Please help with this error. I have 2 seperate files pet.java and dog.java. I don't know what code I'm missing or how to resolve the errors, I've been at this for 3 days trying to trouble shoot. I wrote the code and everything passed except I keep getting below errors: Class Definition: Please click next for more feedback. If you review the UML diagram you will find that the classes are to be in separate class files. Class found, not "Dog" (case-sensitive) // pet.java public class Pet { private String petType; private String petName; private int dogSpaces; private int catSpaces; private int petAge; private int daysStay; private double amountDue; // constructor public Pet (String petType, String petName, int dogSpaces, int catSpaces, int petAge, int dogsStay, double amountDue) { } /** * @return the petType */ public String getPetType() { return petType; } /** * @return the petName */ public String getPetName() { return petName; } /** * @return the petAge */ public int getPetAge() { return petAge; } /** * @return the dogSpaces */ public int getDogSpaces() { return dogSpaces; } /** * @return the catSpaces */ public int getCatSpaces() { return catSpaces; } /** * @return the daysStay */ public int getDaysStay() { return daysStay; } /** * @return the amountDue */ public double getAmountDue() { return amountDue; } /** * @param petType the petType to set */ public void setPetType(String petType) { this.petType = petType; } /** * @param petName the petName to set */ public void setPetName(String petName) { this.petName = petName; } /** * @param petAge the petAge to set */ public void setPetAge(int petAge) { this.petAge = petAge; } /** * @param dogSpaces the dogSpaces to set */ public void setdogSpaces(int dogSpaces) { this.dogSpaces = dogSpaces; } /** * @param catSpaces the catSpaces to set */ public void sectcatSpaces(int catSpaces) { this.catSpaces = catSpaces; } /** * @param daysStay the daysStay to set */ public void setdaysStay(int daysStay) { this.daysStay = daysStay; } /** * @param amountDue the amountDue to set */ public void setAmountDue(double amountDue) { this.amountDue = amountDue; } } /** * @author * dog.java */ public class Dog extends Pet{ public Dog(String petType, String petName, int dogSpaces, int catSpaces, int petAge, int dogsStay,double amountDue) { super(petType, petName, dogSpaces, catSpaces, petAge, dogsStay, amountDue); this.getPetAge(); // TODO Auto-generated constructor stub } public static void main(String[] args) { } private int dogSpaceNumber; private double dogWeight; private boolean grooming; /** * */ /** * @param args public static void main(String[] args) { } */ /** * @return the dogSpaceNumber */ public int getDogSpaceNumber() { return dogSpaceNumber; } /** * @param dogSpaceNumber the dogSpaceNumber to set */ public void setDogSpaceNumber(int dogSpaceNumber) { this.dogSpaceNumber = dogSpaceNumber; } /** * @return the dogWeight */ public double getDogWeight() { return dogWeight; } /** * @param dogWeight the dogWeight to set */ public void setDogWeight(double dogWeight) { this.dogWeight = dogWeight; } /** * @return the grooming */ public boolean isGrooming() { return grooming; } /** * @param grooming the grooming to set */ public void setGrooming(boolean grooming) { this.grooming = grooming; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Please help with this error. I have 2 seperate files pet.java and dog.java. I don't know what code I'm missing or how to resolve the errors, I've been at this for 3 days trying to trouble shoot.
I wrote the code and everything passed except I keep getting below errors:
Class Definition: Please click next for more feedback. If you review the UML diagram you will find that the classes are to be in separate class files.
Class found, not "Dog" (case-sensitive)


Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

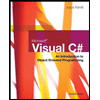
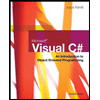