Question 1) Push five integer values into a stack. 2) Pop all values from the stack then store the popped values in a linked list and print it on the screen. However, some parts of the code are missing (indicated by Complete the missing parts. #define STACKSIZE 5 struct stack { The following code implements the following: int items [STACKSIZE]; _top; }; struct node { int data; _next; }; typedef struct node* void push(struct stack, int); int pop(struct stack *); void insert(node *head, int); int main(void) { s.top= for (int i = 0; i < 5; i++) { int num; printf("Enter a Number \n"); scanf (%d, &num); push(- } int x = pop(----------➖➖➖➖➖➖➖); printf("First value is deleted \n"); node *head = (NODEPTR) malloc(sizeof(struct node));; head->data = x; head->next = -------- for (int i = 1; i < 5; i++) { x = pop (- ); insert (head, x); printf("%d is deleted \n", x); } return 0; }//end of main );
Question 1) Push five integer values into a stack. 2) Pop all values from the stack then store the popped values in a linked list and print it on the screen. However, some parts of the code are missing (indicated by Complete the missing parts. #define STACKSIZE 5 struct stack { The following code implements the following: int items [STACKSIZE]; _top; }; struct node { int data; _next; }; typedef struct node* void push(struct stack, int); int pop(struct stack *); void insert(node *head, int); int main(void) { s.top= for (int i = 0; i < 5; i++) { int num; printf("Enter a Number \n"); scanf (%d, &num); push(- } int x = pop(----------➖➖➖➖➖➖➖); printf("First value is deleted \n"); node *head = (NODEPTR) malloc(sizeof(struct node));; head->data = x; head->next = -------- for (int i = 1; i < 5; i++) { x = pop (- ); insert (head, x); printf("%d is deleted \n", x); } return 0; }//end of main );
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 3PE
Related questions
Question

Transcribed Image Text:int pop(struct stack *ps) {
----- X;
if (ps->top== -1) {
printf("--
exit(1);
}
else {
}
X =
ps->top
}
return x;
}
void push(struct stack *ps, int x)
{
if (ps->top == STACKSIZE - 1) {
}
printf(".
exit(1);
}
else {
ps->top = ps->--
while (
}
void insert(node* head, int x) {
node *p = (NODEPTR) malloc(sizeof(struct node));
p->data = x;
p->next = NULL;
node *cur head;
---) {
if (cur->next == NULL)
{
}
x;
break;
-");
-");
![Question
1) Push five integer values into a stack.
2) Pop all values from the stack then store the popped values in a linked list and print it
on the screen. However, some parts of the code are missing (indicated by
The following code implements the following:
Complete the missing parts.
#define STACKSIZE 5
struct stack
{
int items [STACKSIZE];
_top;
};
struct node {
int data;
_next;
};
typedef struct node*
void push(struct stack, int);
int pop(struct stack *);
void insert(node *head, int);
int main(void) {
s.top=
for (int i = 0; i < 5; i++)
{
int num;
printf("Enter a Number \n");
scanf (%d, &num);
push(-
-s;
}
int x = pop(-
-----);
printf("First value is deleted \n");
head->next =
node *head = (NODEPTR) malloc(sizeof(struct node));;
head->data = x;
➖➖➖➖➖➖
}
return 0;
}//end of main
for (int i = 1; i < 5; i++)
{
x = pop (------
);
insert (head, x);
printf("%d is deleted \n", x);
);
Page 2 of 5](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F095291e7-ccfd-4808-a2e2-ecd34a09d8c2%2F4e90beb0-eb38-4eed-be90-8ccc7532bd48%2Fdts1ds4_processed.png&w=3840&q=75)
Transcribed Image Text:Question
1) Push five integer values into a stack.
2) Pop all values from the stack then store the popped values in a linked list and print it
on the screen. However, some parts of the code are missing (indicated by
The following code implements the following:
Complete the missing parts.
#define STACKSIZE 5
struct stack
{
int items [STACKSIZE];
_top;
};
struct node {
int data;
_next;
};
typedef struct node*
void push(struct stack, int);
int pop(struct stack *);
void insert(node *head, int);
int main(void) {
s.top=
for (int i = 0; i < 5; i++)
{
int num;
printf("Enter a Number \n");
scanf (%d, &num);
push(-
-s;
}
int x = pop(-
-----);
printf("First value is deleted \n");
head->next =
node *head = (NODEPTR) malloc(sizeof(struct node));;
head->data = x;
➖➖➖➖➖➖
}
return 0;
}//end of main
for (int i = 1; i < 5; i++)
{
x = pop (------
);
insert (head, x);
printf("%d is deleted \n", x);
);
Page 2 of 5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
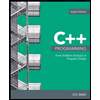
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
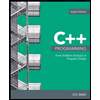
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning