PLEASE EXPLAIN/DISCUSS THE MATLAB CODES BELOW % Let's start the program % start the program % request the user to input the desired option with regard the unit % if the user input is ‘MKS’, then the units will be in meters and seconds % If the user input is ‘FPS’, then the units will be in feet and seconds unit = input("Please choose a unit: MKS (meters and seconds) or FPS (feet and seconds): ", "s"); % conditional statements enable you to select at run time which block of code to execute % in the situation where the user has input “MKS” if (unit == "MKS" || unit == "mks") % gravity is equivalent to -9.81 gravity = -9.81; % in the situation where the user has input “FPS” elseif (unit == "FPS" || unit == "fps") % gravity is equivalent to -32.174 gravity = -32.174; else % in the situation where the user has input none of the provided options error = " Please enter an input only within the provided options "; % disp( ) displays the value of variable without printing the variable name % display error disp(error) % return control to the invoking program before it reaches the end of the script or function return % to terminate statements end % for the variables needed in accordance with the formula % request the user to input the desired value for the magnitude of the initial velocity Vo = input("Enter magnitude of the initial velocity: "); % request the user to input the desired value for the angle theta = input("Enter angle: "); % request the user to input the desired value for the height h = input("Enter height: "); % request the user to input the desired value for the acceleration ax = input("Enter acceleration: "); % mathematical formula equations % formula equation for time time = (Vo.*sind(theta) + sqrt((Vo.*sind(theta)).^2-2*gravity*h)) / (-gravity); t = linspace(0,time); % formula equation for velocity (x) velocityx = Vo*(cosd(theta)); % formula equation for velocity (y) velocityy = Vo*(sind(theta)); % formula equation for displacement (x) displacementx = (velocityx.*t) + ((1/2)*ax*(t.^2)); % formula equation for displacement (y) displacementy = (velocityy.*t) + ((1/2)*gravity*(t.^2)); % formula equation for final velocity (x) finalvelocityx = sqrt(velocityx.^2 + 2*ax*displacementx); % formula equation for final velocity (y) finalvelocityy = sqrt(velocityy.^2 + 2*gravity*displacementy); % formula equation for magnitude magnitude = sqrt((finalvelocityx.^2)+(finalvelocityy.^2)); % For Plotting % for figure 1 figure(1) % plot function is used to create a graphical representation % linewidth specifies the width of the line % -k = solid black plot (t, magnitude,'-k','linewidth',3) % title adds the specified title to the current axes or standalone visualization title('Magnitude of Velocity Versus Time') % labels the x-axis of the current axes or standalone visualization xlabel('Time') % labels the y-axis of the current axes or standalone visualization ylabel('Magnitude of Velocity') % displays the major grid lines for the current axes grid on % for figure 2 figure(2) % plot function is used to create a graphical representation % linewidth specifies the width of the line % -k = solid black plot (displacementx,displacementy,'-k','LineWidth',3) % hold on is utilized to add a second line plot without deleting the existing line plot hold on % title adds the specified title to the current axes or standalone visualization title('Trajectory of the body') % labels the x-axis of the current axes or standalone visualization xlabel("Horizontal Displacement(m)") % labels the y-axis of the current axes or standalone visualization ylabel("Vertical Displacement(m)") % displays the major grid lines for the current axes grid on % for figure 3 figure(3); % hold on is utilized to add a second line plot without deleting the existing line plot hold on % length(t) returns the length of the largest array dimension in t for n = 1:length(t) % plot function is used to create a graphical representation plot (displacementx(1:n),displacementy(1:n), '--r','linewidth',3) % labels the x-axis of the current axes or standalone visualization xlabel("Horizontal Displacement(m)") % labels the y-axis of the current axes or standalone visualization ylabel("Vertical Displacement(m)") % title adds the specified title to the current axes or standalone visualization title("Trajectory") % temporarily stops MATLAB execution and waits for the user to press any key pause(0.1) % hold on is utilized to add a second line plot without deleting the existing line plot hold on % for termination end
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
PLEASE EXPLAIN/DISCUSS THE MATLAB CODES BELOW

Step by step
Solved in 8 steps

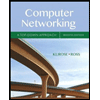
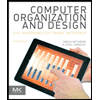
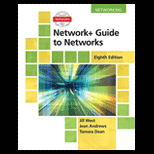
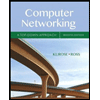
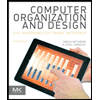
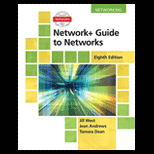
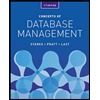
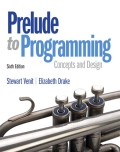
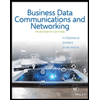