n c++ i have this struct struct Student { string firstName, lastName; int pointTotal; }; void printStudent(const Student & s) { cout << s.pointTotal << "\t" << s.lastName << ", " << s.firstName << endl; } void printAll(Student students[], int numStudents) { for (int i = 0; i < numStudents; i++) printStudent(students[i]); cout << endl; } int main() { const int NUMSTUDENTS = 7; Student students[NUMSTUDENTS] = { {"Brian", "Jones", 45},{"Edith", "Piaf", 45}, {"Jacques", "Brel", 64},{"Anna", "Brel", 64}, {"Carmen", "Jones", 45} , {"Carmen", "Brel", 64}, {"Antoine", "Piaf", 45}, {"Pascal", "Piaf", 64} }; printAll(students, NUMSTUDENTS); sortByPointTotal(students, NUMSTUDENTS); printAll(students, NUMSTUDENTS); return 0; } i need to make a function that uses bubble sort(coded from scratch), but modify it so that the comparison for out-of-order elements takes into account all three fields: totalPoints, lastName and firstName, in decreasing order of importance. In other words... sort by totalPoints as the primary criterion and put bigger point totals before smaller point totals. Then among students with the same totalPoints, sort by lastName, putting students with an alphabetically smaller last name before students with an alphabetically bigger last name. Finally among students with the same totalPoints and lastName, sort by firstName, again in ascending order. the output should look like the image like
in c++
i have this struct
struct Student {
string firstName, lastName;
int pointTotal;
};
void printStudent(const Student & s) {
cout << s.pointTotal << "\t" << s.lastName << ", " << s.firstName << endl;
}
void printAll(Student students[], int numStudents) {
for (int i = 0; i < numStudents; i++)
printStudent(students[i]);
cout << endl;
}
int main() {
const int NUMSTUDENTS = 7;
Student students[NUMSTUDENTS] = {
{"Brian", "Jones", 45},{"Edith", "Piaf", 45},
{"Jacques", "Brel", 64},{"Anna", "Brel", 64},
{"Carmen", "Jones", 45} , {"Carmen", "Brel", 64},
{"Antoine", "Piaf", 45}, {"Pascal", "Piaf", 64} };
printAll(students, NUMSTUDENTS);
sortByPointTotal(students, NUMSTUDENTS);
printAll(students, NUMSTUDENTS);
return 0;
}
i need to make a function that uses bubble sort(coded from scratch), but modify it so that the comparison for out-of-order elements takes into account all three fields: totalPoints, lastName and firstName, in decreasing order of importance. In other words... sort by totalPoints as the primary criterion and put bigger point totals before smaller point totals. Then among students with the same totalPoints, sort by lastName, putting students with an alphabetically smaller last name before students with an alphabetically bigger last name. Finally among students with the same totalPoints and lastName, sort by firstName, again in ascending order.
the output should look like the image like


Step by step
Solved in 2 steps with 1 images

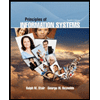
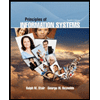