PYTHON PROGRAMMING ONLY PLEASE (Need Help with my User Inputs section only rest of code is fine) Create a program to sort a list of given numbers in ascending order. For example, if given 2,7,2,4,5,6 the output would be 2, 2, 4, 5, 6, 7 Do not use any libraries for the sort you must create the sort algorithm from scratch or use one of the sort codes we used in class. At the very least your program should: Ask the user if they would like to enter numbers into a list or create a random list. If they choose to enter numbers into a list ask them to enter numbers for the list. (Make sure to tell them how to stop entering numbers ie enter q to stop.) If they choose to create a random list ask them how many numbers they would like in their list. Print the unsorted list to the user. Then print the sorted list to the user. Make sure to use exception handling to save your program from crashing. from TimerFile import Timer from RandListFile import RandList from SortFile import Sort from SearchesFile import Search ''' Describe instances of the classes ''' #instances of classes timerInst = Timer() sortInst = Sort() searchInst = Search() randomInst = RandList() #Original Random List to use randomInst.createRandomList(10) randomListToSort = randomInst.getRandList() ''' User Inputs ''' # Python Program to Sort List in Ascending Order randomList = randomInst.getRandList() questionOne = int(input("Would you like to create a list or have a random list ?")) if questionOne == 1: print('You have choose Create A List') else: if questionOne == 2: print('You have choose Random List') print('Your random is list is:',randomList) NumList = [] Number = int(input("How many numbers would you like in the list : ")) for i in range(1, Number + 1): value = int(input("Please enter the number you would like in the list : ")) NumList.append(value) print("Unsorted Array") print(NumList) print('Sorted Array in Ascending Order:') print(NumList) ''' Search using some type of Sort ''' #quickSort print('--- Quick Sort----') quickRandomInst = randomListToSort.copy() quickSortList = randomInst.getRandList() randomInst.showFirtTenOfList() timerInst.startTimer() sortListQuick = sortInst.quick_sort(quickSortList,0,len(quickSortList)-1) randomInst.showFirtTenOfList() timerInst.stopTimer() timerInst.results() timerInst.resetTimer() print('--- End Quick Sort----')
PYTHON
Create a program to sort a list of given numbers in ascending order.
For example, if given 2,7,2,4,5,6 the output would be 2, 2, 4, 5, 6, 7
Do not use any libraries for the sort you must create the sort
At the very least your program should:
- Ask the user if they would like to enter numbers into a list or create a random list.
- If they choose to enter numbers into a list ask them to enter numbers for the list. (Make sure to tell them how to stop entering numbers ie enter q to stop.)
- If they choose to create a random list ask them how many numbers they would like in their list.
- Print the unsorted list to the user.
- Then print the sorted list to the user.
- Make sure to use exception handling to save your program from crashing.
from TimerFile import Timer
from RandListFile import RandList
from SortFile import Sort
from SearchesFile import Search
'''
Describe instances of the classes
'''
#instances of classes
timerInst = Timer()
sortInst = Sort()
searchInst = Search()
randomInst = RandList()
#Original Random List to use
randomInst.createRandomList(10)
randomListToSort = randomInst.getRandList()
'''
User Inputs
'''
# Python Program to Sort List in Ascending Order
randomList = randomInst.getRandList()
questionOne = int(input("Would you like to create a list or have a random list ?"))
if questionOne == 1:
print('You have choose Create A List')
else:
if questionOne == 2:
print('You have choose Random List')
print('Your random is list is:',randomList)
NumList = []
Number = int(input("How many numbers would you like in the list : "))
for i in range(1, Number + 1):
value = int(input("Please enter the number you would like in the list : "))
NumList.append(value)
print("Unsorted Array")
print(NumList)
print('Sorted Array in Ascending Order:')
print(NumList)
'''
Search using some type of Sort
'''
#quickSort
print('--- Quick Sort----')
quickRandomInst = randomListToSort.copy()
quickSortList = randomInst.getRandList()
randomInst.showFirtTenOfList()
timerInst.startTimer()
sortListQuick = sortInst.quick_sort(quickSortList,0,len(quickSortList)-1)
randomInst.showFirtTenOfList()
timerInst.stopTimer()
timerInst.results()
timerInst.resetTimer()
print('--- End Quick Sort----')

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

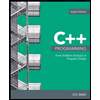
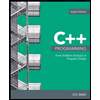