READY CAREFULLY Java add COMMENT TO CODE, please TEST-CASE on how you would test your solution assumptions and hence your code. Example of cases to be tested for are like : What if the array input which is expected does not exist - that is , input is a null. How should your code handle such a situation ? Maybe output some message like "Null input case, so no output"? What if the length of the array is one ?....so on and so forth. In this assignment you are required programs which takes as input two sorted arrays and returns a new array containing the elements found in both the sorted arrays. It is alright if the input arrays have duplicates, but the returned array should be duplicate free! Below is how the arrays are represented ARRAY1[] = [1, 5, 6, 6, 9, 9, 9, 11, 11, 21] Here length of ARRAY1 is m. ARRAY2[] = [6, 6, 9, 11, 21, 21, 21] Here length of ARRAY2 is n. Array to be returned would be: ARRAY[] = [6, 9, 11, 21] ANSWER THE QUESTION BELOW. Implement the function in such a way that your solution solves the problem with O(nlog(m)) time complexity. This brute-force method suggested has a name called "loop-join" where you basically just traverse through the elements of one array comparing it to the elements of the other array. 2. In the form of sentences, as a comment in your code (at the bottom of your Solution2), you are required to suggest how can Solution2 be improved by leveraging the fact that both the arrays are already sorted. Suggest a solution so that your suggested solution can run linearly with O(m + n) time complexity. 5 sentences or less.
READY CAREFULLY
Java add COMMENT TO CODE, please TEST-CASE on how you would
test your solution assumptions and hence your code.
Example of cases to be tested for are like : What if the array input which
is expected does not exist - that is , input is a null. How should your code
handle such a situation ? Maybe output some message like "Null input
case, so no output"? What if the length of the array is one ?....so on and
so forth.
In this assignment you are required programs which takes as input two sorted arrays and returns a new array containing the elements found in both the sorted arrays. It is alright if the input arrays have duplicates, but the returned array should be duplicate free!
Below is how the arrays are represented
ARRAY1[] = [1, 5, 6, 6, 9, 9, 9, 11, 11, 21]
Here length of ARRAY1 is m.
ARRAY2[] = [6, 6, 9, 11, 21, 21, 21]
Here length of ARRAY2 is n.
Array to be returned would be:
ARRAY[] = [6, 9, 11, 21]
ANSWER THE QUESTION BELOW.
- Implement the function in such a way that your solution solves the problem with O(nlog(m)) time complexity. This brute-force method suggested has a name called "loop-join" where you basically just traverse through the elements of one array comparing it to the elements of the other array.
2. In the form of sentences, as a comment in your code (at the bottom of your Solution2), you are required to suggest how can Solution2 be improved by leveraging the fact that both the arrays are already sorted. Suggest a solution so that your suggested solution can run linearly with O(m + n) time complexity. 5 sentences or less.

Find the duplicates in two sorted arrays :
program in java:
public class arrayduplicates {
public static void main(String[] args) {
int[] arr1 = {1,5,6,6,9,9,9,11,11,21};
int[] arr2 = {6, 6, 9, 11, 21, 21, 21};
Integer[] duplicates = duplicates(arr1, arr2);
for(Integer d: duplicates) {
System.out.println(d.intValue());
}
}
public static Integer[] duplicates(int[] arr1, int[] arr2) {
List<Integer> duplicates = new ArrayList<Integer>();
for(int count = 0; count < sorted1.length; count ++) {
for(int counter = 0; counter < arr2.length; counter ++) {
if(sorted1[count] == arr22[counter]) {
duplicates.add(arr1[count]);
} else if(arr1[count] < arr2[counter]) {
break;
}
}
}
return duplicates.toArray(new Integer[duplicates.size()]);
}
}
the above program time complexity is O(n log m)
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

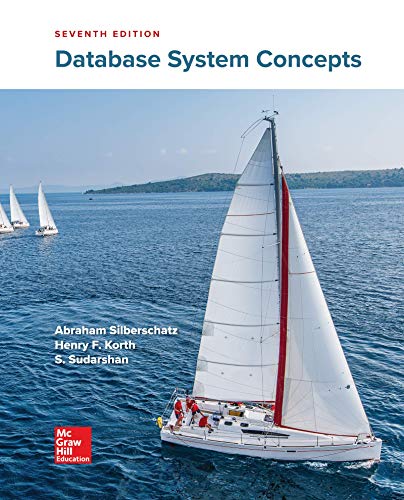
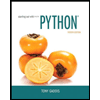
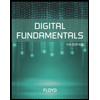
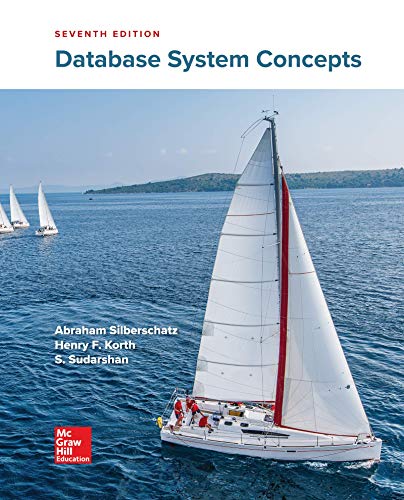
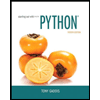
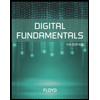
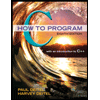
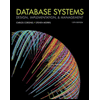
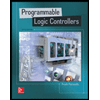