python exercise: Create a system to manage a library's collection of books. You want to create a class hierarchy to represent the different types of books in the library, including fiction books and non-fiction books as well as set up borrowing and returning books. Define a base class called Book with the following attributes and methods: Attributes: title: a string representing the title of the book. author: a string representing the author of the book. isbn: a string representing the ISBN number of the book. pages: an int representing the number of pages in the book copyright: an int listing the year the book was published available: a boolean that indicates whether or not the book is able to be borrow
python exercise:
Create a system to manage a library's collection of books. You want to create a class hierarchy to represent the different types of books in the library, including fiction books and non-fiction books as well as set up borrowing and returning books.
Define a base class called Book with the following attributes and methods:
Attributes:
- title: a string representing the title of the book.
- author: a string representing the author of the book.
- isbn: a string representing the ISBN number of the book.
- pages: an int representing the number of pages in the book
- copyright: an int listing the year the book was published
- available: a boolean that indicates whether or not the book is able to be borrowed
Methods:
- __init__(self, title, author, isbn): a constructor method that sets the initial values of the attributes.
- get_title(self): a method that returns the title of the book.
- get_author(self): a method that returns the author of the book.
- get_isbn(self): a method that returns the ISBN number of the book.
- get_pages(self): a method that returns the number of pages in the book.
- get_copyright(self): a method that returns the year they book was published.
- is_available(self): a method which returns True if the book is available.
- borrow(self): a method that sets the value of available to True if the book is not borrowed and raises a ValueError otherwise. The response for this should let the user know the date it is due back (2 weeks from today).
- return_book(self): a method that sets the value of available to True if the book is currently borrowed, and raises a ValueError otherwise. Include a message thanking the user for returning the book. Make sure to print out the name of the book in the message.
Create a subclass called FictionBook that inherits from the Book class. The FictionBook class should have an additional attribute called genre (a string representing the genre of the book, e.g. "mystery", "romance", etc.). The FictionBook class should also have a method called get_genre(self) that returns the genre of the book.
Create another subclass called NonFictionBook that also inherits from the Book class. The NonFictionBook class should have an additional attribute called subject (a string representing the subject of the book, e.g. "history", "science", etc.). The NonFictionBook class should also have a method called get_subject(self) that returns the subject of the book.
Based on the library system code you wrote, write a suite of test functions that test all functionality of the methods you created.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

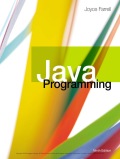
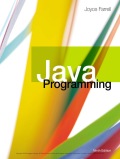