Need python help running code and others. Functions are ideal for use in menu-driven programs. When the user selects an item from a menu, the program can call the appropriate function. Write a menu-driven program for Burgers. Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!) Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!" Ask the user what he/she wants and how many of them. (Check the user inputs) Keep asking the user until he/she chooses the exit option. Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data. Calculate the price. Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price. Display(print) the bill to the user. The bill includes: The food items The quantities The cost of them The total before tax Tax Total price after tax My python code: class ArrayBag(object): """An array-based bag implementation.""" # Class variable DEFAULT_CAPACITY = 10 # Constructor def __init__(self, sourceCollection = None): """Sets the initial state of self, which includes the contents of sourceCollection, if it's present.""" self.items = Array(ArrayBag.DEFAULT_CAPACITY) self.size = 0 if sourceCollection: for item in sourceCollection: self.add(item) # Accessor methods def isEmpty(self): """Returns True if len(self) == 0, or False otherwise.""" return len(self) == 0 def __len__(self): """Returns the number of items in self.""" return self.size def __str__(self): """Returns the string representation of self.""" return "{" + ", ".join(map(str, self)) + "}" def __iter__(self): """Supports iteration over a view of self.""" cursor = 0 while cursor < len(self): yield self.items[cursor] cursor += 1 # Mutator methods def clear(self): """Makes self become empty.""" self.size = 0 self.items = Array(ArrayBag.DEFAULT_CAPACITY) def add(self, item): """Adds item to self.""" if self.size == len(self): self._expand() self.items[self.size] = item self.size += 1 def _expand(self): """Doubles the capacity of self.""" old = self.items self.items = Array(2 * len(old)) for count in range(len(old)): self.items[count] = old[count] # order.py from array import ArrayBag class Order: def __init__(self): self._price_before_tax = 0 self._price_after_tax = 0 self._tax = 0 self._order_items = Array(10) def display_menu(self): print("\n----------- Food Court -----------") print("1. Burger - $5.00") print("2. Pizza - $8.00") print("3. Sandwich - $6.00") print("4. Salad - $4.00") print("5. Pasta - $7.00") def get_inputs(self): self._order_items.clear() while True: try: item_number = int(input("Enter the number of the item you want to order: ")) if item_number == 6: break quantity = int(input("Enter the quantity: ")) if quantity <= 0: raise ValueError("Quantity must be greater than zero.") self._order_items[len(self._order_items)] = (item_number, quantity) self._order_items.size += 1 except ValueError as e: print(e) def calculate(self): self._price_before_tax = 0 for item in self._order_items: if item is None: continue if item[0] == 1: price = 5.0 elif item[0] == 2: price = 8.0 elif item[0] == 3: price = 6.0 elif item[0] == 4: price = 4.0 elif item[0] == 5: price = 7.0 else: raise ValueError("Invalid item number.") self._price_before_tax += price * item[1] def main(): print("Welcome...") order = Order() order.display_menu() if __name__ == "__main__": main()
Need python help running code and others. Functions are ideal for use in menu-driven programs. When the user selects an item from a menu, the program can call the appropriate function. Write a menu-driven program for Burgers. Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!) Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!" Ask the user what he/she wants and how many of them. (Check the user inputs) Keep asking the user until he/she chooses the exit option. Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data. Calculate the price. Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price. Display(print) the bill to the user. The bill includes: The food items The quantities The cost of them The total before tax Tax Total price after tax My python code: class ArrayBag(object): """An array-based bag implementation.""" # Class variable DEFAULT_CAPACITY = 10 # Constructor def __init__(self, sourceCollection = None): """Sets the initial state of self, which includes the contents of sourceCollection, if it's present.""" self.items = Array(ArrayBag.DEFAULT_CAPACITY) self.size = 0 if sourceCollection: for item in sourceCollection: self.add(item) # Accessor methods def isEmpty(self): """Returns True if len(self) == 0, or False otherwise.""" return len(self) == 0 def __len__(self): """Returns the number of items in self.""" return self.size def __str__(self): """Returns the string representation of self.""" return "{" + ", ".join(map(str, self)) + "}" def __iter__(self): """Supports iteration over a view of self.""" cursor = 0 while cursor < len(self): yield self.items[cursor] cursor += 1 # Mutator methods def clear(self): """Makes self become empty.""" self.size = 0 self.items = Array(ArrayBag.DEFAULT_CAPACITY) def add(self, item): """Adds item to self.""" if self.size == len(self): self._expand() self.items[self.size] = item self.size += 1 def _expand(self): """Doubles the capacity of self.""" old = self.items self.items = Array(2 * len(old)) for count in range(len(old)): self.items[count] = old[count] # order.py from array import ArrayBag class Order: def __init__(self): self._price_before_tax = 0 self._price_after_tax = 0 self._tax = 0 self._order_items = Array(10) def display_menu(self): print("\n----------- Food Court -----------") print("1. Burger - $5.00") print("2. Pizza - $8.00") print("3. Sandwich - $6.00") print("4. Salad - $4.00") print("5. Pasta - $7.00") def get_inputs(self): self._order_items.clear() while True: try: item_number = int(input("Enter the number of the item you want to order: ")) if item_number == 6: break quantity = int(input("Enter the quantity: ")) if quantity <= 0: raise ValueError("Quantity must be greater than zero.") self._order_items[len(self._order_items)] = (item_number, quantity) self._order_items.size += 1 except ValueError as e: print(e) def calculate(self): self._price_before_tax = 0 for item in self._order_items: if item is None: continue if item[0] == 1: price = 5.0 elif item[0] == 2: price = 8.0 elif item[0] == 3: price = 6.0 elif item[0] == 4: price = 4.0 elif item[0] == 5: price = 7.0 else: raise ValueError("Invalid item number.") self._price_before_tax += price * item[1] def main(): print("Welcome...") order = Order() order.display_menu() if __name__ == "__main__": main()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
Need python help running code and others.
