Question 1 Create a package named Lab6Q. Follow the parts outlined below: Part A: Add a public class called MyMethod in the Lab6Q package. Just like Math class, this will be your class that will have all your public-static methods, which you will call with the aid of the class name, as you do for Math method calls. Add and define the following public-static methods: • public static double myPow(double x, int y) – this method will accept a real number x as base and an integer number y as power, and return x'. Here instead of using the Math.pow() method you need to define this method (Hint: this method has been solved in the handout) • public static double mySin(double x) – this method will accept a real number (in radian value) and return the sin of that real number. Here instead of using Math.sin() method, you will define this method using the following numerical expression: N (-1)" sin x = x(2n+1) (2n + 1)! n=0 Where, n and N are integer values an0! = 1 for n = 0, and n! = 1 x 2 x 3 x 4 x . .x n, for n>0. Pick N = 20 [Hint: Similar problem has been solved in Unit 5, slide 78 (brief version). Also, this slide has been discussed in the pre-recorded lecture and during the class-lecture where it has been said that this kind of questions are asked in the exam.] Just create a driver method here, and check whether it works or not. sin(Math.PI/6) = 0.50. Do the same for the other methods in this class. • public static double myCos(double x) – this method will accept a real number (in radian value) and return the cos of that real number. Here instead of using Math.cos() method, you will define this method using the following numerical expression: (-1)" -x(2n) (2n)! n=0 cOs x = Pick N = 20. Once you try the above with Math.pow() method, replace that with your myPow() method and see whether you get the same result or not. public static void header and footer methods – copy these methods from the previous lab and paste those here.
Question 1 Create a package named Lab6Q. Follow the parts outlined below: Part A: Add a public class called MyMethod in the Lab6Q package. Just like Math class, this will be your class that will have all your public-static methods, which you will call with the aid of the class name, as you do for Math method calls. Add and define the following public-static methods: • public static double myPow(double x, int y) – this method will accept a real number x as base and an integer number y as power, and return x'. Here instead of using the Math.pow() method you need to define this method (Hint: this method has been solved in the handout) • public static double mySin(double x) – this method will accept a real number (in radian value) and return the sin of that real number. Here instead of using Math.sin() method, you will define this method using the following numerical expression: N (-1)" sin x = x(2n+1) (2n + 1)! n=0 Where, n and N are integer values an0! = 1 for n = 0, and n! = 1 x 2 x 3 x 4 x . .x n, for n>0. Pick N = 20 [Hint: Similar problem has been solved in Unit 5, slide 78 (brief version). Also, this slide has been discussed in the pre-recorded lecture and during the class-lecture where it has been said that this kind of questions are asked in the exam.] Just create a driver method here, and check whether it works or not. sin(Math.PI/6) = 0.50. Do the same for the other methods in this class. • public static double myCos(double x) – this method will accept a real number (in radian value) and return the cos of that real number. Here instead of using Math.cos() method, you will define this method using the following numerical expression: (-1)" -x(2n) (2n)! n=0 cOs x = Pick N = 20. Once you try the above with Math.pow() method, replace that with your myPow() method and see whether you get the same result or not. public static void header and footer methods – copy these methods from the previous lab and paste those here.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:modify this class by taking out most of the previous methods and adding couple of methods as outlined
below.
RecComplexNum
-real: double
-imaginary: double
+RecComplexNum ()
+RecComplexNum (re: double, im: double)
+getPolarFromRec():PolarComplexNum
+displayRecForm():void
Specifications of the RecComplexNum Class:
The constructor without argument will assign all field values to zero.
• The constructor with parameter should accept the real and imaginary values as arguments, and
assign these values to the fields real and imaginary.
The getPolarFromRec() helper method will return the polar form of a RecComplexNum Object, as
a PolarComplexNum object (you need to create this class in next-step). Inside this method the
magnitude and angle of a PolarComplexNum Object will be calculated as follows:
o Magnitude of a complex number a+bi = Va? + b² - here you are required to use your
MyMethod.myPow() method (you just created) and Math.sqrt() method.
o The angle of a complex number is a+bi = tan-1º. Here you will use Math's atan(x) and
toDegrees(x) methods.
o Once done, you will instantiate a PolarComplexNum object with these two values
(magnitude and angle) and return it.
The displayRecForm() method will print the RecComplexNum Object with its real and imaginary
parts (see the sample-output for an idea).
a
Part C:
Add a third public class called PolarComplexNum in the Lab6Q package, and define this class using the
following UML diagram, and associated specifications. This class will hold on to the characteristics of a
Complex class in Polar form.
PolarComplexNum
-magnitude: double
-angle: double
+PolarComplexNum ()
+PolarComplexNum (mag: double, ang: double)
+getRecFromPolar(): RecComplexNum
+displayPolarForm():void
Specifications of the PolarComplexNum Class:
The constructor without argument will assign all field values to zero.
The constructor with parameter should accept the magnitude and angle value (in degree) as
arguments, and assign these values to the fields magnitude and angle.
