Problem: To write the JUnit test cases for our methods, you need to create two test methods: testLeapYear: This method should test if the isLeapYear method returns true for a given leap year. For example, you can choose the year 2020 and verify if the method returns true for it. If it does, the test will pass. If it doesn't, the test will fail. testNotLeapYear: This method should test if the isLeapYear method returns false for a given non-leap year. For example, you can choose the year 1900 and verify if the method returns false for it. If it does, the test will pass. If it doesn't, the test will fail. --------------------------------------------------------------------------------------------------------------------------------------------------------- import static org.junit.jupiter.api.Assertions.assertTrue; import org.junit.jupiter.api.Test; public class LeapYearTest { // Test case to check if a year is a leap year @Test public void testLeapYear() { //create a variable of int type called "year" and assign it with value "2020" //create a variable of boolean type, call it "expectedResult" and assign it with value "true" //Call the method isLeapYear() and pass "year" as an argument to it,store the result in a variable of boolean type called "actualResult" //Verify if the actual result is equal to the expected result using assertTrue() } // Test case to check if a year is not a leap year @Test public void testNotLeapYear() { //create a variable of int type,call it "year" and assign it with value "1900" //create a variable of boolean type, call it "expectedResult" and assign it with value "false" //Call the method isLeapYear() and pass "year" as an argument to it, store the result in a variable of boolean type called "actualResult" //Verify if the actual result is equal to the expected result using assertTrue() } //Do not modify this code // Method to check if a year is a leap year or not private static boolean isLeapYear(int year) { // Check if the year is divisible by 400 or divisible by 4 and not divisible by 100 return year % 400 == 0 || (year % 4 == 0 && year % 100 != 0); } }
-
Problem: To write the JUnit test cases for our methods, you need to create two test methods:
-
testLeapYear: This method should test if the isLeapYear method returns true for a given leap year. For example, you can choose the year 2020 and verify if the method returns true for it. If it does, the test will pass. If it doesn't, the test will fail.
-
testNotLeapYear: This method should test if the isLeapYear method returns false for a given non-leap year. For example, you can choose the year 1900 and verify if the method returns false for it. If it does, the test will pass. If it doesn't, the test will fail.
-
---------------------------------------------------------------------------------------------------------------------------------------------------------

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Code is not working for me. I used the same code but i keep getting error
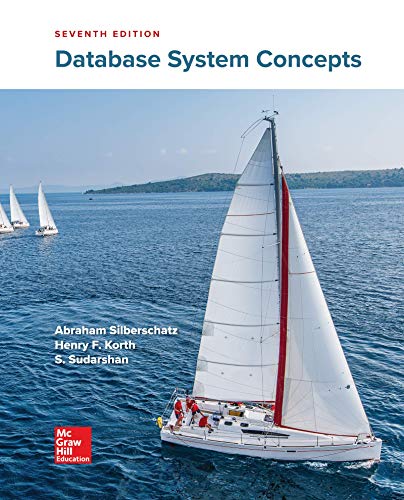
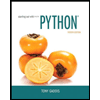
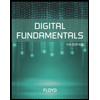
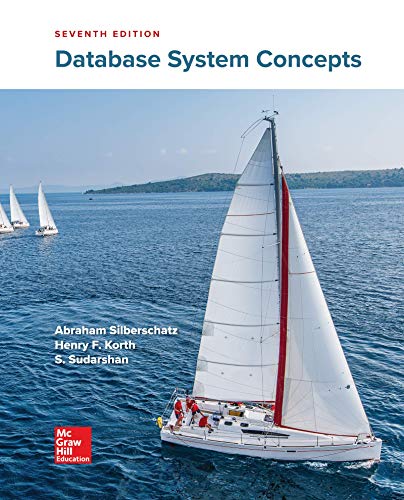
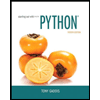
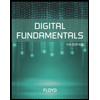
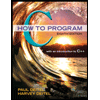
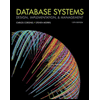
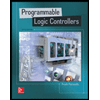