Functions are ideal for use in menu-driven programs. When the user selects an item from a menu, the program can call the appropriate function.
Write a menu-driven program for Burgers.
Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!)
Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!"
Ask the user what he/she wants and how many of them. (Check the user inputs)
Keep asking the user until he/she chooses the exit option.
Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data.
Calculate the price.
Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price.
Display(print) the bill to the user. The bill includes:
The food items
The quantities
The cost of them
The total before tax
Tax
Total price after tax
My python code:
class ArrayBag(object):
"""An array-based bag implementation."""
# Class variable
DEFAULT_CAPACITY = 10
# Constructor
def __init__(self, sourceCollection = None):
"""Sets the initial state of self, which includes the
contents of sourceCollection, if it's present."""
self.items = Array(ArrayBag.DEFAULT_CAPACITY)
self.size = 0
if sourceCollection:
for item in sourceCollection:
self.add(item)
# Accessor methods
def isEmpty(self):
"""Returns True if len(self) == 0, or False otherwise."""
return len(self) == 0
def __len__(self):
"""Returns the number of items in self."""
return self.size
def __str__(self):
"""Returns the string representation of self."""
return "{" + ", ".join(map(str, self)) + "}"
def __iter__(self):
"""Supports iteration over a view of self."""
cursor = 0
while cursor < len(self):
yield self.items[cursor]
cursor += 1
# Mutator methods
def clear(self):
"""Makes self become empty."""
self.size = 0
self.items = Array(ArrayBag.DEFAULT_CAPACITY)
def add(self, item):
"""Adds item to self."""
if self.size == len(self):
self._expand()
self.items[self.size] = item
self.size += 1
def _expand(self):
"""Doubles the capacity of self."""
old = self.items
self.items = Array(2 * len(old))
for count in range(len(old)):
self.items[count] = old[count]
# order.py
from array import ArrayBag
class Order:
def __init__(self):
self._price_before_tax = 0
self._price_after_tax = 0
self._tax = 0
self._order_items = Array(10)
def display_menu(self):
print("\n----------- Food Court -----------")
print("1. Burger - $5.00")
print("2. Pizza - $8.00")
print("3. Sandwich - $6.00")
print("4. Salad - $4.00")
print("5. Pasta - $7.00")
def get_inputs(self):
self._order_items.clear()
while True:
try:
item_number = int(input("Enter the number of the item you want to order: "))
if item_number == 6:
break
quantity = int(input("Enter the quantity: "))
if quantity <= 0:
raise ValueError("Quantity must be greater than zero.")
self._order_items[len(self._order_items)] = (item_number, quantity)
self._order_items.size += 1
except ValueError as e:
print(e)
def calculate(self):
self._price_before_tax = 0
for item in self._order_items:
if item is None:
continue
if item[0] == 1:
price = 5.0
elif item[0] == 2:
price = 8.0
elif item[0] == 3:
price = 6.0
elif item[0] == 4:
price = 4.0
elif item[0] == 5:
price = 7.0
else:
raise ValueError("Invalid item number.")
self._price_before_tax += price * item[1]
def main():
print("Welcome...")
order = Order()
order.display_menu()
if __name__ == "__main__":
main()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
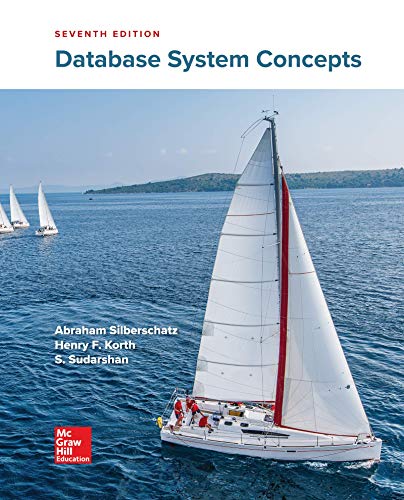
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
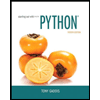
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
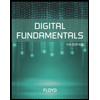
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
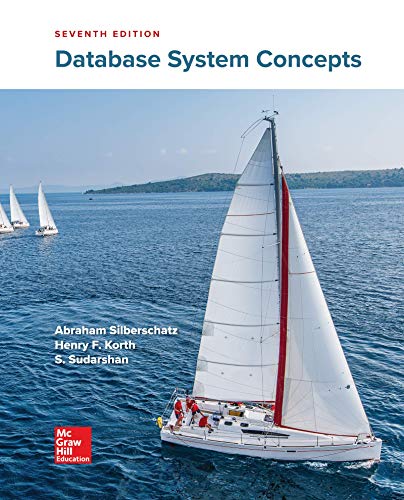
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
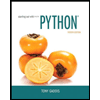
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
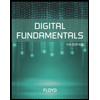
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
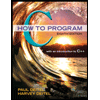
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
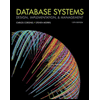
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
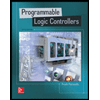
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education