Lennon, John", "(555) 718-4581" };
Tele_typ* first;
first = &t1;
t1.nextaddr = &t2;
t2.nextaddr = &t3;
t3.nextaddr = NULL;
display(first);
return 0;
}
void display(Tele_typ * contents)
// of type Tele_typ
{
while (contents != NULL)
{
cout.setf(ios::left);
cout.width(25); cout << '\n' << contents->name;
cout.width(20); cout << contents->phone_no;
contents = contents->nextaddr;
}cout << endl;
return;
}

Step by step
Solved in 2 steps

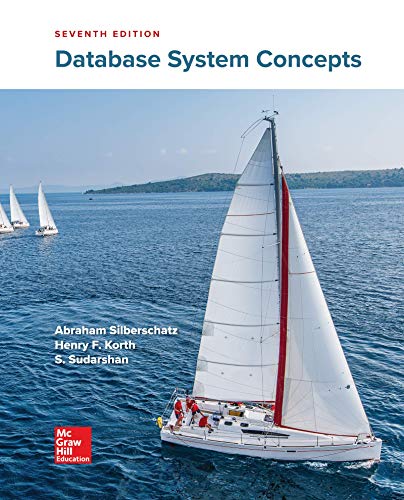
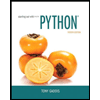
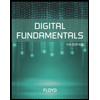
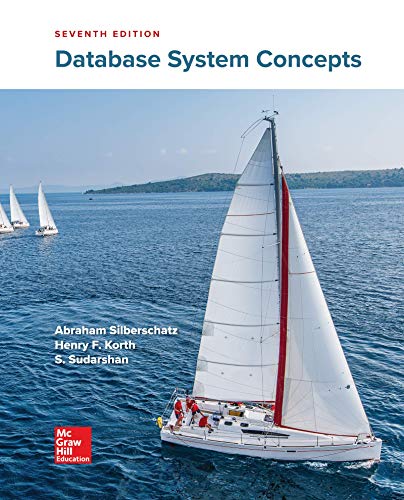
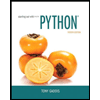
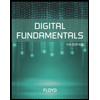
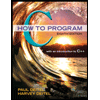
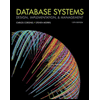
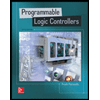