![Question 1
Create a package named Lab6Q. Follow the parts outlined below:
Part A:
Add a public class called MyMethod in the Lab6Q package. Just like Math class, this will be your class that
will have all your public-static methods, which you will call with the aid of the class name, as you do for
Math method calls. Add and define the following public-static methods:
• public static double myPow(double x, int y) – this method will accept a real number x as base and an
integer number y as power, and return x'. Here instead of using the Math.pow() method you need to
define this method (Hint: this method has been solved in the handout)
• public static double mySin(double x) – this method will accept a real number (in radian value) and
return the sin of that real number. Here instead of using Math.sin() method, you will define this
method using the following numerical expression:
N
(-1)"
:2 (2n + 1)!
x(2n+1)
sin x =
n=0
Where, n and N are integer values an0! = 1 for n = 0, and n! = 1 x 2 x 3 x 4 x.
Pick N = 20 [Hint: Similar problem has been solved in Unit 5, slide 78 (brief version). Also, this slide has
been discussed in the pre-recorded lecture and during the class-lecture where it has been said that this
kind of questions are asked in the exam.] Just create a driver method here, and check whether it works or
not. sin(Math.PI/6) = 0.50. Do the same for the other methods in this class.
• public static double myCos(double x) – this method will accept a real number (in radian value) and
return the cos of that real number. Here instead of using Math.cos() method, you will define this
method using the following numerical expression:
..x n, for n>0.
-x(2n)
(2n)!
n=0
cOs X =
Pick N = 20. Once you try the above with Math.pow(() method, replace that with your myPow()
method and see whether you get the same result or not.
• public static void header and footer methods – copy these methods from the previous lab and paste
those here.
Part B:
Add a second public class called RecComplexNum in the Lab6Q package, and define this class using the
following UML diagram, and associated specifications. This class will hold on to the characteristics of a
Complex class in Rectangular/Cartesian form. You can copy-paste your ComplexNumber class from the
previous lab just by copying from its package to your Lab6Q package in the IntelliJ IDE environment, then
after right clicking on it, select Refractor →Rename, and then rename it as RecComplexNum. You need to](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd9c8c467-5941-41b1-96d0-a8f6db4a71ec%2Fd703d712-b00e-40ca-ba62-9f6e25b008fc%2F1nkgkb_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Question 1
Create a package named Lab6Q. Follow the parts outlined below:
Part A:
Add a public class called MyMethod in the Lab6Q package. Just like Math class, this will be your class that
will have all your public-static methods, which you will call with the aid of the class name, as you do for
Math method calls. Add and define the following public-static methods:
• public static double myPow(double x, int y) – this method will accept a real number x as base and an
integer number y as power, and return x'. Here instead of using the Math.pow() method you need to
define this method (Hint: this method has been solved in the handout)
• public static double mySin(double x) – this method will accept a real number (in radian value) and
return the sin of that real number. Here instead of using Math.sin() method, you will define this
method using the following numerical expression:
N
(-1)"
:2 (2n + 1)!
x(2n+1)
sin x =
n=0
Where, n and N are integer values an0! = 1 for n = 0, and n! = 1 x 2 x 3 x 4 x.
Pick N = 20 [Hint: Similar problem has been solved in Unit 5, slide 78 (brief version). Also, this slide has
been discussed in the pre-recorded lecture and during the class-lecture where it has been said that this
kind of questions are asked in the exam.] Just create a driver method here, and check whether it works or
not. sin(Math.PI/6) = 0.50. Do the same for the other methods in this class.
• public static double myCos(double x) – this method will accept a real number (in radian value) and
return the cos of that real number. Here instead of using Math.cos() method, you will define this
method using the following numerical expression:
..x n, for n>0.
-x(2n)
(2n)!
n=0
cOs X =
Pick N = 20. Once you try the above with Math.pow(() method, replace that with your myPow()
method and see whether you get the same result or not.
• public static void header and footer methods – copy these methods from the previous lab and paste
those here.
Part B:
Add a second public class called RecComplexNum in the Lab6Q package, and define this class using the
following UML diagram, and associated specifications. This class will hold on to the characteristics of a
Complex class in Rectangular/Cartesian form. You can copy-paste your ComplexNumber class from the
previous lab just by copying from its package to your Lab6Q package in the IntelliJ IDE environment, then
after right clicking on it, select Refractor →Rename, and then rename it as RecComplexNum. You need to
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

Recommended textbooks for you
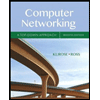
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
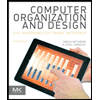
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
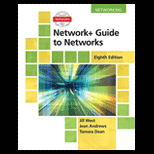
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
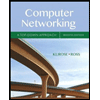
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
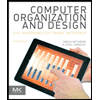
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
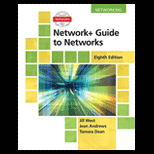
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
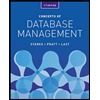
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
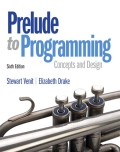
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
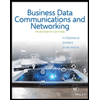
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY