Provide a detailed explanation line by line. Explain how this C++ program functions Source Codes #include using namespace std; // Node struct to hold data and pointer to next node struct Node { int data; Node* next; }; // Linked List class class LinkedList { private: Node* head; // pointer to head node public: LinkedList() { head = NULL; // initialize head to NULL } // Insert a new node at the front of the list void insert(int value) { Node* newNode = new Node(); // create a new node newNode->data = value; // set the data of the new node to the input value newNode->next = head; // set the next pointer of the new node to the current head node head = newNode; // set the head pointer to the new node } // Delete a node with a specific value from the list void deleteNode(int value) { Node* current = head; // set a pointer to the current node Node* previous = NULL; // set a pointer to the previous node // Iterate through the list to find the node with the specific value while (current != NULL) { if (current->data == value) { // If the current node is the head, update the head pointer if (previous == NULL) { head = current->next; } // Otherwise, update the previous node's next pointer else { previous->next = current->next; } delete current; // delete the current node return; } // Update the previous and current pointers previous = current; current = current->next; } cout << "Element successfully removed.\n"; } // Print the full list void getFullList() { Node* current = head; // set a pointer to the current node cout << "Full List: "; // Iterate through the list and print each node's data while (current != NULL) { cout << current->data << " "; current = current->next; } cout << endl; } // Check if the list is empty bool isEmpty() { if (head == NULL) { return true; } return false; } //to modify an existing element void modify(int ele,int ele2) { Node* current = head; // set a pointer to the current node bool f=false; // Iterate through the list and print each node's data while (current != NULL) { if(current->data==ele){ current->data=ele2; f=true; } current = current->next; } if(f) cout<<"\nAll occurrences of "<>ch; switch(ch){ case 1 : cout<<"Enter an element to insert : "; cin>>ele;list.insert(ele); cout << "Element successfully added.\n";break; case 2 : cout<<"Enter an element to delete : "; cin>>ele;list.deleteNode(ele);break; case 3: cout<<"Enter an element to be Modified : "; cin>>ele; cout<<"Enter the new value : "; cin>>ele2; list.modify(ele,ele2);break; case 4: // Print the updated list list.getFullList(); break; case 5: // Check if the list is empty if (list.isEmpty()) { cout << "The list is empty." << endl; } else { cout << "The list is not empty." << endl; } // Output: The list is not empty. break; case 6: exit(0); default : cout<<"Invalid choice..."; } } return 0;
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Provide a detailed explanation line by line. Explain how this C++ program functions
Source Codes
#include <iostream>
using namespace std;
// Node struct to hold data and pointer to next node
struct Node {
int data;
Node* next;
};
// Linked List class
class LinkedList {
private:
Node* head; // pointer to head node
public:
LinkedList() {
head = NULL; // initialize head to NULL
}
// Insert a new node at the front of the list
void insert(int value) {
Node* newNode = new Node(); // create a new node
newNode->data = value; // set the data of the new node to the input value
newNode->next = head; // set the next pointer of the new node to the current head node
head = newNode; // set the head pointer to the new node
}
// Delete a node with a specific value from the list
void deleteNode(int value) {
Node* current = head; // set a pointer to the current node
Node* previous = NULL; // set a pointer to the previous node
// Iterate through the list to find the node with the specific value
while (current != NULL) {
if (current->data == value) {
// If the current node is the head, update the head pointer
if (previous == NULL) {
head = current->next;
}
// Otherwise, update the previous node's next pointer
else {
previous->next = current->next;
}
delete current; // delete the current node
return;
}
// Update the previous and current pointers
previous = current;
current = current->next;
}
cout << "Element successfully removed.\n";
}
// Print the full list
void getFullList() {
Node* current = head; // set a pointer to the current node
cout << "Full List: ";
// Iterate through the list and print each node's data
while (current != NULL) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
// Check if the list is empty
bool isEmpty() {
if (head == NULL) {
return true;
}
return false;
}
//to modify an existing element
void modify(int ele,int ele2) {
Node* current = head; // set a pointer to the current node
bool f=false;
// Iterate through the list and print each node's data
while (current != NULL) {
if(current->data==ele){
current->data=ele2;
f=true;
}
current = current->next;
}
if(f)
cout<<"\nAll occurrences of "<<ele<<" was replaced with "<<ele2<<endl;
else
cout<<"\nThe element not found\n";
cout << endl;
}
};
int main() {
LinkedList list;
int ch,ele,ele2;
while(true){
cout<<"\n1.Insert Data \n2.Delete Data \n3.Modify Data \n4.Get Full List \n5.Empty List \n6.Exit\n\nEnter Your Choice : ";
cin>>ch;
switch(ch){
case 1 : cout<<"Enter an element to insert : ";
cin>>ele;list.insert(ele);
cout << "Element successfully added.\n";break;
case 2 : cout<<"Enter an element to delete : ";
cin>>ele;list.deleteNode(ele);break;
case 3: cout<<"Enter an element to be Modified : ";
cin>>ele;
cout<<"Enter the new value : ";
cin>>ele2;
list.modify(ele,ele2);break;
case 4: // Print the updated list
list.getFullList(); break;
case 5: // Check if the list is empty
if (list.isEmpty()) {
cout << "The list is empty." << endl;
}
else {
cout << "The list is not empty." << endl;
}
// Output: The list is not empty.
break;
case 6: exit(0);
default : cout<<"Invalid choice...";
}
}
return 0;
}

Step by step
Solved in 2 steps

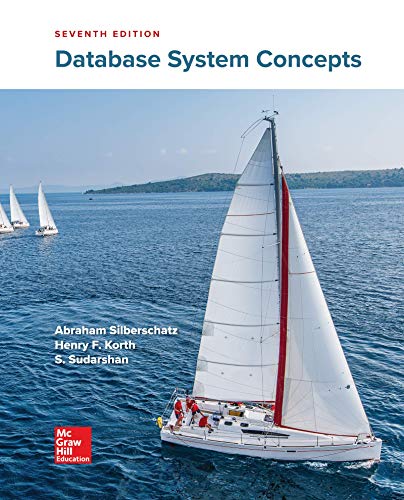
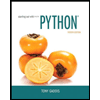
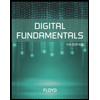
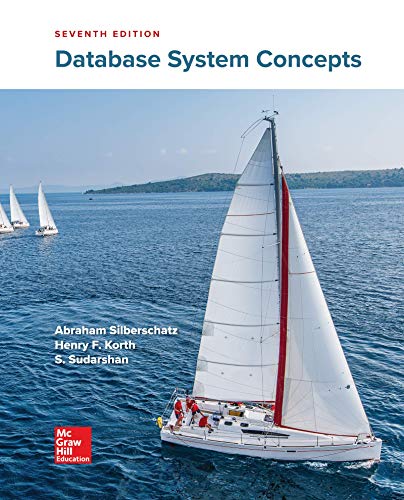
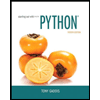
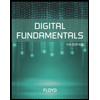
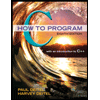
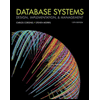
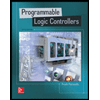