Now let's get ready for some customers. Ensure that your Library can do the following: 1. Print opening hours for all libraries (they all open and close at the same time 9AM-5PM). 2. Print the address of the library. 3. Add a book to the book collection. 4. Borrow and return books from the book collection. 5. Print all available books. With all these methods implemented we are ready to open to the public! Pre-opening Day You've realized that you should test your implementation before opening it the public! This means you will act as the first customer to the libraries you are building, and perform at least the following in your main method: 1. Enter your libraries (one is at "120 Queen St.", and the other at "228 College St."). 2. In the Library on Queen street, add these four books to the collection: The DaVinci Code a. b. 3. 4. Le Petit Prince A Tale of Two Cities C. d. The Lord of The Rings Leave the library on College street empty. Print the opening hours for the libraries, and make sure they all match (i.e. not unique to instances) 5. Do the same for the addresses but this time they shouldn't match. 6. Borrow "The Lord of The Rings" at the library on Queen St., and without returning it, try to borrow it again. 7. Borrow the same book at the library on College St. 8. Print the books you have available at each library. 9. Return the book "The Lord of the Rings". 10. Re-print the books available. Competition A competing library opened up next door on the same day as you. The library has all the same features as yours currently has. Implement some feature(s) in your library that will attract more people to yours! It should be created using 2 additional classes.
Now let's get ready for some customers. Ensure that your Library can do the following: 1. Print opening hours for all libraries (they all open and close at the same time 9AM-5PM). 2. Print the address of the library. 3. Add a book to the book collection. 4. Borrow and return books from the book collection. 5. Print all available books. With all these methods implemented we are ready to open to the public! Pre-opening Day You've realized that you should test your implementation before opening it the public! This means you will act as the first customer to the libraries you are building, and perform at least the following in your main method: 1. Enter your libraries (one is at "120 Queen St.", and the other at "228 College St."). 2. In the Library on Queen street, add these four books to the collection: The DaVinci Code a. b. 3. 4. Le Petit Prince A Tale of Two Cities C. d. The Lord of The Rings Leave the library on College street empty. Print the opening hours for the libraries, and make sure they all match (i.e. not unique to instances) 5. Do the same for the addresses but this time they shouldn't match. 6. Borrow "The Lord of The Rings" at the library on Queen St., and without returning it, try to borrow it again. 7. Borrow the same book at the library on College St. 8. Print the books you have available at each library. 9. Return the book "The Lord of the Rings". 10. Re-print the books available. Competition A competing library opened up next door on the same day as you. The library has all the same features as yours currently has. Implement some feature(s) in your library that will attract more people to yours! It should be created using 2 additional classes.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
in Java

Transcribed Image Text:Now let's get ready for some customers. Ensure that your Library can do the following:
1. Print opening hours for all libraries (they all open and close at the same time 9AM-5PM).
2. Print the address of the library.
3. Add a book to the book collection.
4. Borrow and return books from the book collection.
5. Print all available books.
With all these methods implemented we are ready to open to the public!
Pre-opening Day
You've realized that you should test your implementation before opening it the public! This means you will
act as the first customer to the libraries you are building, and perform at least the following in your main
method:
1. Enter your libraries (one is at "120 Queen St.", and the other at "228 College St.").
2. In the Library on Queen street, add these four books to the collection:
a. The DaVinci Code
b.
Le Petit Prince
A Tale of Two Cities
C.
d. The Lord of The Rings
3.
Leave the library on College street empty.
4. Print the opening hours for the libraries, and make sure they all match (i.e. not unique to
instances)
5.
Do the same for the addresses but this time they shouldn't match.
6. Borrow "The Lord of The Rings" at the library on Queen St., and without returning it, try to
borrow it again.
7.
Borrow the same book at the library on College St.
8. Print the books you have available at each library.
9. Return the book "The Lord of the Rings".
10. Re-print the books available.
Competition
A competing library opened up next door on the same day as you. The library has all the same features
as yours currently has. Implement some feature(s) in your library that will attract more people to yours! It
should be created using 2 additional classes.

Transcribed Image Text:in separate files.
you'll need to build and run a small Library in Lennoxville. All classes must be created
Book
First, start by building a class that describes a Book at a Library. It should have one private instance
variable for the title of the book, and one for its availability (borrowed or in stock).
Add methods for setting and getting the availability variable, along with a getter for the title. Consider how
these should be named for code clarity. For instance, using a method called returned () to return a
Book to the Library could be used as a setter for the borrowed field. The same logic could be applied to
when it's borrowed:
public void borrow () {
}
this.borrowed = true;
Furthermore, the getter for the borrowed variable could be named isBorrowed as it is more descriptive
about what it answers when compared to getBorrowed. Keep this technique in mind for future class
designs.
Library
Now we have the inventory designed and ready for building a little Library!
Build a class called Library that contains a book collection and a given address. Given that we'd like to
expand our book collection over time, we won't be able to use standard arrays. To have a dynamically-
sized, resizable, or growable array, we will make use of the ArrayList class:
https://docs.oracle.com/en/java/javase/21/docs/api/java.base/java/util/ArrayList.html
To make a vector for the book collection, you can do the following:
ArrayList<Book> bookCollection = new ArrayList<Book> ();
bookCollection.add (new Book ("The Lord of the Rings"));
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
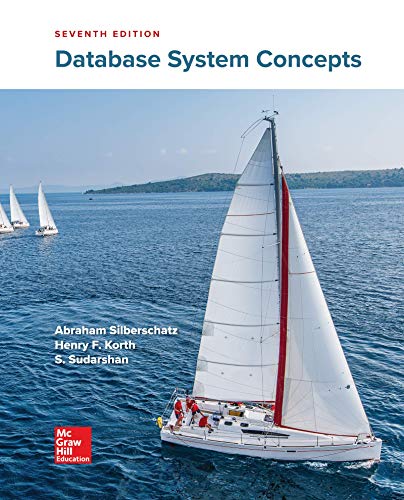
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
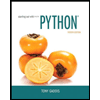
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
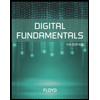
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
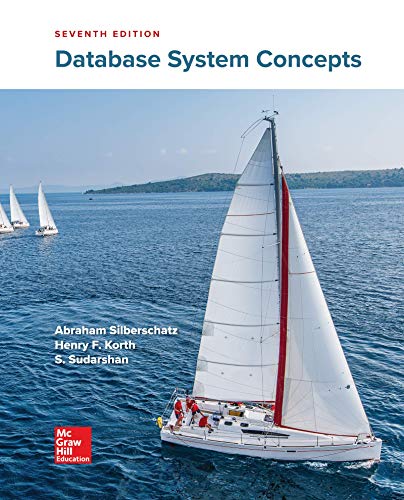
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
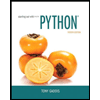
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
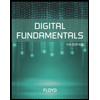
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
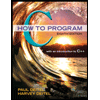
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
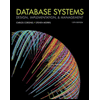
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
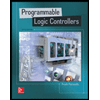
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education